Arithmetic Operators
Arithmetic operators are used in mathematical expression the similar way in which they are used in algebra. The subsequent table lists the arithmetic operators:
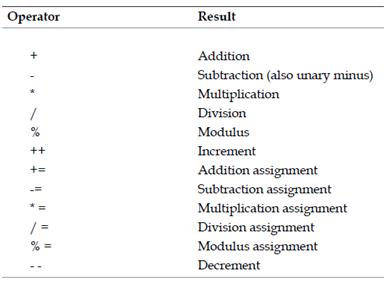
The operands of arithmetic operators must be of a numeric type. You cannot utilize them on boolean types but you could use them on char types, because the char type in
Java is an essentially, a subset of int.
// Show the basic arithmetic operators.
class basicMath {
public static void main (String args [ ] ) {
/ / arithmetic using integers
System.out.print1n ("Integer Arithmetic")
int a = 1 + 1;
int b = a * 3;
int c = b / 4;
int d = c - a;
int e = -d ;
System.out.println ( "a= " +a);
System.out.println ("b =" + b);
System.out.println ("c=" + c);
System.out.println ("d =" + d);
System.out.println (" e =" + e);
// arithmetic using doubles
System. out. println ("\no Floating Point Arithmetic");
double da = 1 + 1;
double db = da * 3;
double dc = db / 4;
double dd = dc - a;
double de = - dd;
System.out.println ("da=" +da);
System.out.println ("db=" +db);
System.out.println ("dc=" +dc);
System.out.println ("dd=" + dd);
System.out.println ("de =" +de);
Whenever you run this program, you will see the given output. Integer Arithmetic
a= 2
b= 6
c=1
d = -1
e = 1
Floating point Arithmetic
da = 2
db = 6
dc = 1.5
dd = -0.5
de = 0.5