Write a subroutine in C for toggling the cursor using old directives.
;
; use small memory model for C - near code segment
_DATA SEGMENT WORD 'DATA'
CURVAL EQU [BP+4] ; parameters
_DATA ENDS
_TEXT SEGMENT BYTE PUBLIC 'CODE'
DGROUP GROUP _DATA
ASSUME CS:_TEXT, DS:DGROUP, SS:DGROUP
PUBLIC _CURSW
_CURSW PROC NEAR
PUSH BP ; BP register of caller is saved
MOV BP, SP ; BP is pointing to stack now
MOV AX, CURVAL
CMP AX, 0H
JZ CUROFF; Execute code for cursor off
CMP AX, 01H
JZ CURON; Execute code for cursor on
JMP OVER ; Error in parameter, do nothing
CUROFF: ; write code for curoff
:
:
JMP OVER
CURON: ; write code for curon
:
:
OVER: POP BP
RET
_CURSW ENDP
_TEXT ENDS
END
Why the parameter is found in [BP+4]? Please look into the following stack for answer.
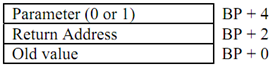