Write a program to echo the string 'Hello' to the serial channel (SCI) using the protocol of baud rate 9600,8 bits , no parity and 1 stop bit
Consider the baud register as shown below
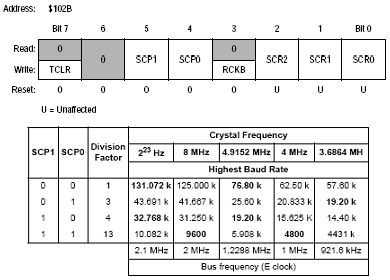
Using a combination of bits5,4,2,1,0 we can set up the required baud rate .On the target system provided the input clock crystal is a 8Mhz (2Mhz internal E) version , therefore for a baud rate of 9600 is the easiest one
Bit 5= 1 Bit 4 = 1 Bit 2 = 0 Bit 1 = 0 Bit 0 = 0
i.e. (2Mhz / 13) = 9600 *8 bits per second i.e. 9600 Baud
Baud = 0x30
The bit length mode is set by bit 4 of the SCCR1 as shown below
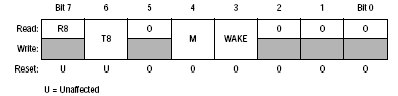
Bit = 0 'Start bit 8 data bits 1 stop bit'
Bit = 1 'Start bit 9 data bits 1 stop bit '
Therefore bit 4 of sccr1 = 0 Sccr1 = 0x00
The SCI control 2 enables us to set interrupts if data is received or can be transmitted; in this case we simply want to enable the transmitter and receiver
Therefore
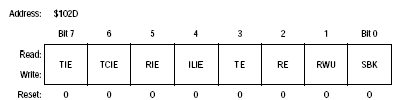
TE Bit 3, 2= 1
Sccr2 = 0x0c
This completes the SCI set-up, the remaining register is useful in determining whether data is received or can be transmitted
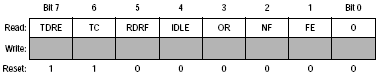
We can simply check bit7 if this is '1' we can send more data ,Since the configuration is actually set-up on the development boards we do not need to re-program the baud rates but simply use the comms channel. Therefore the a simple hello display program follows
name ex6 ;Use of the uart to echo Hello ten times
baud equ $2b ;Baud rate register
sccr1 equ $2c ;SCI control 1
sccr2 equ $2d ;SCI control 2
scsr equ $2e ;SCI status
scdr equ $2f ;Serial data register
org $500 ;Start on external ram
;Note that the uart is configured by the monitor
;the following codes are used for eprom use
; sccr1 = 0 hex (No 9th bit)
;baud = 30 Hex (9600 using 8Mhz xtal )
; sccr2 = 0c hex (Enable tx)
ldx #$0a ;load up Counter with 10
loop ldaa #'H' ;load up ASCII H
jsr txd ;Send data
ldaa #'e' ;Load up ASCII e
jsr txd ;Send data
ldaa #'l' ;Load up ASCII l
jsr txd ;Send data
ldaa #'l' ;Load up ASCII l
jsr txd ;Send data
ldaa #'o' ;Load up ASCII o
jsr txd ;Send data
ldaa #$0a ;Load up ASCII newline
jsr txd ;Send data
ldaa #$0d ;Load up ASCII return
jsr txd ;Send data
dex ;X=X-1
bne loop ;repeat till zero
swi ;return back to monitor
txd ldab scsr ;Get sci status
andb #$80 ;Mask off bit 7 TDRE
beq txd ;Wait till ready to transmit
staa scdr ;send data from A out
ldy #$ff ;Software time delay
loop1 dey ;instead of XON/XOFF protocol
bne loop1 ;wait till y = 0
rts
end