Stage One Define the specification of the program
Add two nxm size matrices
Stage Two Divide the program up into separate modules
Input Matrix
Add Matrix
Display Matrix
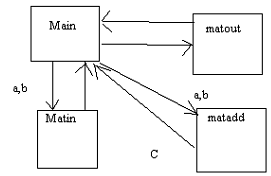
Stage Four Develop the Algorithm
Title: Matin
Input: Matrix array, size, name
Output: Matrix array
Function: Load up array from standard input/output
Title: Matadd
Input: Matrix array1, Matrix Array2, size ,
Output: Matrix array3
Function: Matrix Array3 = Matrix Array2 + Matrix Array1
Title: Matout
Input: Matrix array, size,
Output: none
Function: Display an array using standard input/output
Stage Five Code each module and write a main program to combine all the code.
Title: Matin
Input: Matrix array, size ,
Output: Matrix array
Function: Load up array from standard input/output
Before we code these modules we need to look at passing arrays in and out of functions. Here we must introduce the concept of the address of a variable. Within micros each variable is stored in memory at an address i.e. house number. If we pass across the actual address to a function and use that address to store data , then upon the return of the function , we have automatically return the required data , without using the return statement . This is termed 'return by address' and is how can works i.e.
scanf("%f",&value);
Here the function returns back the figure into the variable value, the '&' means pass across the address not the actual value. Simply to use arrays in functions we must pass the address across else we must use the return command.
void matin (int n, int m ,char name,float a[11][11])
{
/* Author: Mr James Mc Carren */
/* Company: Staffordshire University */
/*Date: 26th August 2012 */
/*Version 1.0 */
/*Function: Procedure to input a nxm matrix using standard input
Parameters used: n,m size of matrix Name Character to identify Which matrix is loaded Temp of type d which is an two dimensional array
Modifications: none */
int i,j;
/*Load up matrix A*/
for (j=1;j<=n;j++)
{
for (i=1;i<=m;i++)
{
printf(" Please enter element %3d %3d ", j ,i);
printf("of matrix %c\n\r",name);
scanf("%f",&a[j][ i]);
}
}
return;
}
Title: Matadd
Input: Matrix array1, Matrix Array2 , size ,
Output: Matrix array3
Function: Matrix Array3 = Matrix Array2 + Matrix Array1
void matadd (int n,int m,float a[11][11],float b[11][11],float c[11][11])
{
/*Author: Mr James Mc Carren
Company: Staffordshire University
Date: 26th August 2012
Version 1.0
Function: Procedure to add two nxm matrixes using standard input
Parameters used: n,m integer values matrix size Array A and B of type d Array C used as output Name Character to identify Which matrix is loaded Type d which is an two dimensional array
Modifications: none*/
int i,j;
/*Add matrix A to B to make C i.e [C] = [A] + [B]*/
for (j=1;j<=n;j++)
{
for (i=1;i<=m;i++)
{
c[j][ i] = a[j][ i] + b[j][ i];
}
}
return;
}
Title: Matout
Input: Matrix array, size ,
Output: none
Function: Display an array using standard input/output
void matout (int n, int m, char name, float c[11][11] )
{
/*Author: Mr James Mc Carren
Company: Staffordshire University
Date: 26th August 2012
Version 1.0
Function: Procedure to input an nxm matrix using standard input
Parameters used: n,m size of matrix Temp of type d which is an two dimensional array
Modifications: none*/
int i,j;
/*Display matrix C*/
for (j=1;j<=n;j++)
{
for (i=1;i<=m;i++)
{
printf("Element %3d %3d ", j , i );
printf("of matrix %c is %10.2f \n\r",name, c[j][ i]);
}
}
}