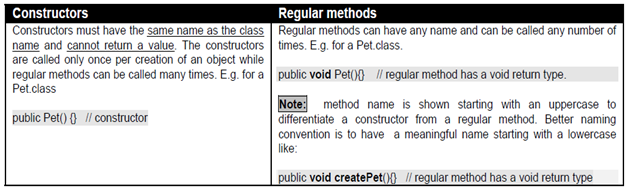
What occurs if you do not give a constructor? Java does not actually need an explicit constructor in the class description. If you do not add a constructor, the Java compiler can create a default constructor in the byte code with an empty argument. This default constructor is same to the explicit “Pet(){}”. If a class add one or more explicit constructors like “Pet(){}” or “public Pet(int id)” etc, the java compiler does not prepare the default constructor “Pet(){}”.
How to call the superclass constructor in sub class? If a class called “SpecialSet” extends your “Set” class then you will use the keyword “super ” to invoke the superclass’s constructor. E.g.
public SpecialSet(int id)
{
super(id); //must be the very first statement in the other constructor.
}
To call a regular method in the super class need: “super .myMethod( );”. This may be called at any line. Some frameworks based on JUnit include their own initialization code, and not only do they need to remember to invoke their parent's setup() function, you, as a user, have to remember to invoke theirs after you wrote your initialization code:
public class UnitTestCase extends TestCase {
public void setUp() {
super .setUp();
// do class own initialization
}
}
public void cleanUp() throws Throwable
{
try {
… // Do stuff here to clean up your object(s).
}
catch (Throwable t) {}
finally{
super .cleanUp(); //clean up your parent class.
// super.regularMethod() can be called at any line.
}
}