Type casting defines treating a variable of one type as though it is another type.
When up casting primitives as given below from left to right, automatic conversion happens. But if you derive from right to left, explicit casting or down casting is needed. Casting in Java is safer and easier than in C or other languages that allow arbitrary casting. Java only lets casts happen when they build sense, such as a cast between a float and an int. However you can't cast between a String and an int.
Byte, short, int, long, float, and double
int i = 5;
long j = i; //Right. Implicit casting or up casting
byte b1 = i; //Wrong casting. Compile time error "Type Mismatch".
byte b2 = (byte) i ; //Right. Explicit casting or Down casting is required.
When it needs to object references you may always cast from a subclass to a superclass because a subclass object is also a superclass instance. You may cast an object implicitly to a super class type. If that were not the case polymorphism couldn't be possible.
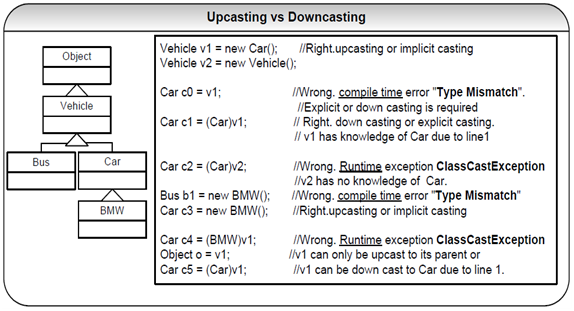
You can cast down the hierarchy as well but you have to explicitly write the cast and the object must be a legitimate instance of the class you are casting to. The ClassCastException is given to indicate that code has tried to cast an object to a subclass of which it is not an instance of that class. If you are using J2SE 5.0 then "generics" will remove the need for casting otherwise you may deal with the problem of incorrect casting in two ways:
1. Use the exception handling process to catch ClassCastException.
2. Use the instance of statement to protect against incorrect casting.