Use DDA algorithm to get the output of your program as shown in Figure
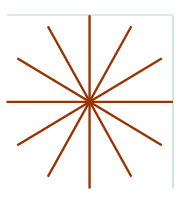
ANs: Use DDA( ) function to plot line segments that have end points on diametrically opposite points on the circumference of a circle. Take these points on uniform distance. For example, you may use the following function CirclePoints() to get line segment endpoints (x1 [i], y1[i]) and (x2[i], y2[i]), i=0,1,....5 and use the array 2 y ,2 x ,1y ,1x as input to your dda() function. Call the DDA function six times as given in plot Lines code below.
// Compute points on the circumference of a circle.
int Points(int r, int xc, int yc, int x1[6], int y1[6],int
x2[6], int y2[6])
{
float pi = 3.1416;
int i=0;
for(float theta = 0; theta
{
x1[i] = xc + r*cos(theta);
y1[i] =yc+r*sin(theta);
x2[i] = xc + r*cos(pi+theta);
y2[i] =yc+r*sin(pi+theta);
i++;
}
return x1[6], y1[6], x2[6],y2[6];
}
//Plot line segments using DDA algorithm
void plotLines()
{
int xx1[6],yy1[6],xx2[6],yy2[6];
Points(100, 200, 200, xx1, yy1, xx2,yy2);
for(int i=0;i<=5;i++)
dda(xx1[i], yy1[i], xx2[i], yy2[i]);
}
Add the above code in your program for line segment generation using dda() function and call the function plotLines in your RenderScene() function.