Creating Table
create table cars (Vin_num varchar(30),primary key(Vin_Num),Make varchar(15),Model varchar(20),Year int,Price varchar(20));
Inserting Records:
insert into cars values ('1A2A3A4A','Toyota','Camry',2005,'$14,500');
insert into cars values ('1B2B3B4B','Nissan','Altima',2007,'$16,550');
insert into cars values ('1C2C3C4C','Honda','Civic',1999,'$9,999');
insert into cars values ('1D2D3D4D','Chevrolet','Camero',2009,'$27,500');
insert into cars values ('1E2E3E4E','Chrysler','Crossfire',2010,'$35,499');
insert into cars values ('1F2F3F4F','Ford','Focus',1998,'$6,500');
insert into cars values ('1G2G3G4G','Dodge','Ram', 2008,'$6,555');
Table: customers
Creating Table
create table customers(Cust_ID varchar(8),primary key(Cust_ID),Cust_Name varchar(20), Address varchar(60),Phone varchar(15));
Inserting Records:
insert into customers values ('CUS001','John','625,Quincy Ave,Scranton,PA','435-345-3455');
insert into customers values ('CUS002','Peter','302, Phelps St,Scranton,PA','345-234-5234');
insert into customers values ('CUS003','Kevin','90, Richmond St,Dunmore,PA','345-345-2642') ;
insert into customers values ('CUS004','Sujal','114,S Irving Ave, Avoca,PA','655-854-8899');
insert into customers values ('CUS005','Rich','225, N Main St,Dicksoncity,PA','556-889-8896');
insert into customers values ('CUS006','Frank','850, Adams Ave, Kingston,PA','545-879-9988');
Table: employees
Creating Table
create table employees(Emp_ID varchar(8),primary key(Emp_ID),Emp_Name varchar(20),Position varchar(45),Salary varchar(10));
Inserting Records:
insert into employees values ('EMP001','Chris','Manager','$52,500');
insert into employees values ('EMP002','Kelly','Area Manager','$48,000');
insert into employees values ('EMP003','Andrew','Sales Manager ','$46,500');
insert into employees values ('EMP004','Tom','Executive','$45,000');
insert into employees values ('EMP005','Rick','Salesman','$35,500');
insert into employees values ('EMP006','Harris','Accountant','$30,500');
Table: sales
Creating Table
create table sales (Sale_ID varchar(8),primary key(sale_ID),Cust_ID varchar(8),Vin_Num varchar(10),Emp_ID varchar(8),foreign key (Cust_ID) references customers(Cust_ID),
foreign key (Vin_Num) references cars(Vin_Num), foreign key (Emp_ID) references employees(Emp_ID));
Inserting Records:
insert into sales values ('SLS001','CUS002','1C2C3C4C','EMP001');
insert into sales values ('SLS002','CUS002','1D2D3D4D','EMP002');
insert into sales values ('SLS003','CUS003','1E2E3E4E','EMP004');
insert into sales values ('SLS004','CUS004','1C2C3C4C','EMP003');
insert into sales values ('SLS005','CUS005','1F2F3F4F','EMP005');
insert into sales values ('SLS006','CUS001','1B2B3B4B','EMP006');
ERD:
Also I put Primary key(pk) and Foreign key(fk) into chart.
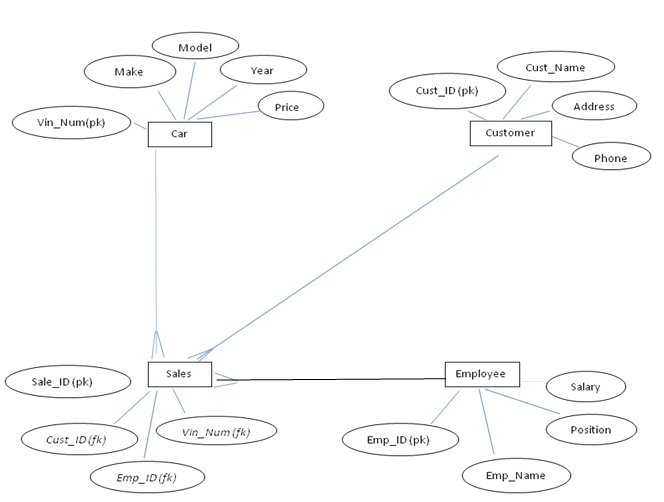
Need to be use it.
I need just PHP coding for 4 tables (car, customer, employees, and sales).
Also you can see first 2 pages are needed to be used information for PHP coding.
Also I put Example for inserting a record, updating a record, and deleting a record. You can see on the following pages.
But when you do PHP coding make sure uppercase is uppercase (Capital letter) and lowercase is lowercase (small letter).
Also you can see in ERD chart what's going on.
Each table need to be 3 files. (Car has 3 files, employees has 3 files, customer has 3 files, sales has 3 files)
For example, Always use to Add Vin_Num,etc.......
So, total 12 files.
Don't worry about this part I will fix it myself. Leave it same.
$conn = mysql_connect("database host", "username", "password");
$result = mysql_select_db("database name", $conn);