Convert ASCII code to its BCD equivalent. This can be achieved by simply replacing bits in upper four bits of byte by four zeros. For illustration the ASCII '1' is 32h = 0010B. By making upper four bits as 0 we obtain 0000 0010 that is 2 in BCD. Number attained is known as unpacked BCD number. Upper 4 bits of this byte is zero. So upper four bits can be used to store another BCD digit. The byte so obtained is known as packed BCD number. For illustration an unpacked BCD number 59 is 00000101 00001001 which is 05 09. Packed BCD will be 0101 1001 which is 59.
The algorithm to convert two ASCII digits to packed BCD can be defined as:
Convert first ASCII digit to unpacked BCD.
Convert second ASCII digit to unpacked BCD.

Move first BCD to upper four positions in byte.

Pack two BCD bits in one byte.
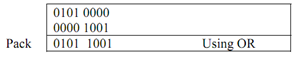
The assembly language program for the above can be written in the below manner.
; ABSTRACT Program produces a packed BCD byte from 2 ASCII
; encoded digits. Assume the number as 59.
; The first ASCII digit (5) is loaded in BL.
; The second ASCII digit (9) is loaded in AL.
; The result (packed BCD) is left in AL.
; REGISTERS ; Uses CS, AL, BL, CL
; PORTS ; None used
CODE SEGMENT
ASSUME CS:CODE
START: MOV BL, '5'; Load first ASCII digit in BL
MOV AL, '9'; Load second ASCII digit in AL
AND BL, 0Fh; Mask upper 4 bits of first digit
AND AL, 0Fh; Mask upper 4 bits of second digit
MOV CL, 04h; Load CL for 4 rotates
ROL BL, CL; Rotate BL 4 bit positions
OR AL, BL; Combine nibbles, result in AL contains 59
; As packed BCD
CODE ENDS
END START
Discussion:
8086 doesn't have any instruction to swap lower and upper 4 bits in a byte so we need to use rotate instructions that too by 4 times. Out of two rotate instructions RCL and ROL we have chosen ROL as it rotates the byte left by one or more positions whereas RCL moves MSB into carry flag and brings original carry flag in the LSB position and that is not what we want.