CIRCULARLY LINKED LISTS IMPLEMENTATION
A linked list wherein the last element points to the first element is called as CIRCULAR linked list. The chains do not specified first or last element; last element does not have the NULL pointer. The external pointer provides reference to starting element.
The possible operations on a circular linked list are following:
Ø Insertion,
Ø Deletion, and
Ø Traversing
Figure indicates a Circular linked list.
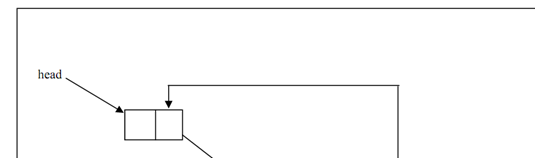
Figure: A Circular Linked List
Program indicates the creation of a Circular linked list.
#include
#include
#define NULL 0
structlinked_list
{
int data;
structlinked_list *next;
};
typedefstructlinked_listclist;
clist *head, *s;
void main()
{
voidcreate_clist(clist *);
int count(clist *);
void traverse(clist *);
head=(clist *)malloc(sizeof(clist));
s=head;
create_clist(head);
printf(" \n traversing the created clist and the starting address is %u \n", head);
traverse(head);
printf("\n number of elements in the clist %d \n", count(head));
}
voidcreate_clist(clist *start)
{
printf("input the element -1111 for coming out of the loop\n");
scanf("%d", &start->data);
if(start->data == -1111)
start->next=s;
else
{
start->next=(clist*)malloc(sizeof(clist));
create_clist(start->next);
}
}
void traverse(clist *start)
{
if(start->next!=s)
{
printf("data is %d \t next element address is %u\n", start->data, start->next);
traverse(start->next);
}
}
if(start->next == s)
printf("data is %d \t next element address is %u\n",start->data, start->next);
int count(clist *start)
{
if(start->next == s)
return 0;
else
}
return(1+count(start->next));