For good understanding of the application of the rules specified above see the following figure, where the shaded region demonstrates the clipped polygon.
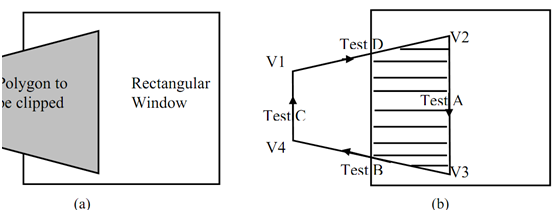
Figure: Sutherland-Hodgman Polygon Clipping
Pseudo code for Sutherland - Hodgman Algorithm
Define variables
In VertexArray is the array of input polygon vertices
outVerteArray is the array of output polygon vertices
Nin is the number of entries in inVertexArray
Nout is the number of entries in outVertexArray
n is the number of edges of the clip polygon
ClipEdge[x] is the xth edge of clip polygon defined by a pair of vertices
s, p are the start and end point respectively of current polygon edge
i is the intersection point with a clip boundary
j is the vertex loop counter
Define Functions
AddNewVertex(newVertex, Nout, outVertexArray)
: Adds newVertex to outVertexArray and then updates Nout
InsideTest(testVertex, clipEdge[x])
: Checks whether the vertex lies inside the clip edge or not;
retures TRUE is inside else returns FALSE
Intersect (first, second, clipEdge[x])
: Clip polygon edge (first, second) against clipEdge[x], outputs the intersection point
{ : begin main
x = 1
while (x ≤ n) : Loop through all the n clip edges
{
Nout = 0 : Flush the outVertexArray
s = inVertexArray[Nin] : Start with the last vertex in inVertexArray
for j = 1 to Nin do : Loop through Nin number of polygon vertices (edges)
{
p = inVertexArrray[j]
if InsideTest(p, clipEdge[x] = = TRUE then : Case A
and D
if InsideTest(s, clipEdge[x] = = TRUE then
AddNewVertex(p, Nout, outVertexArray) : Case A
else
i = Intersect(s, p, clipEdge[x]) : Case D
AddNewVertex(i, Nout, outVertexArray)
AddNewVertex(p, Nout, outVertexArray)
end if
else : i.e. if InsideTest(p, clipEdge[x] = = FALSE
(Cases 2 and 3)
if InsideTest(s, clipEdge[x]) = =TRUE then : Case B
{
Intersect(s, p, clipEdge[x])
AddNewVertex(i, Nout, outVertexArray)
end if : No action for case C
s = p : Advance to next pair of vertices j = j + 1
end if : end {for}
}
x = x + 1 : Proceed to the next ClipEdge[x +1]
Nin = Nout
inVertexArray = outVertexArray : The output vertex array for the current clip edge becomes the input vertex array for the next clip edge
} : end while
} : end main