Reference no: EM132364416
Programming Task
Background
For this assessment, you will be required to write a simple budget Python program using techniques discussed in class.
It is expected that you program all the input and output yourself, making the experience seem like that of a standard command line application. Students are required to follow methods discussed in class, other methods won't be necessarily penalised, but penalties may occur if they clearly are against the spirit of the assessment.
DO NOT worry about errors due to the user inputting incorrect (or unexpected) values (e.g. a string where an int is expected), you don't need to counter them. Though, there should be no errors when the user actually inputs what is expected. Money can either be an int (whole number, e.g. 1, 2, 3, 50, 70, 100 etc) or floats (decimal number, 1.0, 2.55, 3.8457, 100.2 etc), it doesn't matter! Feel free to choose.
The user will be asked for (on a monthly basis)
• The name of their company
• The amount of rent their company must pay
• The total amount their company spends on goods and/or services
• The total amount of salary their company pays its employees
• The total amount of income their company receives from clients/customers (before tax)
• The tax in decimal (i.e. 10% = 0.1, 50% = 0.5) their company must pay from their income
The program should then output
• Some form of title (or notice) which includes the company name
• Total expenditure per month (= rent + goods/services + salary)
• Income after tax (= gross income - [tax * gross income], or, = [1 - tax] * gross income)
• Net profit per month (= income after tax - expenditure)
• Net profit per year (= net profit per month * 12)
• Some kind of remark or note regarding the current situation of the company, for example if it's making a lot of profit you may mention it, or if it's losing money you may mention it. Any kind of statement is generally okay, so long as it's appropriate; be creative! :) (clearly this must use if statements to function).
The below is generally how your program's output should look (captured directly from Python IDLE), note that the black text is user input.
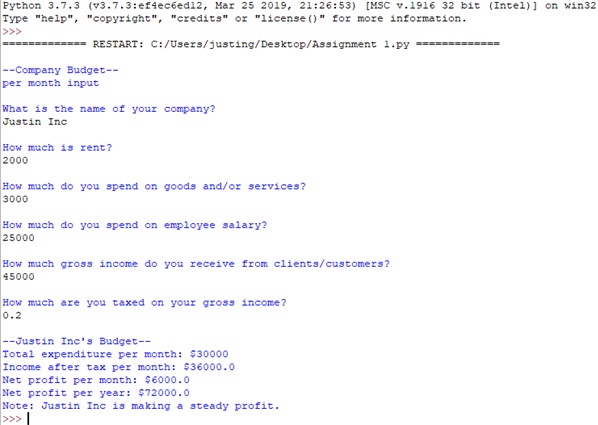
Hints
• Use print("...") for the general output, remember to use print() by itself if you want to add more lines between outputs.
• While all money may be represented using ints, the value of tax must be a float as it's a decimal value.
• User input is captured by the input("...") function.
• Please review the user input content.
o Get string from user -> name = input()
o Get int form user -> rent = int(input())
o Get float from user -> tax = float(input())
Presentation
Assignment files must run without error through the Python interpreter.
Make sure the code is readable. This includes utilizing meaningful variable names and comments, making sure it is spaced out and that lines are not too long (if they start to become too long, break them up. A general rule is each line shouldn't be more than around 80 characters long).