Reference no: EM132799559
Python Programming Assignment -
Section 1 - short answers, you should type your answers either in this Word document or as a separate text file such as created in notepad. Neatness and clarity matter in your answer.
1. Given: B = (3.5, 3.7, 5.0, 10.6, 25.3, 50.6)
a. What is wrong with: B[3] = 10.6?
b. Write output for: print('Slice sum = {mySum:06.2f}'.format(mySum=sum(B[-3:1:-1])))
c. Write an equivalent line of code to the above print statement using all positive numbers for slicing.
d. Is a, b, c = B[-1:-5:-1] allowed?
e. If d. is allowed, what is the value of b? If d. is not allowed, correct the statement (by changing only one character) and tell the value of b.
2. True or False: (about Python)
a. A tuple can be redefined within its original scope?
b. Python relies on indentation to define scope in the code?
c. def is a keyword used to identify a block of code that runs only when called?
d. A callback function cannot be defined inside of another function?
e. A lambda function can only take one argument?
3. a. Write a single line of code that will produce a 6×6 identity matrix (list of lists).
b. Modify your line of code from a. to fill all the non-diagonal terms with a formatted string of the form 'r,c' where r is the row index and c is the column index in the matrix.
Section 2 - solutions problems 4 - 6 should be written in separate Python files titled EX1-4.py, EX1-5.py, EX1-6.py.
4. An exercise in producing pseudorandom numbers, indexing, and data check/verification. Write a Python program to generate a 10×10 matrix filled with random integers in the range of 0 to 100 (without duplicate numbers).
Output your 10×10 matrix to the console in the format:
A=
[82, 18, 74, 50, 33, 16, 8, 92, 60, 85]
[23, 78, 5, 75, 6, 1, 66, 32, 19, 54]
[91, 55, 28, 90, 36, 52, 0, 65, 30, 14]
[79, 9, 63, 94, 58, 96, 24, 71, 64, 37]
[35, 42, 17, 84, 98, 80, 10, 70, 7, 29]
[59, 25, 99, 11, 62, 87, 27, 51, 83, 40]
[81, 4, 41, 3, 2, 76, 26, 39, 73, 88]
[86, 45, 21, 48, 97, 61, 67, 68, 93, 89]
[77, 46, 38, 57, 13, 22, 72, 34, 47, 31]
[95, 44, 12, 53, 69, 15, 49, 20, 56, 43]
Seed values = [1234,19857,25000]
Verified 0 duplicated numbers!
5. a. Use your pseudorandom number generator from question 4 to generate a list of 1000 random floating point numbers between 0 and 1 (duplicates are okay). Consider these values to represent probability levels that can be found by integrating the Gaussian probability density function (PDF) between
x = μ-5⋅σ to x=xi such that pi = P(x<xi|N(μ,σ)).
b. Using your Simpson function (nPoints = 50) to integrate the Gaussian PDF combined with your Secant method, find the set of x values compatible with your list of 1000 probabilities.
c. Finally, calculate the estimates of the population parameters μ and σ2 using the unbiased sample estimators from you set of x values. Compare your values to those from N(175, 15).
Output the values for your population estimators like:
Population mean estimate = y.yy
Population variance estimate = z.zz
6. The following describes a tiny part of the airplane design process - Choosing the engine size needed to meet a take-off distance requirement (STO). STO is the distance an airplane will roll on the runway before it is able to lift off into the air. Calculating STO is performed using a sequence of five equations shown below. Those equations require seven parameters that control takeoff performance. The engine thrust parameter is a major factor in determining take-off distance. The graph shows how take-off distance gets shorter as engine thrust is increased.
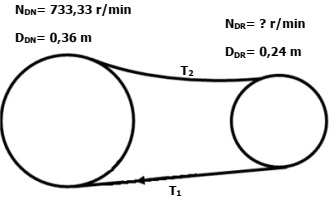
Write a Python program that includes and calls the following three functions:
a) def STO(thrust):
Thrust: the value of the engine thrust. Important, this is the only argument to be passed to this function. All of the other required airplane parameters may be assumed constant. Local variables for those parameters may be defined and assigned values inside the STOfunction. Use the airplane parameter values given in the figure above.
The function returns: the airplane take-off distance, calculated using the five equations given above. The first four of those equations calculate the value of three constants to be used in the fifth equation (VTO, A and B). That fifth equation requires the use of numerical integration. Use your Simpson function to perform the integration.
b) def ThrustNeededForTakeoff(distance):
distance: the required take-off distance. Important, this is the only argument to be passed to this function. All of the other required airplane parameters may be assumed constant. Local variables for those parameters may be defined and assigned values inside the ThrustNeededForTakeofffunction.
The function returns: the engine thrust needed to allow the airplane to take-off in the specified distance. Hint: The ThrustNeededForTakeofffunction behaves as the inverse of the STOfunction. Therefore it must use a root-finding method to find the value of thrust that causes: STO(thrust)-distance = 0. Use your Secant function to solve for this value of thrust.
c) def main():
main() has no arguments and no return value.
main() does the following:
a) calls STO(13000)to calculate the take-off distance for an engine thrust of 13000 pounds. Print the answer with one decimal place, using a nice text label. The nice text label should include the thrust value of 13000 pounds.
b) calls ThrustNeededForTakeoff(1500)to calculate the thrust needed to allow takeoff in 1500 feet. Print the answer with two decimal places, using a nice text label. The nice text label should include the takeoff distance of 1500 feet.
c) calls ThrustNeededForTakeoff(1000)to calculate the thrust needed to allow takeoff in 1000 feet. Print the answer with two decimal places, using a nice text label. The nice text label should include the takeoff distance of 1000 feet.
Attachment:- Python Programming Assignment File.rar