Reference no: EM132348732
Programming Assignment -
Background - The belligerent country of Kablamistan has launched an inter-continental ballistic missile towards a country 10000km away which threatens to destroy the peaceful Goners who live there.
Fortunately the United Nations has anticipated this possibility and out of concern for the citizens of Goneria has placed a high-powered laser at the midpoint between the site of the missile's launch (Launchtown, Kablamistan) and the target (Doomsville, Goneria). The laser is capable of destroying the missile in mid-air.
Unfortunately the laser's guidance system got fried when the soldier monitoring the system blew its fuse when trying to cook a pop-tart with the laser and no replacement component could be found. Given the military's well known policy of awarding contracts to the lowest bidder, you've been given the job of developing software to upload into the 80286 computer that will be used to drive the laser!
The diagram below illustrates the situation.
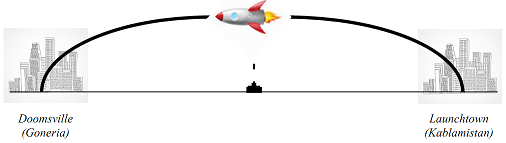
The technical specifications are as follows:
The missile
- is fired at Doomsville at 12:30PM, i.e. at lunchtime in Launchtown!
- flies an elliptical path (with a major radius of 5000km and a minor radius of 50km) which is 15709km long.
- travels each of the 180 x 1° segments in which it can be targeted by the laser in 43.635s; and
The laser
- is 5000km between Launchtown and Doomsville directly below the path of the missile;
- requires 65s to charge before it may be fired;
- moves in 1° increments - where 0° is along the ground to Launchtown, 90o is straight up, and 180o is along the ground to Doomsville;
- requires 35s to move 1o (and starts perpendicular to the ground, i.e. at 90o);
- should only be fired at degrees in the range 1-179o;
- may move while being charged; and
- fires instantly for an infinite distance destroying anything in its path.
Given an angle t in the range of 0-180o for the direction of the laser, the position of the missile on its elliptical path is the coordinate (x, y) where x = 5000*Math.cos(r) and y=50*Math.sin(r). r is the value t converted to radians and the conversion can be done with a built-in method: r=Math.toRadians(t);
If the missile will be at this coordinate at the time the laser is aiming there then this is a firing solution. Firing solutions consist of:
- the time the laser should be fired;
- the degree at which the laser should be aimed; and
- the resulting coordinate at which impact with the missile would occur.
Program specification - You and your partner must write a Java program to calculate and display all possible firing solutions. Firing solutions should be determined in order (from the greatest distance from Doomsville to the least distance from Doomsville).
Your solution should run in Dr Java and comprise the following files:
- TimeInterface.java and Time.java - the Time ADT as used in lectures and tutorials, and extended with a method to add seconds to an existing time. The heading for this additional function should be: void addSeconds(double s);
Only the whole number portion of the double value should be added to the time object;
- FiringSolutionInterface.java and FiringSolution.java - the specification and implementation (respectively) of a firing solution. A firing solution should be implemented as a class with instance variables which consist of:
- the time the laser should be fired (as a Time value);
- the degree at which the laser should be aimed (as an int); and
- the resulting coordinate at which impact with the missile would occur (as two double fields, x and y).
and the operations should include a getter and setter for each field.
- AssigOne219.java - the file which contains the main() method and other functions which implement the required task.
A starting point is available on MyLO for you to download. You should complete all of the required files. You may need nextLine() and nextInt() from java.util.Scanner, print() and println() from java.lang.System, compareTo() and charAt() from java.lang.String, valueOf() from java.lang.Integer, and format() from java.text.DecimalFormat. You will also need to use if and if-else statements, and for and while loops.
Algorithm - AssigOne219.java should implement the following algorithm:
setting up the launch time
iterating through each position (degree) of the missile from Launchtown to Doomsville and for each
iterating through the following steps until a solution is found or it is determined there are no solutions
calculating how the laser would need to move to get there
calculating how the missile would need to move to get there
determining how many seconds the movement of each item would take and, if the laser can get there no later than the missile
- determine the (x,y) coordinate of impact and the time
- create a firing solution of these values
- display the time and firing solution (you can make use of the defined DecimalFormat objects to format the output of Time and FiringSolution objects)
otherwise
- moving the missile to the next location (degree)
Program Style - Your program should follow the following coding conventions:
- final variable identifiers should be used as much as possible, should be written all in upper case and should be declared before all other variables
- variable identifiers should start with a lower case letter
- every if and if-else statement should have a block of code (i.e. collections of lines surrounded by { and }) for both the if part and the else part (if used)
- every do, for, and while loop should have a block of code (i.e. {}s)
- the keyword continue should not be used
- the keyword break should only be used as part of a switch statement
- opening and closing braces of a block should be aligned
- all code within a block should be aligned and indented 1 tab stop (or 4 spaces) from the braces marking this block
- instance variables should be used sparingly with parameter lists used to pass information in and out of functions
- local variables (excluding loop counters) should only be declared at the beginning of methods (either as parameters or otherwise)
Note - There should be a block of header comment which includes at least - file name, student names, student identity numbers, a statement of the purpose of the program, date, the percentage of the work completed by the authors - 50:50 is expected and assumed but reasons should be given if it is more/less than this and Each variable declaration should be on a single line and should be commented. There should be comments that identify groups of statements that do various parts of the task. Comments should describe the strategy of the code and should not simply translate the Java into English.
Attachment:- Programming Assignment Files.rar