Reference no: EM132097648
Engineering Computing Assignment -
Question 1: Numerical vs. Analytical Solution
The exact solution of the falling parachutist problem can be expressed as:
v(t) = g(mman+mchute)/c[1-e(-ct/mman+mchute)]
where, v is the velocity, g is the gravity, mman and mchute are the mass of the parachutist and the parachute respectively, c is the drag coefficient, and t the time.
(a) Write a MATLAB script/function and use symbolic programming to find the exact solution from t = 0 to t = 50.0 seconds. Use g = 9.81 m/s2, mman = 84.0 kg, mchute = 31.0 kg and c = 75.0 kg/s. Assume v = 0.0 at t = 0.0. Plot the exact solution (v vs t).
(b) The numerical solution to the same problem can be computed using Euler's method with the equations below:
vt_(i+1) = vt_i + dv/dt(ti+1 - ti)
with dv/dt = g - (c/(mman+mchute))vt_i
Implement this in MATLAB and find a time step for the numerical solution that brings the largest error between the numerical and analytical velocities to under 1.0 m/s. Plot the numerical solution over the exact solution from (a).
(c) Use your implementation of the numerical method to find a value of the drag coefficient c, that results in a steady-state velocity of less than 10m/s. Plot the solution over those from (a) and (b).
Question 2: Design of a Rollercoaster
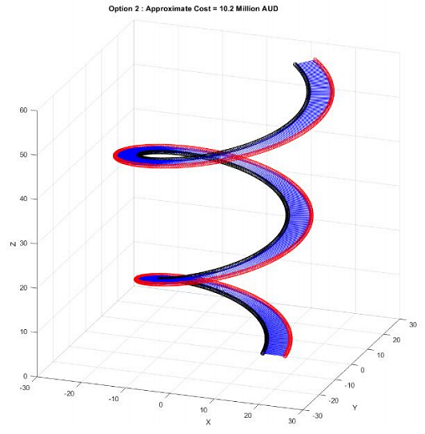
You work at an engineering firm that specialises in the design of rollercoasters. The hard work is already over! Your colleague has designed a rollercoaster for a client and it is your job to present the results to the client. Here is the information that you receive from your colleague.
The rollercoaster consists of an inner and outer track, the 3D profile of which are based on a helix as follows:
x = βcos(αθ)
y = βsin(αθ)
z = θ
For the inside track, βinner linearly increases from 10.0 to 20.0 m. For the outside track, βouter linearly increases from 15.0 to 25.0 m. For both tracks, θ linearly increases from 0 to 20*pi radians. The variable α controls the overall shape and number of rotations of the tracks.
(a) Write a function in MATLAB that computes and plots the inner and outer tracks in 3D. Join corresponding points on the inner and outer tracks with lines (e.g. see figure above). Plot the changing track shapes using α values ranging from 0.1 to 0.5 in steps of 0.125 (use a new figure or subplot for each α value). Hint: Use the linspace, plot3 and plot commands. Additional useful commands may be grid, hold, axis, view, xlabel, title, subplot etc.
(b) Compute the total path length for each of the track shapes above. Assuming that each meter of track costs 40000 AUD to construct, calculate the total cost of constructing each of them and print this cost in millions of AUD in the figure title (e.g. see figure above).
Hint: Use an average βmiddle ranging from 12.5 to 22.5 m. Compute the path and approximate it with a series of small line segments to get the total length as the sum of individual lengths.
Question 3: Beam Deflection Analysis
The deflection y of a beam subject to a tapering load w, may be computed as
y(x) = w/120EIL[-x5+2L2x3 - L4x]
Use w = 122.35 kN/cm, L = 150 cm, E = 27000 kN/cm2, I = 35000 cm4.
(a) Compute and plot the beam deflection, y, along the beam length, x, as well as the rate of change of deflection (dy/dx).
(b) Implement the bracketing method "false position" and use the plots from step (a) to choose a sensible bracketing window. Find the point of maximum deflection (where dy/dx = 0), and compute the x and y(x) values at this point. DO NOT use the MATLAB built-in function fzero. Write your own bracketing function that implements the false-position method. Use εs = 0.01%.
Hint: Use the MATLAB examples from Week 3's lecture as a template.
(c) Use fprintf to print the bracketing results for each iteration as values of: [iteration_number xl xu xr f(xl) f(xr) f(xl)f(xr) εa].
Attachment:- Matlab Assingment File.rar