Reference no: EM132387926
Question 1
The following program compiles and runs. What is the output of this program? public class KitchenAppliance {
private float price; // the price of the appliance
private String type; // type of the appliance
private String make; // appliance make
// default constructor
public KitchenAppliance ()
{
price = 0.0f; type = "not set";
make = "unknown";
}
//parameterised constructor
public KitchenAppliance (float price, String type, String make) { this.price = price;
this.type = type; this.make = make;
}
@Override
public String toString() {
return String.format("KitchenAppliance price: %.2f, type: %s, make: %s ", price ,
type , make );
}
public static void main(String [] args ) {
KitchenAppliance defaultAppliance = new KitchenAppliance(); System.out.println(defaultAppliance);
KitchenAppliance myKettle = new KitchenAppliance(105.90f, "Stainless Steel
Kettle", "Beville");
System.out.println(myKettle);
}
}
Question 2
The following code defines class Publication, with a constructor that defines the type of the publication (journal, conference, book chapter) and year of publication, and a toString() method that returns a String containing the Publication's type and year.
public class Publication {
private String publicationType; private int year;
public Publication (String publicationType, int year) { this.publicationType = publicationType;
this.year = year;
}
@Override
public String toString()
{
return String.format( "Publication Type: %s, Year: %d", this. publicationType,
this.year );
}
}
Write java code for the four methods given below and a main method to create a Publication object having publication type - journal, and year - 2016.
• Two set methods to set the values of publicationType and year.
• Two get methods to return the publicationType and year.
Question 3
Use the Publication class in Question 2 (repeated below) to create a subclass called JournalPublication. The JournalPublication class will have additionally:
• Two private data members, journalName of type String to store the name of the journal (eg. Applied Soft Computing), and impact factor of type float (eg. 2.86).
• A constructor that uses the super class constructor.
• A toString() method that uses the super class toString() method and additional format specifiers to display the private data member values.
public class Publication { private String publicationType; private int year;
public Publication (String publicationType, int year) { this.publicationType = publicationType;
this.year = year;
}
@Override
public String toString()
{
return String.format( "Publication Type: %s, Year: %d",
this.publicationType, this.year );
}
}
Question 4
The source code given below has some missing lines of code as indicated. Complete the missing lines of code in the anonymous inner class for JComboBox event handling so that as the user selects a publication title, the publication type of that title should be displayed in the JTextField, txtpublicationType as shown in Figure 1 below.
import java.awt.*; import javax.swing.*; import java.awt.event.*; import java.util.*;
public class PublicationGui extends JFrame {
// GUI component variables private JLabel lblAuthorName; private JTextField txtAuthorName; private JLabel lblpublicationTitle;
private final JComboBox<String> cmbTitlesJComboBox; // for publication titles
private JLabel lblpublicationType; private JTextField txtpublicationType;
// list of publication titles (3), and corresponding public types.
private static final String[][] publications = { {"Intelligent System for Personal Diet Management", "Book Chapter"}, {"Family System for Home Automation Applications", "Journal"}, {"User Requirement for Technology Investment", "Conference"}};
public PublicationGui () {
// GUI components initialised
super("Publication List ");
JPanel topPanel = new JPanel (new GridLayout(2,1)); // Panel with 2 rows
lblAuthorName = new JLabel("Author Name"); txtAuthorName = new JTextField(30); lblpublicationTitle = new JLabel("Publication Title"); String [] articles = new String[3];
int i = 0;
for (String [] titles: publications)
articles[i++] = titles[0]; //creates an array of titles
//initialise to display titles
cmbTitlesJComboBox = new JComboBox<String>(articles); cmbTitlesJComboBox.setMaximumRowCount(5); lblpublicationType = new JLabel("Publication Type"); txtpublicationType = new JTextField(30);
JPanel RowOnePanel = new JPanel(new FlowLayout()); JPanel RowTwoPanel = new JPanel(new FlowLayout()); RowOnePanel.add (lblAuthorName);
RowOnePanel.add (txtAuthorName);
RowTwoPanel.add (lblpublicationTitle); RowTwoPanel.add (cmbTitlesJComboBox); RowTwoPanel.add (lblpublicationType); RowTwoPanel.add (txtpublicationType);
topPanel.add (RowOnePanel); topPanel.add (RowTwoPanel);
add (topPanel, BorderLayout.NORTH);
// anonymous inner class for JComboBox event handling
// as the user selects a publication title, the publication type of that title should be
// displayed in the JTextField, txtpublicationType as shown in Figure 1 below.
cmbTitlesJComboBox.addItemListener( new ItemListener() {
// student to complete missing code
......................
.......................
......................
} //end anonymous inner class
); // end call to addItemListener
}
public static void main(String args[]) { JFrame testFrame = new PublicationGui();
testFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); testFrame.setSize(600, 200);
testFrame.setVisible(true);
}
}

Figure 1 Publication Gui output
Question 5
The following program is based on linked list operations. What will be the output when the program given below is executed?
import java.util.LinkedList; import java.util.ListIterator; public class PublicationTypes {
public static void main(String[] args)
{
LinkedList<String> publicationTypes = new LinkedList<String>(); ListIterator<String> iter;
publicationTypes.add("Book Chapter"); System.out.println("List: "+ publicationTypes);
publicationTypes.addFirst("Journal Article"); publicationTypes.addLast("Conference Paper"); System.out.println("List: "+ publicationTypes);
int index = publicationTypes.indexOf("Book Chapter"); publicationTypes.set(index,"Book" ); publicationTypes.addFirst("Book Chapter"); System.out.println("List: "+ publicationTypes);
iter = publicationTypes.listIterator(); iter.next();
iter.next(); iter.add("Patents");
System.out.println("List: "+ publicationTypes);
}
}
Question 6
(a) By using the definition of Big-O, show that the running time term T(n)=78+2n + n2 is O(n2 ). Write your answer in no more than three or four lines.
(b) For the following running time expressions, what would be the order of the growth rate of each in terms of Big-O notation?
i. 2n +5n
ii. n3 + 2n-1 +5 log2n
iii. 2n2 +10√n +15
Question 7
Suppose there is a comma separated values file (.csv) - journals.csv with data format (journalId , journal Name, impactFactor) as given below:
j01, Expert Systems, 2.45
j02, Applied Soft Computing, 2.58
The following program contains the partial code. Complete the program code so that it can read the data file (journals.csv) and display the details of the highest impact factor journal:
• Journal Name
• Impact Factor.
// Partial Code import java.io.*; import java.util.*;
public class JournalFile { private static Scanner input;
public static void main(String[] args) { String highestImpactJournal = ""; String journalId = "",
journalName = "", line ="";
String[] temp = new String[3]; float impactFactor = 0.0f; float highestImpact =0.0f;
File journals; // open file for reading and throw exception for failure to open.
try {
journals = new File("journals.csv"); input = new Scanner(journals);
} catch (FileNotFoundException fileNotFoundException) {
// Student to complete exception handling code
}
try {
// read file, compute the name and impact factor of the journal with highest
// impact factor and display these details
while (input.hasNextLine()) {
// Student to complete
// Read each line
//spilt the comma separated values and store in an array of Strings
journalId = temp[0]; journalName =temp[1];
impactFactor = Float.parseFloat(temp[2]);
// Student to complete
// find the highest impact factor and corresponding journal name
}
}
catch (NoSuchElementException elementException) { System.err.println("File improperly formatted. Terminating.");
}
catch (IllegalStateException stateException) { System.err.println("Error reading from file. Terminating.");
}
// Student to complete: display journal name with the highest impact factor
// Student to complete: close file.
} // end main
} //end class
Question 8
The SetQueue() class given below has method enQueue() to implement the enqueue operation. Write the missing lines of code as indicated in the source code below. Write the output of the program.
import java.util.SortedSet; import java.util.TreeSet; import java.util.Iterator; import java.util.LinkedList;
public class SetQueue {
private double values[] = {2.5, 1.2, 1.5, 5.6, 1.5, 8.7, 9.5, 2.5}; private LinkedList<Double> queue;
private SortedSet<Double> ordered;
public SetQueue() {
queue = new LinkedList<Double>(); // LinkedList to be used as a queue
ordered = new TreeSet<>(); //creates a set
}
public void enQueue()
{ //Student to complete Use appropriate Linked list method to fill the queue
// with Array elements of values[] given above.
............................
}
public void displayQueueFillSet() { System.out.println("Queue contains:"); while(queue.size()> 0)
{ // Student to complete display queue elements and remove these
//elements from queue and add to the set, ordered given above.
.............................................
}
System.out.println();
}
public void displaySet() {
Iterator <Double> pointer = ordered.iterator(); System.out.println("Set contains:");
while (pointer.hasNext()) { System.out.printf("%.2f, ",pointer.next());
}
}
public static void main (String[] args) { SetQueue set = new SetQueue(); set.displayQueueFillSet(); set.displaySet();
}
}
Question 9
The following program creates a class named GenericStack and then uses it to create a Stack of Strings. The Stack of Strings should be populated with the values from the Array of Strings, words given below. Complete the missing lines of code.
import java.util.LinkedList; public class GenericStack <T> {
private LinkedList <T> elements; public GenericStack() {
elements = new LinkedList <T> ();
}
public void push (T value)
{ // Student to complete the code to push value to the stack
//Use appropriate LinkedList method
}
public static void main(String [] args)
{
String [] words = {"push", "pop", "pull", "put", "post"};
//Student to complete: create a Stack <String>
//Student to complete: push values from the words Array into the
//Stack <String>
}
}
Question 10
In the binary search tree shown below certain values are misplaced by not following the binary search tree rules. Correct the misplaced values.
List the values of A and B in the tree shown below so that it is a binary search tree. Manually provide the inorder, preorder and postorder traversals of the binary search tree with values of A, B, and all values correctly placed.
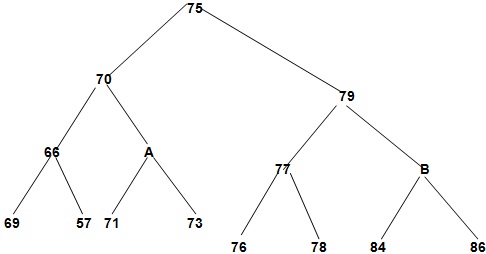
Question 11
Write a method to calculate the sum of numbers 1 to n recursively. For example, if n is 4, sum of 1 to 4 , will be 10 and the method should return 10. Write a main method to test your method.
Attachment:- Data Structures and Algorithms Exam.rar