Reference no: EM132387942
Question 1
The following program compiles and runs. What is the output of this program?
public class Rectangle
{
private double length; private double width;
public Rectangle()
{
length = 1.5;
width = 2.5;
}
public Rectangle( double theLength, double theWidth )
{
length = theLength; width = theWidth;
}
public double getLength()
{
return length;
}
public double getArea()
{
return (length * width);
}
public boolean isSquare()
{
return (length == width);
}
public static void main( String[] args )
{
Rectangle myRec1 = new Rectangle(2.0, 3.0); System.out.println("The length is: " + myRec1.getLength()); System.out.println("The area is: " + myRec1.getArea()); System.out.println("Is it a square? " + myRec1.isSquare()); Rectangle myRec2 = new Rectangle(); System.out.println("The area is: " + myRec2.getArea()); System.out.println("Is it a square? " + myRec2.isSquare());
}
}
Question 2
The following code defines class Car, with a constructor that specifies the car's model (carModel) and price (carPrice), and a toString method that returns a String containing the car's model and price.
public class Car
{
private String carModel; private double carPrice;
public Car(String model, double price)
{
carModel = model; carPrice = price;
}
public String toString()
{
return String.format( "%s price %.2f", carModel, carPrice );
}
}
Your tasks are to:
• define two set methods to set the carModel and carPrice.
• define two get methods to retrieve the carModel and carPrice.
• create a Car object with the model name "Ford" and the price 20000.00, and display a String containing the value of the object's instance variables.
Write java code for the above mentioned tasks.
Question 3
Based on the following Java programs, what will be the output when the
PayrollSystemTest is executed?
public abstract class Employee
{
private String firstName; private String lastName;
public Employee( String first, String last)
{
firstName = first; lastName = last;
System.out.println(firstName +" "+lastName);
}
public abstract double earnings();
}
public class HourlyEmployee extends Employee
{
private double wage; private double hours;
public HourlyEmployee(String first, String last, double hWage, double hWrk)
{
super( first, last); wage = hWage; hours =hWrk;
}
public double earnings()
{
System.out.println( "Earnings method starts..." ); if ( hours <= 50 )
return wage *hours; else
return 50 * wage + (hours - 50 ) * wage;
}
}
public class PayrollSystemTest
{
public static void main( String[] args )
{
Employee cEmployee = new HourlyEmployee("Mik", "Ton", 10, 51 ); System.out.println( "Calculate Employee Earnings \n" ); System.out.printf("Employee earned $%,.2f\n\n", cEmployee.earnings() );
}
}
Question 4
What will be the output when the following program is executed? Show all GUI components including frames, combo boxes, buttons and message dialog boxes.
import javax.swing.*; import java.awt.*; import java.awt.event.*;
public class UnitTimetable extends JFrame
{
private JPanel panel; private JButton exitButton;
private JComboBox dataBox;
public UnitTimetable()
{
super("Timetable for programming units..."); setSize(500, 400); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); dataBox = new JComboBox();
dataBox.addItem("Unit COIT20245"); dataBox.addItem("Unit COIT20256"); exitButton = new JButton("EXIT");
dataBox.addActionListener(new DataComboBoxListener()); exitButton.addActionListener(new ExitButtonListener());
panel = new JPanel(); panel.add(dataBox); panel.add(exitButton); add(panel);
setVisible(true);
}
private class DataComboBoxListener implements ActionListener
{
public void actionPerformed(ActionEvent e)
{
int index = dataBox.getSelectedIndex(); switch (index)
{
case 0: JOptionPane.showMessageDialog(null, "Thur 4-6pm"); break; case 1: JOptionPane.showMessageDialog(null, "Mon 1-3pm"); break;
}
}
}
private class ExitButtonListener implements ActionListener
{
public void actionPerformed(ActionEvent e)
{
System.exit(0);
}
}
public static void main(String[] args)
{
UnitTimetable mFrame= new UnitTimetable();
}
}
Question 5
The following program is based on linked list operations. What will be the output when this program is executed?
import java.util.List; import java.util.LinkedList;
import java.util.ListIterator;
public class LinkedListTest
{
public static void main(String[] args)
{
LinkedList<String> myList = new LinkedList<String>(); ListIterator<String> iter;
myList.add("Brisbane"); myList.addLast("Rocky"); System.out.println("List: "+myList); myList.addLast("Sydney"); myList.addLast("Perth"); System.out.println("List: "+myList); iter = myList.listIterator(); iter.next(); iter.next();
iter.add("Mackay"); iter.add("Melbourne"); System.out.println("List: "+myList); iter.next();
while (iter.hasNext()) System.out.println("List: "+ iter.next()); iter.add("Canberra");
System.out.println("List: "+myList);
}
}
Question 6
(a) By using the definition of Big-O, show that the running time of term T(n)=112+3n+n2 is O(n2). Write your answer in no more than four lines.
(b) Assume that each of the following operators =, <, &&, [ ], ==, -- counts as an operation. What will be the worst-case running time in terms of total number of operations for the following code?
for (int i=N; i>0; i--) {
if ( (i>0) && (data[i] == 3) ) keyFound = 1;
}
Suppose there is a text file (pub.txt) with data format (publication name, publication type, ranking) as below:
Publication name Type Ranking
Pattern-recognition journal 1
Neurocomputing journal 2
International-conference-neural-networks conference 1
Question 7
The following program contains the partial code. Complete the program code so that it can read the data file (pub.txt) and display the following information on the screen.
• publications with ranking 2.
• total number of journal publications.
• total number of conference publications.
import java.io.*; import java.util.*; public class FileTest
{
public static void main(String[] args) throws java.io.IOException
{
int countJournal=0, countConference=0; try
{
File pubs=new File("pub.txt"); Scanner input=new Scanner(pubs); while (input.hasNext())
{
String pubName = input.next(); String pubType = input.next(); int pubRank = input.nextInt();
//Display publication (name, type, ranking) if ranking is 2
/*Student to COMPLETE*/
//Count journal publications and conference publications
/*Student to COMPLETE*/
}
//Display total number of journals and total number of conferences
/*Student to COMPLETE*/
//Close the file
/*Student to COMPLETE*/
}
catch (FileNotFoundException fileNotFoundException)
{
/*Student to COMPLETE*/
}
}
}
Question 8
What is the output from the following sequence of queue operations? Assume that the PriorityQueue class here is a class that has implemented standard Queue operations offer, peek and poll.
PriorityQueue<Integer> q = new PriorityQueue<Integer>(); int num1 = 50, num2 = 100;
q.offer(num1); q.offer(num2); q.poll();
System.out.println(q.peek()); q.poll();
q.offer(120); System.out.println(q.poll()); q.offer(num1); q.offer(num2); q.offer(num1); System.out.println(q.peek());
while (!q.isEmpty()) System.out.print(q.poll() + " ");
Question 9
The following code is a recursive method. Show how you work out the result of call calcValue(6,1).
public static int calcValue(int num1, int num2)
{
if (num1 <= num2)
return 0;
else
}
return calcValue(num1-1, num2) + 1;
Question 10
What are the values of A and B in the tree shown below so that it is a binary search tree? List the values of A and B. Manually provide the inorder, preorder and postorder traversals of the search tree shown below.
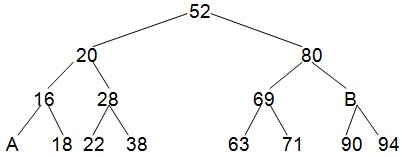
Attachment:- Examination.rar