Reference no: EM132387930
Question 1
The following codes define a class named Car. The instance variables of this class are the car make and model description - makeDes, and the car sale price - price. The class contains a parametrized constructor, two get/set methods, a method named isLuxury() to be defined and the method toString() to be defined.
public class Car
{
private String makeDes; private int price;
final int LUX_STANDARD=70000;
public Car(String des, int p)
{
makeDes = des; price = p;
}
public boolean isLuxury()
{
//................ To be defined
}
public String toString()
{
//............ to be defined
}
} //end class definition
Your tasks are:
• to define two set methods to set the makeDes and price of the Car.
• to define two get methods to retrieve the makeDes and price of the Car.
• to define the isLuxury( ) method. If the price is greater than 70000, the method returns true otherwise returns false.
• to define the toString() method that returns a string containing the value of instance variables as well as the information whether this car is luxury or not.
• to define a main method that creates a Car object with the makeDes "VW Tiguan 2017" and the price 47000, and display a string containing the value of the created object's instance variables.
Question 2
Based on the following Java programs, what will be the output when the ParcelDemo is executed?
public class Parcel
{
private double weight;
public Parcel(double w)
{
weight=w;
}
public double getWeight()
{
return weight;
}
public double calculateCost()
{
double cost=0.0; if(weight<1.0)
cost=2; else
cost=2+(weight-1)*1.5; return cost;
}
public String toString()
{
return "Ordinary parcel: "+weight+"kg, total shipping cost
tiny_mce_markerquot;+calculateCost();
}
}
public class InsuredParcel extends Parcel
{
private double insurance;
public InsuredParcel(double w)
{
super(w);
if(w<1.0)
insurance=5.0; else
insurance=10.0;
}
public double getInsurance()
{
return insurance;
}
public double calculateCost()
{
return getInsurance()+super.calculateCost();
}
public String toString()
{
return "Insured parcel: "+super.getWeight()+"kg, total
shipping cost tiny_mce_markerquot;+calculateCost();
}
}
public class ParcelDemo
{
public static void main(String[] args)
{
Parcel p1=new Parcel(0.5); System.out.println(p1.toString());
Parcel p2=new InsuredParcel(0.5); System.out.println(p2.toString());
Parcel p3=new Parcel(4); System.out.println(p3.toString());
InsuredParcel p4=new InsuredParcel(4); System.out.println(p4.toString());
}
}
Question 3
Suppose there is a text file named DriverRecord.txt with the data format (license number, first name, last name and demerit point) as below:
licenseNo FirstName LastName demerit-point
011292900 Ronny Sydney 1
303930776 Louise Park 8
508363737 Jack London 8
009876662 Steven Walnut 3
The following program contains the partial code. Complete the program code so that it can read the data file (DriverRecord.txt) and display the following information on the screen:
• driver's license number and full name who has(ve) 8 demerit points
• total number of drivers with 8 demerit points
• total number of drivers in the data file
import java.io.*; import java.util.*;
//definition of file test class public class FileTest
{
public static void main(String[] args) throws java.io.IOException
{
int count=0, countDemerit=0;
try
{
FileReader reader=new FileReader("DriverRecord.txt"); Scanner fileIn=new Scanner(reader);
while(fileIn.hasNext())
{
//write your code to complete the required task
//on the answer booklet
}
// write your code for required task on answer booklet
.................................... reader.close();
}
catch(FileNotFoundException e)
{
System.out.println("not found file error");
}
}
}//end of class definition
Question 4
The figure as shown below is a graphical user interface (GUI) that is related to a java application on general knowledge quiz. List all the javax.swing components in this GUI. Explain how the layout manager might be used for building this GUI. (Note: The javax.swing components must be written in correct case.)
Question 5
The following program is based on linked list operations. What will be the output when this program (LinkedListTest.java) is executed?
import java.util.LinkedList; import java.util.ListIterator; import java.util.Collections;
public class LinkedListTest {
public static void main(String[] args)
{
LinkedList<Stock> list = new LinkedList<Stock>(); ListIterator<Stock> iter;
String[] code={"CBA","ANZ","BHP","WOW","RIO"};
double [] price={81,30,24.5,25,75};
Stock[] s=new Stock[5];//create a Stock array for(int i=0;i<s.length;i++)
s[i]=new Stock(code[i],price[i]);
for(int i=0;i<s.length;i++) list.addLast(s[i]);
System.out.println("The List contains: "+list);
list.removeFirst(); System.out.println("The List: "+list);
list.set(0,new Stock("TLS",3.5)); list.addLast(new Stock("WES",42));
iter = list.listIterator();
System.out.print("The List contains: "+list.size()+ "stocks\n");
while(iter.hasNext()) System.out.print(" "+ iter.next());
Collections.reverse(list); System.out.println("\nThe List contains: "+list);
}
}
Note: see next page for the definition of the Stock class
//Stock class definition public class Stock
{
private String code; private double price;
//parametrised constructor public Stock(String c, double p)
{
code=c; price=p;
}
public String toString()
{
return code+" tiny_mce_markerquot;+price;
}
}
Question 6
Consider the following Java program:
import java.util.*;
public class UseStack
{
public static void main(String[] args)
{
int n=4;
Stack<Integer> s=new Stack<Integer>(); while(n>0)
{
s.push(n);
n--;
}
int result=1; while(!s.isEmpty())
{
int integer=s.pop(); result=result*integer;
}
System.out.println("result= "+result);
}
}
What value is displayed when this program executes? What mathematical function does this program evaluate? Write your answer in no more than three or four lines.
Question 7
What is the output from the following sequence of priority queue operations? Assume that the PriorityQueue class here is a class that has implemented standard priority queue operations offer, peek and poll.
PriorityQueue<String> q = new PriorityQueue<String>(); String c1="ADL", c2="SYD";
q.offer("BNE"); q.offer(c1); q.offer("CNS"); q.poll();
System.out.println(q.peek());
q.poll(); System.out.println(q.poll());
q.offer("ROK"); q.offer(c2); q.offer("MEL");
System.out.println(q.peek());
while(!q.isEmpty()) System.out.println(q.poll()+ " ");
Question 8
(a) Rank the following growth rate from slowest to fastest:
O(n)
O(long(n)) O(2n)
O(n3)
Briefly explain your answer.
(b) For the following run time expressions, what would be the order of the growth rate of each in terms of Big-O notation? (3 marks)
(i) n+log(n)
(ii) n2+10n+25
(iii) 2n+n2+100
Question 9
The following method binarySearch(int []a, int key) takes a sorted integer array and a search key as arguments. It searches the array using the binary search algorithm and returns the location of the matched element. If not found, it will return -1.
//binary search method for an integer array public static int binarySearch(int [] a, int key)
{
int low=0;
int high=a.length-1;
int middle=(low+high+1)/2; int location=-1;
do
{
if(key==a[middle]) location=middle;
else if(key<a[middle]) high=middle-1;
else
low=middle+1;
middle=(low+high+1)/2;
}while((low<=high) && (location==-1));
return location;
}
Develop a generic version of binarySearch()method that uses a generic type T array instead of an integer array, and a generic type T search key instead of the integer search key. The method then applies to an array of any object type.
Write a test program to test this method with the following arrays and search keys 50 and 7.7 respectively:
Integer [] intArray = {10,20,30,40,50}; Double [ ] dArray={1.1,2.5,3.3,7.7,9.9};
Question 10
The following diagram represents a binary search tree (BST), in which each node stores a name. Your task is to manually provide the inorder, preorder and postorder traversals of this tree. In addition, if the names Jack and Victoria are required to insert into two nodes on the tree so that the tree is still a binary search tree, where these two names should be placed? Draw your diagram.
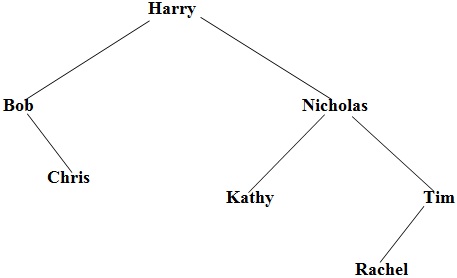
Question 11
What does the following program do? Justify your answer by showing the calls of the recursive method. What will be the output of running this program?
public class RecursiveTest
{
public static int f( int a, int n )
{
if ( n == 1 ) return a;
else
return a * f( a, n - 1 );
} // end method f
public static void main( String args[] )
{
int a=2; int n=4;
int result = f( a, n );
System.out.printf( "The result is %d\n", result );
} // end main
}// end of class definition
Attachment:- TERM 3 STANDARD EXAMINATION.rar