Reference no: EM132387933
Question 1
The following program is based on linked list operations. What will be the output when the program given below is executed?
import java.util.LinkedList; import java.util.ListIterator;
public class GraduandsList {
public static void main(String[] args)
{
LinkedList<String> graduands = new LinkedList<String>();
ListIterator<String> iter; graduands.add("William Bhullar"); System.out.println("List: "+ graduands);
graduands.addLast("Andrew Zhang"); System.out.println("List: "+ graduands);
int index = graduands.indexOf("William Bhullar"); graduands.set(index,"Sajan Tabang" ); graduands.addFirst("William Bhullar"); System.out.println("List: "+ graduands);
iter = graduands.listIterator(); iter.next();
iter.next(); iter.add("Prasad Patel");
System.out.println("List: "+ graduands);
}
}
Question 2
Write the missing lines of code and the output of the following program.
import java.util.HashSet; import java.util.Set;
public class Q2SetUnion {
static Set<Integer> setOne = new HashSet<>(); static Set<Integer> setTwo = new HashSet<>();
public static void main(String[] args) {
final Integer groupOne [] = { 2, 5, 17, 27, 7,37 };
final Integer groupTwo [] = { 3, 8, 15, 25, 5, 17};
//initialise sets
for (Integer temp: groupOne)
{
setOne.add(temp);
}
for (Integer temp: groupTwo)
{
setTwo.add(temp);
}
// Complete the following lines of code using the comments as a clue.
// Create a new set named union to store the values of the resulting union
// of the given two sets.
// implement union of the two sets.
// display the values in set named union.
Question 3
Complete the code given below to enable the following functionalities:
(a) To add all the numbers from the array ‘values' to the priority queue ‘q1'.
(b) Display the content of q1 (Hint: create a copy of the queue and use poll on the copy).
(c) Replace the number ‘2' with ‘5' in ‘q1'.
import java.util.PriorityQueue; public class PriorityQueue {
public static void main (String [] args)
{
PriorityQueue<Integer> q1 = new PriorityQueue<>();
Integer [] values = { 9, 2, 12, 7, 15};
//fill the queue q1 with elements from the array values.
// Write code to display the contents of queue q1.
// write code to replace the element ‘2' with ‘5' in q1.
} // end main
} //end class
Question 4
(a) By using the definition of Big-O, show that the running time term T(n)=100+2n+
n2 is O(n2).
(b) For the following running time expressions, what would be the order of the growth rate of each in terms of Big-O notation?
i) 2n+10n
ii) n2 + 10n log2n
iii) n+ 10√n +5
Question 5
Complete the missing lines of code in the program given below to display:
(a) Sorted values of the given array using Lambdas and streams.
(b) Display the String names in ascending order after filtering the values starting with g or less.
public class Q5LambdasAndStreams {
public static void main( String[] args )
{
int [] numbers = {14, 3, 27, 12, 7, 18, 57, 15, 8};
List<Integer> values = new LinkedList<>(); String[] names = {"Lily", "Lin", "Ann", "Bill",
"Michael", "Kay", "Sam"};
for (int n: numbers)
values.add(n);
//Dsiplay the sorted values.
// write your code
//Display names in ascending order after filtering the names strating with ‘g' or less.
// write your code
} //end main
} //end class
Question 6
Assume that a SQL server connection exists to a database named Restaurant. You are given the query to create a Table named Customers that stores customer name and customerId as primary key as shown in the incomplete code given below.
(a) Complete code to execute the given query to create the table.
(b) Use PreparedStatement to search a customer with a given name.
import java.sql.Connection; import java.sql.DatabaseMetaData; import java.sql.DriverManager;
import java.sql.PreparedStatement; import java.sql.ResultSet;
import java.sql.SQLException; import java.sql.Statement;
public class Q6Database {
public static void main(String[] args) {
final String MYSQL_URL ="jdbc:mysql://localhost:3306"; final String DB_URL = MYSQL_URL +"/Restaurant";
//initialise MySql usename and password final String USER_NAME ="tomm"; final String PASSWORD = "mtom0001"; Connection sqlConnection;
Statement statement; PreparedStatement searchOneCustomer;
// query string to create Customers table
final String TABLE_CUSTOMERS_QRY = "CREATE TABLE" +
"CUSTOMERS (CustomerId INTEGER not NULL" + "AUTO_INCREMENT,Name VARCHAR(30)," +
"PRIMARY KEY (CustomerId) )";
// sql driver is registered and connected to the database.
sqlConnection = DriverManager.getConnection(DB_URL,
USER_NAME, PASSWORD);
statement = sqlConnection.createStatement();
//complete the missing code to execute the query to create Customers table
If (!tblCustomersExist)
// write your code
//Write PreparedStatement to execute a query to searchOneCustomer
searchOneCustomer = // write your code
} //end main
} //end class
Question 7
The following program creates a class named GenericStack and then uses it to create a Stack of Strings. Complete the code for the method push() which takes a value of type T and adds to the elements in the GenericStack. Write code to populate the GenericStack with the values from the Array of Strings, cities given below using your push method.
import java.util.LinkedList; public class GenericStack <T> {
private LinkedList <T> elements; public GenericStack() {
elements = new LinkedList <T> ();
}
//Write code for the push method
//Use appropriate LinkedList method to add elements to the stack.
public static void main(String [] args)
{
String [] cities = {"bne", "mel", "syd", "crns" };
//Write code to create an object of type GenericStack
// Use the push method you have written to populate it with values from cities.
for (String s: cities)
// write missing code
} // end main
}// end class
Question 8
Write a recursive method, public int findSum(int n) which will recrusively calculate and return the sum of numbers 0 to n.
Question 9
What is the output from the following sequence of operations?
Queue<Integer> q = new LinkedList<>(); int a =10;
q.offer(5);
q.offer(a);
q.offer(15); System.out.println (q.peek()); q.poll();
q.offer(50);
Iterator iter = q.iterator(); while(iter.hasNext())
System.out.print(q.poll()+ " ");
Question 10
In the binary search tree shown below certain values are misplaced by not following the binary search tree rules. Correct the misplaced values.
List the values of A and B in the tree shown below so that it is a binary search tree. Manually provide the inorder, preorder and postorder traversals of the binary search tree with values of A, B, and all values correctly placed.
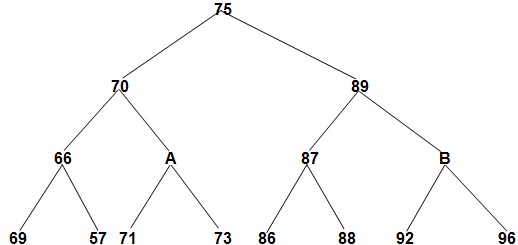
Attachment:- Term 1 Standard Examination 2018.rar