Reference no: EM133020602
QUESTION 1 Write a Python program that read a whole number from the user. You program should then print "negative" if the number is negative, "positive" if the number is positive, and "zero" if the number is zero. Draw the flow chart of your program.
QUESTION 2 Write a Python program which calculates a student's final grade based upon a single assessment item. The user should be asked to enter the mark for the assessment item and the maximum number of marks available. These values should then be converted into a percentage, and this percentage used to calculate the final mark which is then displayed to the screen. The cutoff values for final grades are as follows:
85-100 HD
75.84.99 D
65.74.99 C
50-64.99 P
<50 F
Note that if the user enters a negative grade or above 100, your program needs to return an error.
QUESTION 3
Access the Australian Taxation Office website and find the current tax tables for individuals. Write a program that asks the user to enter their tax file number (using a 'string data type) and then to enter their taxable income for the current financial year. If the user does not have an Australian tax file number, they should enter '0' instead.
Then, based on the taxable income, the program should return the tax payable on their taxable income. After you finish your program, draw a simple flowchart for it.
QUESTION 4
Write Python programs to implement each of the following flowcharts:
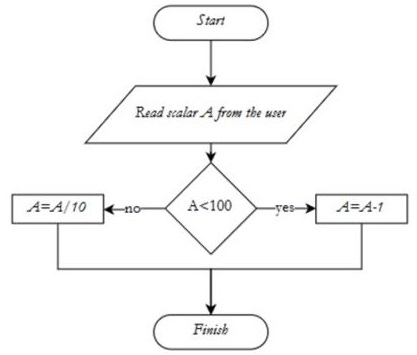
QUESTION 5
Write a program to prompt for and read a sequence of non-negative numbers (with a negative number to end input).
QUESTION 6
Write a program to prompt for and read a sequence of non-negative numbers (with a negative number to end input) and then print the least non-negative input number. If the first number read is negative, print "No numbers.". Round the least number off to one decimal place.
QUESTION 7
Write a program that simulates rolling a dice. The program shouid ask tne user how many die rolls they wish to do, and then print out that number of randomised die rolls on one line separated by spaces. Eventually, you
need to print out the summation of dice numbers. Note that you will have to use a definite loop for this question.
QUESTION 8 Write a guessing game where the user has to guess a secret number. After every guess the program tells the user whether his number was too large or too small. At the end the number of tries needed should be printed. You may count only as one try if the user inputs the same number consecutively. Note that for this question, you need to use a combination of loops and conditional statements.