Reference no: EM132387927
Question 1
The following program compiles and runs. What is the output of this program?
public class MysteryFigure
{
private int length; private int height;
public MysteryFigure ()
{
length = 4;
height = 3;
}
public MysteryFigure ( int num1, int num2 )
{
length = num1; height = num2;
}
public int getHeight()
{
return height;
}
public double getArea()
{
return (height * length);
}
public boolean isRectangle()
{
return (height != length);
}
public static void main( String[] args )
{
MysteryFigure fig = new MysteryFigure(); System.out.println("Height is: " + fig.getHeight()); System.out.println("Area is: " + fig.getArea()); System.out.println("Is it a Rectangle? " + fig.isRectangle()); MysteryFigure fig1 = new MysteryFigure(2, 4); System.out.println("Height is: " + fig1.getHeight()); System.out.println("Area is: " + fig1.getArea());
}
}
Question 2
The following code defines class Account, with a constructor that specifies the Account name and balance, and a toString method that returns a String containing the Account name and balance.
public class Account
{
private String accountName; private double accountBalance;
public Account(String name, double balance)
{
accountName = name; accountBalance = balance;
}
public String toString()
{
return String.format("%s balance is %.2f", accountName, accountBalance);
}
}
Your tasks are to:
• define two set methods to set the account name and balance.
• define two get methods to retrieve the account name and balance.
• create an Account object with the name "CQU" and the balance 812.85, and display a String containing the value of the object's instance variables.
Write java code for the above mentioned tasks.
Question 3
Based on the following Java programs, what will be the output when the EmployeeEarningSystem is executed?
public abstract class Employee
{
private String firstName; private String lastName;
public Employee( String first, String last)
{
firstName = first; lastName = last;
System.out.println(firstName +" "+lastName);
}
public abstract double earnings();
}
public class HourlyEmployee extends Employee
{
private double wage; private double hours;
public HourlyEmployee( String first, String last, double hWg, double hWk )
{
super( first, last); wage = hWg; hours =hWk;
}
public double earnings()
{
System.out.println( "This method calculates earnings based on hours" ); if ( hours <= 40 )
return wage *hours; else
return 100 * wage + (hours - 40 ) * wage;
}
}
public class EmployeeEarningSystem
{
public static void main( String[] args )
{
Employee currentEmployee = new HourlyEmployee("Kim", "Su", 5, 41 ); System.out.println( "Welcome to Employee Earnings Calculator\n" ); System.out.printf("Yr earnings are $%,.2f\n",currentEmployee.earnings() );
}
}
Question 4
What will be the output when the following program is executed? Show all GUI components including frames, menus and message dialog boxes.
import javax.swing.*; import java.awt.*; import java.awt.event.*;
public class Mystery extends JFrame implements ActionListener
{
public Mystery()
{
super("Welcome to Mystery Program"); setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JMenuBar menuBar = new JMenuBar(); setJMenuBar(menuBar);
JMenu fileMenu = new JMenu("File"); menuBar.add(fileMenu);
JMenu toolsMenu = new JMenu("Tools"); menuBar.add(toolsMenu);
JMenuItem openMenuItem = new JMenuItem("Open File"); openMenuItem.setActionCommand("Open File"); openMenuItem.addActionListener(this);
JMenuItem saveMenuItem = new JMenuItem("Save File"); saveMenuItem.setActionCommand("Save File"); saveMenuItem.addActionListener(this);
fileMenu.add(openMenuItem); fileMenu.add(saveMenuItem); setVisible(true);
}
public void actionPerformed(ActionEvent e)
{
String clickedString = e.getActionCommand(); if (clickedString.compareTo ("Open File") == 0)
{
JOptionPane.showMessageDialog(null, "Open an existing file.");
}
if (clickedString.compareTo ("Save File") == 0)
{
JOptionPane.showMessageDialog(null, "Save data to an existing file.");
}
}
public static void main(String[] args)
{
Mystery myProg= new Mystery();
}
}
Question 5
The following program is based on linked list operations. What will be the output when this program is executed?
import java.util.List; import java.util.LinkedList;
import java.util.ListIterator;
public class LinkedListTest
{
public static void main(String[] args)
{
LinkedList<String> myList = new LinkedList<String>(); ListIterator<String> iter;
myList.add("Cairns"); myList.addLast("Townsville"); System.out.println("List: "+myList); myList.addLast("Rockhampton"); myList.addLast("Gympie"); System.out.println("List: "+myList); iter = myList.listIterator(); iter.next(); iter.next(); iter.add("Brisbane"); iter.add("Sydney"); System.out.println("List: "+myList); iter.next();
while (iter.hasNext()) System.out.println("List: "+ iter.next()); iter.add("Melbourne");
System.out.println("List: "+myList);
}
}
Question 6
(a) By using the definition of Big-O, show that the running time of term T(n)=120+4n+ n2 is O(n2). Write your answer in no more than four lines.
(b) Assume that each of the following operators =, <, &&, [ ], ==, ++ counts as an operation. What will be the worst-case running time in terms of total number of operations for the following code?
for (int i=0; i<N; i++) {
if ( (i<N) && (data[i] == 5) ) keyFound = 1;
}
Question 7
Suppose there is a text file (publication.txt) with data format (publication name, publication type, ranking) as below:
Publication name Type Ranking
Neural-networks journal 1
International-conference-pattern-recognition conference 3
Deep-learning journal 2
The following program contains the partial code. Complete the program code so that it can read the data file (publication.txt) and display the following information on the screen.
• publications with ranking 1.
• total number of journal publications.
• total number of conference publications. import java.io.*;
import java.util.*; public class FileTest
{
public static void main(String[] args) throws java.io.IOException
{
int countJournal=0, countConference=0; try
{
File pubs=new File("publication.txt"); Scanner inp=new Scanner(pubs); while (inp.hasNext())
{
String pubName = inp.next(); String pubType = inp.next(); int pubRank = inp.nextInt();
//Display publication (name, type, ranking) if ranking is 1
/*Student to COMPLETE*/
//Count journal publications and conference publications
/*Student to COMPLETE*/
}
//Display total number of journals and total number of conferences
/*Student to COMPLETE*/
//Close the file
/*Student to COMPLETE*/
}
catch (FileNotFoundException fileNotFoundException)
{
/*Student to COMPLETE*/
}
}
}
Question 8
What is the output from the following sequence of queue operations? Assume that the PriorityQueue class here is a class that has implemented standard Queue operations offer, peek and poll.
PriorityQueue<Integer> q = new PriorityQueue<Integer>(); int num1 = 100, num2 = 120;
q.offer(num1); q.offer(num2); q.poll();
System.out.println(q.peek()); q.poll();
q.offer(50); System.out.println(q.poll()); q.offer(num2); q.offer(num1); q.offer(num2); System.out.println(q.peek());
while (!q.isEmpty()) System.out.print(q.poll() + " ");
Question 9
Provide a declaration and implementation of the generic method findMaximum() that takes an array of generic type T as the argument and returns the maximum value of the array.
Write a demonstration program to verify this method with the following arrays.
Integer [] integerArray={5, 30, 12, 5};
Double [] doubleArray={5.5, 4.5, 4.0, 10.5};
String[] stringArray = {"cat", "dog", "horse", "fox"};
Question 10
What does the following program do? Justify your answer (write maximum 3 lines). What will be the output of this program?
public class Recursion
{
public static int guessProg( int num)
{
if (( num==0) || (num==1)) return num;
else
return guessProg(num-1) + guessProg(num-2);
}
public static void main(String[] args)
{
System.out.println(guessProg (3));
System.out.println(guessProg (4));
}
}
Question 11
What are the values of A and B in the tree shown below so that it is a binary search tree? List the values of A and B. Manually provide the inorder, preorder and postorder traversals of the search tree shown below.
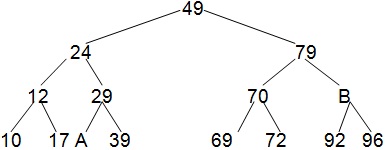
Attachment:- Term 2 Exam.rar