Reference no: EM132371374
Assignment - Paired Software Development
Overview
The aim of this assignment is to provide you, as students with the opportunity to apply knowledge and skills related to current good design principles and practices. You will follow an Agile system development approach by working in pairs in an iterative and incremental manner to write code and conduct unit and integration tests. Whilst coding you will apply SOLID design principles, design patterns and refactoring to satisfy the functional requirements of a portion of an animal clinic system.
Learning Outcomes Assessed
The following course learning outcomes are assessed by completing this assessment:
• S1. Apply software engineering principles to design and implement software applications.
• S2. Operate CASE software to develop appropriate models of software systems.
• S3. Develop comprehensive unit test suites.
• A1. Write integrated reports, using appropriate models, providing detailed analysis of given textual scenarios.
• A2. Implement software applications, using appropriate software engineering techniques, from a given textual scenario.
Assessment Details
Background
Agile System Development/Approach
The notion of Agile System Development is a counter-cultural movement that addresses change from a radically different perspective to those of the traditional software development world which is characterized by the engineering and process improvement advocates (Boehm and Turner, 2003). Agile Development has been introduced to satisfy the notion of change in the Digital/Information Age and will flourish because it helps businesses to deal with relentless change and speed and because it helps create the workforce culture of the future (Highsmith, 2002).
As the Agile Manifesto (Beck et.al., 2001) notes, Agile Software development values:
• Individuals and interactions over processes and tools;
• Working software over comprehensive documentation;
• Customer collaboration over contract negotiation; and
• Responding to change over following a plan
Adopting an Agile Development approach means there is an emphasis on flexibility and rapid response so that new and changing requirements can be anticipated during development (Satzinger, Jackson and Bird, 2016). This approach includes the notion of time-boxed iterative and incremental development (Larman, 2004).
A number of lightweight methodologies have been described that try to honour the values and principles of the Agile Manifesto. One of these is the adaptive, Agile development methodology of Extreme Programming (XP). Amongst its practices are Pair Programming, Test First Programming and Continuous Integration. You need to understand this background as you attempt this assignment.
Good Design
Your lecture slides for week eight state that when you achieve good design, your systems:
• are easily understood;
• are simple to modify and extend; and
• can be reused
When you don't have good design you may have smells in the categories identified by Martin (2003) - Rigidity,
Fragility, Immobility, Viscosity, Needless Complexity, Needless Repetition or Opacity. You may have specific Object Oriented (OO) smells as identified by Fowler (2000) or more generic and updated versions of these identified in his second edition (Fowler 2019). You should think about refactoring those. All systems should follow good design principles before any code is written, during development and after code has been "completed".
There is a strong interrelationship between what has been discovered by others and applying that knowledge to the work you do. Whether it is the more generic principles identified by the Guide to the Software Engineering Body of Knowledge (SWEBOK) (Borque and Fairley, 2014) - Abstraction, Coupling and Cohesion, Decomposition and Modularization, Encapsulation and Information Hiding, Separation of Interface and Implementation, Sufficiency, Completeness and Primitiveness, and Separation of Concerns - the five principles of OO class design1 (SOLID) and the additional package cohesion and package coupling principles identified by Martin (2003), these and more summarized by Beedle (n.d.), the nine OO Principles of Freeman and others (2008) or the Design Patterns2 identified for OO systems by Johnson and others (1995) and advanced since then into all aspects of pattern thinking e.g. Architecture, Cloud, Data and Enterprise (see for example, Alur, Crupi and Malks (2003); Buschmann, Henney and Schmidt (2007); Fowler (n.d., 2003); Hammer (2013); Hohpe and Woolf (2004); Homer et. al. (2014); Microsoft (2009); Portland Pattern Repository (n.d.); Teale et. al. (2003); Trowbridge et. al. (2003)), to become an expert software engineer you need to be able to synthesise and be proficient at appropriately applying this knowledge. You need to be able to have some modeling language e.g. UML for communicating with both IT literate and illiterate stakeholders. You also need to understand other aspects of the tools that you are using, for example paradigms in or computational models of programming languages3. When you are concentrating on a particular perspective e.g. in this case you are concentrating on an OO perspective, you need to be able to see the system at various levels and understand the potential crossovers between for example design patterns and refactoring (see Fowler (2019) and Kerievsky (2005)).You are not expected to know all this for this assignment; you are though expected to be aware of where what you are doing might sit.
So as you are still beginning your journey, what follows is a guided path through some of the previous material. You will apply some aspects of Agile Development and good OO Analysis, Design and Implementation to a portion of the Federation Animal Clinic system. You need to follow the steps to write code, test it, refactor it into the desired patterns in an iterative and incremental manner and to report on your activities.
Federation Animal Clinic System
The Federation Animal Clinic system is being built to provide practice management services for veterinarians and staff at the Federation Animal Clinic. The system will allow appointments to be made by pet owners with staff at the clinic. Specifically, the system will allow staff to create details of animals, owners and staff, to make, view, change and cancel appointments and to charge and deposit funds for services. You have been provided with code (in GitHub) that displays the front screen of the system. Included with the code are some text files that provide the persistent data repository for the system for the functionality that you will be addressing. The image below displays that front screen.
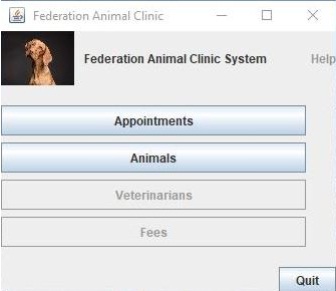
You have also been provided with the screen for the Appointments sub-system. This screen displays the sub- system functions - Make Appointment, View Appointments, Cancel Appointment and Transfer Appointments. A screen shot of that sub-system screen appears below:
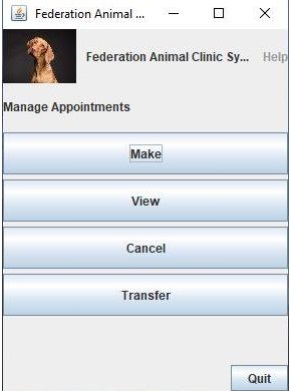
For this assignment you must initially write the code for the Make Appointment screen. You must then re-design the code to match stipulated design patterns.
You have the following user story for the Make Appointment screen. "As a receptionist I want to be able to make appointments for customer animals so that vets and other staff know what their calendar looks like for the next working week".
After some discussion with the staff member further details were obtained and these are included in the iteration table (later in this document) in the notes column of the first iteration.
In each of the tutorials from now until the end of the semester you will be asked to complete some of the requirements outlined below. Completing the tutorials will help you complete the assignment.
Assessable Tasks/Requirements
You are to complete the steps outlined below and submit a report and associated code solution. You need to use a number of software tools in this assignment. Access and documentation links to these tools are included in your Moodle shell. You are expected to use good design and coding principles and practices learned in lectures. Your design should be free from smells as much as possible. You should implement the required design patterns and practise refactoring. You are expected to follow pair programming practices. You need to use JUnit for your unit, regression and integration testing. You are expected to test thoroughly for exception handling, positive results, negative results, boundary conditions etc.and as a team provide evidence of that testing.
1. Step 1 - Identify who you will be working with and form your Pair.
2. Step 2 - Access the code
a) Complete the following to steps if necessary (you should already be familiar with these from Assignment
1) to setup your GitHub repository and download your starting code:
a. Enter the GitHub classroom to create your individual repository in GitHub;
b. If you haven't used GitHub before you will need to create an account using the link on the sign- in screen. Create a GitHub account by following the instructions in the handout (Registering for and using a GitHub repository and the GitHub Classroom) available in the Study Resources section of your Moodle shell.
c. Follow the separate handout (Using Eclipse IDE to interface with GitHub repository v2) available in the Study Resources section of your Moodle shell. Start at page 7 to page 11 to download and import the provided starter code from the GitHub classroom and load it into a new local repository in Eclipse.
d. Now you have imported the starter code in a project repository in Eclipse.
b) Run the system. You should see the main screen of the Federation Animal Clinic.
3. Step 3 - Begin Group Report
Open a new document in Word (or equivalent), This document will be your group submission. It will contain the pair response to each Refactor/Re-Design step of the assignment i.e. Refactoring/Design Name, Description, Steps taken, Design principles followed, Class Diagram, Test Information
4. Step 4 - Begin Individual Report
Open a new document in Word (or equivalent), This document will be your individual submission. It will contain an individual response to each re-design step of the assignment i.e. What went well, What could have been improved, Review/Description of the work done in Iteration, Measure of individual contribution (%),
Measure of partner contribution (%) (These two measures should tally to 100%).
5. Step 5 - Iterate through the following tasks (until you've completed all tasks identified in the Iteration Tasks table below):
A. Understand requirements for task
Do you really understand what is required? Ask your lecturer for clarification about functional requirements. Consult the Satzinger, Jackson and Bird (2016) text for a general overview of OO analysis and design, UML and methodologies. Consult your lecture notes and sources such as Parsons (2012) and Sharan (2014) for Java; Freeman and others (2008), Hammer (2013), Holzner (2006), Johnson and others (1995), Metsker and Wake (2006), Martin (2003), Shalloway and Trott (2004) for principles and design patterns and Fowler (2000, 2019), Kerievsky (2005) and Suryanarayana, Samarthyam and Sharma (2015) for refactoring and the interrelationships with design patterns.
B. Iterate through (until satisfied):
i. Identify how you would change/refactor
Perhaps the most accessible books for this are Freeman and others (2008), Hammer (2013), Holzner (2006), Fowler (2000, 2019) and Kerievsky (2005).
ii. Identify and if necessary construct tests to perform
You need to be able to have some form of testing class/es using JUnit that allow you to test. Consult your lecture notes and tutorial seven for this. The books by Langr (2015) and Parsons (2012) would also be helpful.
iii. Test
Run the appropriate suite of tests to ensure the tests are valid/system is working and to understand what your baseline is.
iv. Code
Resolve to follow good design principles and practices as well as conventions.
v. Test
Run your suite of tests to ensure the system is still working and you have satisfied requirements.
vi. Integrate
As you complete your work, commit and push your new code from your local Eclipse repository to save it in your own online GitHub repository. It may be necessary to add files to the index before committing.
C. Update your Individual Report
D. Update your Group Report
6. Step 6 - Complete Group Report
7. Step 7 - Submit Group Work
One member needs to take responsibility for submitting the group's work.
8. Step 8 - Complete Individual Report
Tally the individual measures for each iteration, for each partner and provide a value out of 100% for each partner.
9. Step 9 - Submit Individual Report
Iteration Tasks
Note: In the requirements that follow, there is an expectation that you will implement the patterns in a pre- functional Java form i.e. you will not use lambda and functional programming4 extensions introduced from Java 8 onwards.
Iteration
|
Week
|
Task
|
Notes
|
1
|
8
|
Construct Make Appointments screen
|
You need to provide functionality on the one screen to select an Animal, select a Veterinarian, select a Room, select a Type of Appointment, specify one or more additional resources required for the appointment based on ResourceType (e.g. assistance -
e.g. anaesthetic nurse or handler; materials - e.g., bed, cage), key in the appointment date
and time, provide a button to SAVE the details (test on SAVE for a duplicate and display message or display message for successful save), provide a button to CANCEL the entry (clear fields) and provide a button to QUIT (returning to Appointments sub-system screen). Look at the code for the existing screens to help you with how you might create. Swing rather than JavaFX is expected to be used here. Look at Parsons (2012), Sharan (2014) and the API for further help.
|
2
|
9
|
Implement Singleton pattern for AppointmentBook class access to file
Implement MVC pattern for Make Appointments
|
You need to have only one access point and one AppointmentBook so that all appointments are synchronized. The AppointmentBook is read from and saved to a file. Look at Satzinger, Jackson and Bird (2016), pp. 431-433 and Holzner (2006), pp. 91-99 (We don't need to worry about multi- threading here).
For the MVC pattern follow the example in Freeman et. al (2008) pp. 528-544 (implementation of MVC via the Strategy, Composite and Observer patterns) and the discussion in Satzinger, Jackson and Bird (2016) pp. 428-429 and try to match to this
situation.
|
3
|
10
|
Implement Façade pattern for Appointments
|
Use a Façade class to hide the complexity of all the work that goes into managing Appointments i.e. The sub-system screen should use this Façade to Make, View, Cancel and Transfer appointments. Look at
Freeman et. al. (2008), pp. 254-270 or Holzner (2006), pp. 134-144
|
4
|
11
|
Implement Factory and Decorator patterns to deal with types of Appointments and allocation of
ResourceTypes
|
Change the Make Appointments code so that a simple Factory is used to create the correct type of appointment and use the Decorator pattern to handle the allocation of ResourceTypes to Appointments. Look at Freeman et. al. (2008), pp. 109-117 and 79-
105 or Holzner (2006), pp. 39-59
|
Attachment:- Paired Software Development.rar