Reference no: EM132401528
INFS1609 Fundamentals of Business Programming Assignment - UNSW Business School, University of New South Wales, Australia
Part 1 - Tutorials Marks
Implement a simple system to calculate a student's tutorial mark for each week. Your program should ask for: the student's name, tutorial, number of questions for that week, number of test cases passed, the number of test cases and weighting of each question. Your program should then print the score and the Moodle summary data.
You will need to design a TutorialMark class which contains the appropriate attributes to store all of the information. You must create the appropriate getter, setter and constructor methods. You may create additional classes if you feel that it is necessary for this task.
We will provide you with a TestTutorialMark class, this class is responsible for prompting the user for input and displaying the output as required below. Text written in red below in the samples indicate user input. Round to 1 decimal place when required.
You can assume that all values entered are valid.
Part 2 - Stationery Store
You've been asked by a stationery store to implement a basic database system. The relationship between the objects is dictated below:
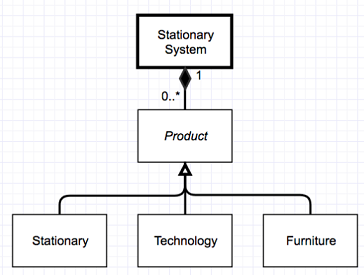
Each class has the following attributes:
- Product(int id, String name, int quantity)
- Stationery has in addition (String colour)
- Technology has in addition (int powerConsumption, int warrantyPeriod)
- Furniture has in addition (int weight, boolean warehouse)
The stationery shop is a bit quirky; the price of each item is calculated in the following way:
- Product = length of the product name * quantity
- Stationery = length of the colour name * quantity
- Technology = power consumption / warranty period
- Furniture = weight / quantity
Programming tasks -
Using your knowledge of OOP, Inheritance and Polymorphism design, implement the classes above.
You should submit 6 Java files: Stationery.java, Technology.java, Furniture.java, Product.java, StationerySystem.java and TestStationerySystem.java.
You will need to implement a StationarySystem class which is in charge of maintaining the objects, the StationarySystem constructor should take an int which specifies the number of different product types the StationarySystem can hold, and should provide the following publicly accessible methods described under the functionality table.
The price of items should be a double rounded to 2 decimal places where necessary.
All items are located at the store by default, only certain furniture items are located at the warehouse.
Design explanation -
You must submit a design.txt file containing your answer to the following question (100 word maximum): Explain how you have implemented printInventory() and if applicable, which aspect of OOP are you utilising to do so?
Attachment:- Business Programming Assignment File.rar