Reference no: EM133337489
Stage 1
The coursework involves implementing a simulation of a road intersection. This first stage develops a basic GUI-based Java application, which you will then extend in Stage 2. Stage 1 is designed to assess your understanding of planned iterative development and your knowledge of data structures, exception handling and unit testing, which are all taught in the first half of the course.
Functional requirements
We consider a signalised road intersection of an arbitrary number of road segments (Fig. 1). For experimental work in this coursework, we consider an intersection with only four segments.
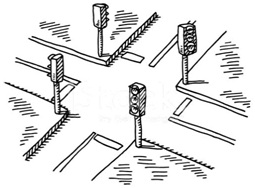
Figure 1. Typical signalised road intersection with four road segments
Traffic on road segments is controlled through a group of phases. Each phase is an interval of time assigned to the movement of traffic through a traffic signal. In this coursework, we consider only vehicles and no pedestrians. Figure 2 shows an example of traffic signal with 8 standard phases.
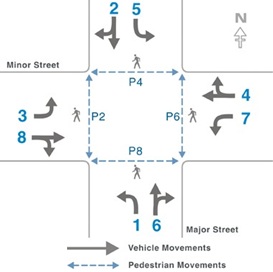
Figure 2. Eight (8) standard phases traffic signal (source 1)
Each phase has a fixed duration. The complete sequence of all phases is a cycle. The same cycle then gets repeated continuously.
You will not be writing the simulation code until Stage 2. For Stage 1, you are asked to create a GUI-based application that shows the initial state of the simulation, based on reading information from files and information added by the user. Specifically:
1. When started, the application reads the configuration of the intersection from a text file (intersection.csv): each line has a phase number and the phase duration in seconds.
2. Each vehicle has a specified segment where it begins, and has an identifier (ex. A plate number), a type (car, truck, bus), a crossing time in seconds that defines how long it takes it to cross the intersection, a direction (straight, right, left), intersection crossing status (crossed, not crossed), length in metres, and an emission (grams of CO2 per minute while waiting or moving). A typical emission rate is about 10 grams per minute for diesel vehicles and 5 grams per minute for petrol vehicles.
3. The application initially reads data from a file (vehicles.csv). The file represents a list of vehicles. The file is organised one vehicle per row, containing the above information.
4. While running, the application should allow the user to add vehicles through a simple GUI. The user defines the segment on which the vehicle arrives, an identifier, the type, crossing time, crossing status (initially false), length, and emission rate.
5. The GUI (Fig. 3) should display the list of vehicles with their corresponding segments and crossing status. In addition, the GUI displays the number of vehicles waiting to cross each segment, the total length of waiting vehicles within each segment, the average crossing time per segment, and the total emissions calculated for all vehicles waiting to cross the intersection.
6. The application exits when the user decides (by clicking on an exit button).
7. Before the application exits, it should generate a report with the total number of vehicles crossed per phase, the average waiting time to cross, and the total emissions. The report should be written to a text file.
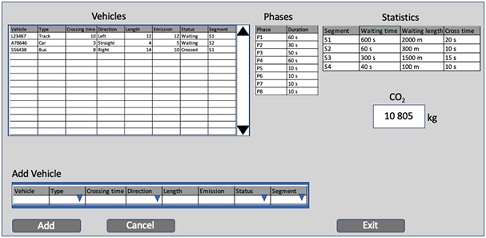
Figure 3. Sample GUI for stage 1 (colours are not required)
You can add extra functionalities to your GUI, such as sorting vehicles by segment, status, and type. The user could be given the possibility of changing the number of phases and/or their
durations. For example, instead of 8 phases, we can add pedestrian phases or use a standard 4 phases. The standard 4 phases control consists of having left turn done simultaneously in two phases and straight crossing done in two phases (Fig. 4). In stage 1, we expect you to implement only the GUI elements for the extra functionality about phases. The actual simulation of variant phases would be implemented in stage 2.
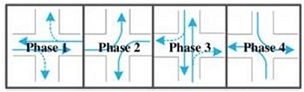
Figure 4. Four phases (source 2).
Non-functional requirements
These are the software engineering requirements for Stage 1:
1. Your application should be implemented using Java.
2. Develop your program using planned iterative development. In this stage you should do all the design before writing the code. Decide on all the classes for this stage, their instance attributes, and methods. Try using a design method and diagrams where appropriate. Make a plan to divide the work between you, in such a way each person can work independently where possible. How will you test your code? Decide when and how often you need to meet or be in contact. How will you integrate your work?
3. Base all your decisions just on the requirements for this stage. Do not use agile development at this stage, and do not plan ahead to Stage 2. However, do take notes about the development process and your experiences, since you will be asked to summarise this in your report.
4. Your program should read the data from the files at the start and store it into appropriate data structures. When reading the files, you do not need to think ahead to the GUI or reports; just store the data so that it can be accessed easily (e.g. is a list suitable? would a map be useful?). Then, write methods to analyse the data, which is likely to involve using more data structures. When making your decisions, imagine that you have many vehicles, on different segments, and with different directions.
5. Use version control. Your group should set up a repository, and a link to this repository should be included in your report. Note that we will check the commit history, so make sure this reflects your individual contributions.
6. Use exceptions to catch errors in the data. Each group should decide what makes valid data (e.g., length, range, number of characters, etc.) If an error is found, just continue without that line of data. Provide suitable data to check that your program is working correctly, e.g. input files with some errors.
7. You should throw exceptions in the constructor of at least one class, to ensure that the objects of that class that you create are valid (e.g. vehicle unique identifier), and you should write at least one of your own exception classes.
8. Use JUnit to test some of your constructors and/or methods, particularly ones involving calculations, e.g. the method that estimates the CO2 emission. You could try test-driven development for these methods. If you create a JUnit test for a method, you should test all the paths in the method, not just one.
Development Plan
One person in the group should submit this document, which gives details of your class diagram, chosen data structures and work plan, before you start coding. This should be done by Friday in Week 5. Submission is mandatory and your group will be penalised if it is not submitted on time. This document should be a maximum of 3 pages in length.
Group Report
The report will consist of several sections:
1. Names of group members and a summary (no more than one page) explaining who did which parts of the application and which parts of the report.
2. A link to your repository.
3. Does your program meet the specification, or are there some bits missing or bugs outstanding? Either provide a single sentence "This program meets the specification" or provide details of problems that you know about.
4. Suitable UML class diagram(s) showing the associations between the classes, and the contents of each class.
5. Explain which data structures you used, which classes they are used in, and why you chose them. (Some tools for creating UML diagrams hide the data structure of associated classes. Ensure the reader knows what these are.)
6. What decisions has your group made about the functionality of the program? (This is just information, not a technical report). Include the format of any identifiers and details about how length of waiting is calculating per segment.
7. Testing: What did you test using JUnit? Give details of which JUnit tests relate to which methods. (No need to print all the code, the marker will look at this electronically). Which types of exception did you use, in which method, and for what purpose?
Try to keep the writing concise. Your report should be no longer than 12 pages.
Stage 2
Stage 2 is designed to assess your ability to use thread-based programming and design patterns, and your understanding of agile development, which are all taught in the second half of the course. In this stage, your application will be extended to simulate vehicles moving through their segments and crossing the intersection over time. This will involve the addition of threads and design patterns, and the development of a suitable GUI to show the state of the simulation.
Core Functional Requirements
These are the core functional requirements for Stage 2. In this stage, you are expected to simulate moving vehicles from their entry point until they cross the intersection.
1. As for Stage 1, the application will read in details of the intersection and existing vehicles when it starts up. We consider that all added vehicles are in queues to cross the intersection (one queue per segment). Each added vehicle will be positioned at the end of the queue and therefore will be on a certain distance from the intersection3. You might need to keep track of the vehicle position as attribute or calculate it by summing the length of the preceding vehicles.
2. In addition to initial vehicles, an arrival process will randomly generate vehicles and allocate them to segments. You might also need to register any new vehicle with its preceding vehicle and with the central controller (see hereafter).
3. Each vehicle needs to communicate the distance it travelled to its following vehicle.
3 We consider that the segments have infinite length
4. The application continuously repeats the traffic control cycle. Each cycle consists of a sequence of phases. At each phase, vehicles cross to their destination. We consider that segments have lanes dedicated to turning left, so that traffic moving straight is not blocked. In a segment, after each phase, vehicles waiting to cross can only travel the distance left by the vehicles which crossed.
5. A central controller will update the traffic signal (green, orange, red) according to the phase durations.
6. After each change of the signal, vehicles are notified of the new status of the signal. On receiving the notification, the vehicles in the segment get ready to move and wait for the preceding vehicle to send them the distance they travelled.
7. In each phase and for each direction, we consider that only one vehicle enters the intersection at a time. When current vehicle crossing time is over, the next vehicle can enter the intersection. However, you can create other designs that you deem more appropriate.
8. The GUI is updated with changes that happen to the vehicles: whether they crossed and they are still waiting, their total waiting time.
9. The total emission, average waiting time, and total waiting length are then updated and displayed.
10. The simulation stops when the user pushes the exit button.
11. A log should be kept which records events as they happen, e.g. a vehicle crossed, a vehicle was added, a signal status has changed... This log should be written to a file when the application exits.
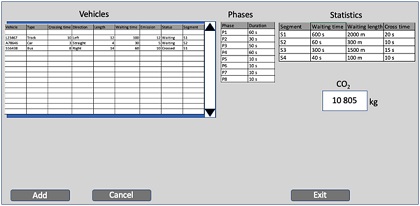
Figure 5. Example of GUI for stage 2 (layout and display format are to be adapted)
Software Engineering Requirements
These are the software engineering requirements for Stage 2:
1. You should experiment with agile methods. For your initial overall plan, simply decide how many iterations you will have, and which features will be developed in each iteration. Then plan each iteration when you start work on it. Plan, design,
develop and test each iteration without considering features in the future iterations. You may need to refactor your code at the end of each iteration, before continuing.
2. Spend some of the time trying out agile techniques such as pair programming, stand- up meetings and time-boxing, and decide whether they are practical for you to use in your project or not. Note down your observations.
3. Use threads and ensure that they are synchronized where necessary and do not interfere with one another (see the learning materials in Part 2 of the course). For the core functional requirements, you should have at least one thread for each vehicle, and one thread for the signal controller. The intersection can be designed as one buffer (for each direction) that can hold a single vehicle during its crossing time. When the buffer is empty, the next vehicle can enter. Your simulation must coordinate vehicle-threads when accessing the intersection (which is a shared resource). You are encouraged to add more threads to the application when you develop extensions and consider more advanced scenarios like the producer-consumer model.
4. Java provides thread-safe versions of some of its collection classes. However, you should not use these since this will limit your ability to demonstrate your knowledge of threading.
5. Your design should include design patterns. As a minimum, you should use the Singleton pattern to implement your log class, and the observer and MVC patterns in your GUI (see the learning materials in Part 2 of the course). You are free to use other design patterns.
6. Use packages appropriately.
7. Continue to use version control.
8. The final application should be exported to a JAR file, from which the program can be run. Resource files (e.g. text files) should be included in the jar file. See the lecture on JAR files for information about how to do this.
Extended Functional Requirements
You are also asked to extend the core requirements. Marks will be awarded based on the complexity of your extension(s) and the knowledge and understanding required to implement them. The following are some suggestions. You are free to come up with your own, though you may want to check with your lecturer before starting work on them:
1. The application can simulate the traffic on variant phase policies (example 4 phases as described in stage 1 above).
2. The application can implement an adaptive control policy by estimating the phase durations depending on the waiting time at each segment in order to reduce the overall waiting time and length (therefore reducing emissions).
3. The application could allow creating a chain of two or more intersections. In this case the traffic coming out from one intersection feeds to the next intersection through the shared segment.
4. With two or more intersections connected, the application could implement a light- wave traffic control scheme. This consists of allowing vehicles that cross a green light at an intersection to cross with minimal waiting time (or none) the next light as long as it runs at a standard speed (e.g. 60 km/h).
Group Report
The group report for stage 2 should include the following:
1. A brief description of the functionality that your system provides and what it does not do, i.e. does it meet the specification, are there some bits missing or bugs outstanding. How did you extend the core requirements?
2. UML class diagram(s) showing the associations between the classes, and the contents of each class - possibly not both in the one diagram.
3. Details about how you developed your program using agile processes. What features did you include in each iteration? Which agile techniques did your group use?
4. An explanation of how and where threads are used in your application.
5. An explanation of how and where design patterns are used in your application.
6. Sample screen shots.
7. A brief comparison of your development experiences in Stage 1 and Stage 2. Did plan-driven or agile development work better for your group? What problems did you encounter, and what might you do differently next time?
The technical sections of your report should be written for someone who has studied the material on this course but is not familiar with the coursework. You should include diagrams (including UML diagrams where appropriate) and snippets of code where relevant. Try to keep the writing concise. Your report should be no longer than 15 pages.
Attachment:- road intersection.rar