Reference no: EM132223910
Introduction to Programming in C Assignment -
Objectives - To design, implement and document more complex modular programs that use functions, loops, arrays and pointers.
Do all exercises and submit a single Word file. Submissions must be a single Word file containing the work done by the student (student name and student ID should be clearly stated in the first page). The file must contain two parts for each exercise proposed in this assignment as detailed below.
Part I: The first part must contain the source code. Please use font Courier New with a size of 8 points, use indentation to make the code readable, and observe the following requirements:
- The source code must be the result of your own original and individual work.
- The source code must be entirely written in the C programming language. Source code written in any other programming languages (e.g., C++) will receive a mark of zero.
- The use of global variables is (in general) not needed in ELEC129 assignments and its use is not allowed unless otherwise stated. All variables should be local to a function (i.e., declared within the body of a function). The use of global variables will be penalised. If you are in doubt, ask a lab demonstrator to check your source code.
- All exercises can be solved based on concepts explained in previous lectures. Students are allowed to use concepts from other (future) chapters but this is not expected. Feel free to discuss your approach to solving the exercises with a lab demonstrator.
Part II: The second part must contain a detailed explanation of the software development process followed by the student (i.e., the first five steps of the software development method):
1. Problem specification: Formulation of the problem and its objectives.
2. Analysis: Identification of: i) inputs, ii) outputs, and iii) other relevant aspects, requirements or constraints (e.g., relevant formulas, etc.).
3. Design: Formulation of the algorithm (list of steps) needed to solve the problem. At this stage, the problem should be divided into a number of sub-problems that can be solved using functions.
4. Implementation: List of functions used in the program (function names), indicating for each function which step(s) of the algorithm is/are implemented by the function.
5. Testing and verification: Explanation of how the program was tested and verified. Snapshots of the program's output window can be obtained by using [Alt] + [PrtScr(or PrintScreen)] and pasting into the report.
Exercise -
The objective of this assignment is to implement the tic-tac-toe game with a C program.
The game is played by two players on a board defined as a 5 X 5 grid (array). Each board position can contain one of two possible markers, either 'X' or 'O'. The first player plays with 'X' while the second player plays with 'O'. Players place their markers in an empty position of the board in turns. The objective is to place 5 consecutive markers of the same type in a line (a line can be any row, any column or any diagonal). The first player who manages to place 5 markers in a line wins. The game is played until one of the players wins or until the board is full with no player having 5 markers in a line (i.e., the result of the game is a draw).
Your program should do the following:
1) Ask at the beginning if the game is going to be played by two human players (i.e., human player vs. human player) or by one human player and the computer (i.e., human player vs. computer). In the latter case (i.e., human player vs. computer) there must be an option that allows the player to choose the first to play (either the computer or himself/herself).
2) Players will play in turns (i.e., the first player will place an 'X' on the board, then the second player will place an 'O' in the next move, and so on).
a. When it is a human player's turn, the selected board position must be introduced by the user by indicating the corresponding row and column numbers. Your program should display numbers around the board, as shown in the example below, in order to assist the player:
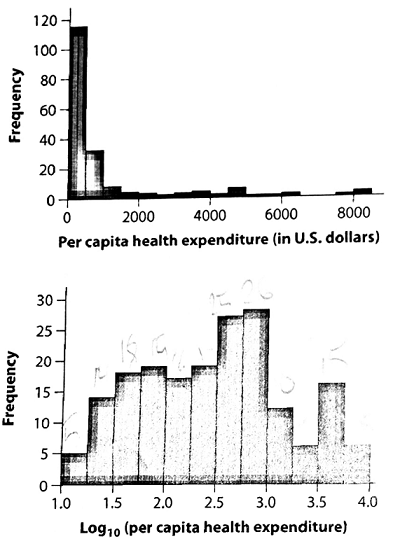
b. When it is the computer's turn (in human vs. computer mode), the computer should choose a valid empty position. A possible tactic to choose the position is suggested in point 6 (see below).
3) If a human player choses an illegal position (out of bounds or already taken) then a message should be displayed. The program should keep asking the player to introduce the position for his/her move until a valid position is chosen by the player.
4) After each move, the entire board containing all previous moves should be reprinted. The following is an example of how the board could appear on the screen:
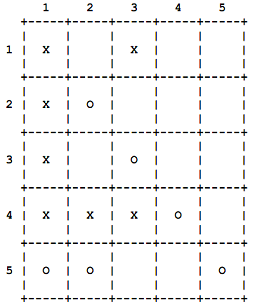
5) When there are 5 consecutive markers (either 'X' or 'O') in line anywhere in the board (i.e., any row, column or diagonal) the game should finish and the program should display a message indicating who has won the game. The game should also finish when the board is full with no player having 5 markers in a line; in this case the program should display a message indicating that the final result of the game is a draw.
6) When the user chooses to play in human vs. computer mode, the following computer tactic may be employed in every computer's turn:
a. The computer first checks all possible valid moves and if there is any move that allows the computer to win immediately, that move is selected.
b. If (a) is not possible, then the computer checks if the human player is about to win in the next move. If this is the case, then the computer blocks that move.
c. If cases (a) and (b) are not relevant, then the computer can choose a random empty position. This can be done with the rand() function, which is declared in the C standard header file stdlib.h. The following statements:
int x = rand() % 5;
int y = rand() % 5;
store in x and y random integer numbers between 0 and 4. This can be used to generate the row and column numbers until an empty position is found.
Attachment:- Assignment Files.rar