Reference no: EM131417006
Use Java to implement the Dynamic Linkage pattern specified below.
You need to implement all of the classes as defined below substituting the yellow classes with your own class definitions.
For example, you need to define your own FoodProcessorProgram, such as Salsa and PotatoSalad in place of YourRecipeA and YourRecipeB.
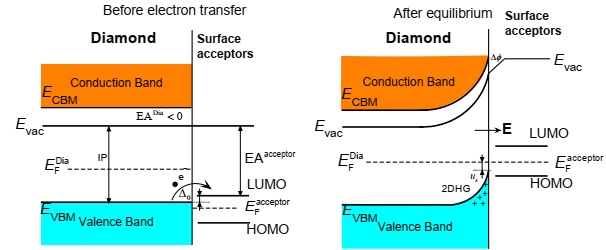
FoodProcessorEnvironmentIF and AbstractFoodProcessorProgram are the same as specified in the book.
AbstractFoodProcessorEnvironment and ChocolateMilk are provided below.
Your Client/main program needs to
• ask user to pick a directory to find FoodProcessorProgram (Recipe) which will be the classPath
Ex. User selects ./bin as the class path directory, your program creates a File object with "./bin" as parameter, then you call toURI() then call toURL() to get the URL representation of the user selected directory. The selected URL representation of classPath will be used as a parameter to create user selected environment.
You can use either JFileChooser to let user select a directory or use command prompt.
• ask user to pick one of your FoodProcessorEnvironments to use
Ex. User selects YourFoodProcessorEnvironmentA, your program creates an instance of YourFoodProcessorEnvironmentA. Or you can create both instances at the beginning then assign the selected on to the current environment.
• ask user to pick one of FoodProcessorPrograms(Recipes) to use
Your program needs to show all the .class files under the user selected directory (classPath) and allows user to select one of the .class files as the program to be run
(started). Ex. User selects ChocolateMilk which is a String that will be the parameter passed to run() on user selected environment.
• call run() on the user selected environment with user selected
FoodProcessorProgram (Recipe) as parameter.
Class AbstractFoodProcessorEnvironment -
import java.net.URL;
import java.net.URLClassLoader;
/**
* class AbstractFoodProcessorEnvironment
* implements FoodProceesorEnvironmentIF which contains methods called by Food processor programs
* defines instance variable URL[] classPath which contains the classPaths
* that will be searched to load Food processor programs
* defines void run(String programName) which will be called by an initialization program
* which wants to use an instance of this environment to find the named programName in classPath
* using URLClassLoader to load a class of programName then creates a new instance of program
*/
public abstract class AbstractFoodProcessorEnvironment implements FoodProcessorEnvironmentIF { private URL[] classPath;
public AbstractFoodProcessorEnvironment(URL cp){
classPath = new URL[]{cp};
}
/**
* Run the named program.
* @param programName the name of the program to run.
*
* Creates an URLClassLoader based on the specified classPath
* the class loader loads the class based on the programName
* the program class then creates a new instance of the program
*
* Sets this environment to be the newly created program instance's environment,
*
* Calls start() on the program
*/
abstract void run(String programName); void display(String s) {
System.out.println("Displaying the program name - "+s);
} // display(String)
URL[] getURL(){
return classPath;
}
} // class AbstractFoodProcessorEnvironment
Class ChocolateMilk -
public class ChocolateMilk
extends AbstractFoodProcessorProgram {
/**
* Return the name of this food processing program object.
*/
public String getName() { return "ChocolateMilk"; }
/**
* A call to this method tells a food processing program to start
* doing whatever it is supposed to be doing.
*/
public void start() { double milkWeight; double cocoWeight;
System.out.println("\n--------- Starting Recipe ---------");
System.out.println("Place milk on the scale ..."); milkWeight = getEnvironment().weigh(); System.out.println("Milk weight is "+milkWeight);
System.out.println("Remove milk from scale and place it in the mixer ...");
System.out.println("Place Coco powder on the scale ..."); cocoWeight = getEnvironment().weigh(); System.out.println("Coco weight is "+cocoWeight);
System.out.println("Remove Coco powder from scale and place it in the mixer ..."); getEnvironment().mix(4);
System.out.println("--------- End Recipe ---------\n");
} // start()
//...
} // class ChocolateMilk - a ConcreteFoodProcessorProgram
A sample output below with user selected directory as ./bin and ChocolateMilk as selected FoodProcessorProgram.
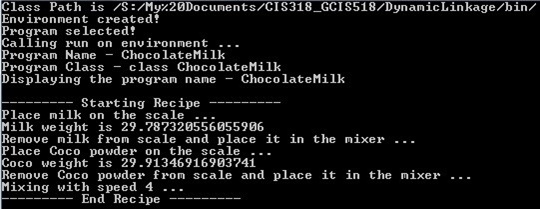
You need to include a header section for each of your Java program.
The header basically is a comment section at the beginning of each of your Java file about the name of file, what the file is about, the creator, when was the file created, last modified for what reasons.