Reference no: EM131056103
Pass Task 5.1: Hand Execution of Arrays
Overview
Arrays are key to understanding how the computer can work with large amounts of data. When you understand how arrays work you will be ready to start making more complex programs.
Purpose: Learn about how arrays work in the computer.
Task: Hand execute the provided code snippets to demonstrate key aspects of working with arrays.
Instructions
Arrays are incredibly useful, and really important to understand. Hand execution can really help you understand these more fully.
To represent an array draw a box divided into sections for each value. This represent the array's location in memory, providing you with access to the array as a whole and to the individual elements.
The following is an example of how to represent an array in your Hand Execution.
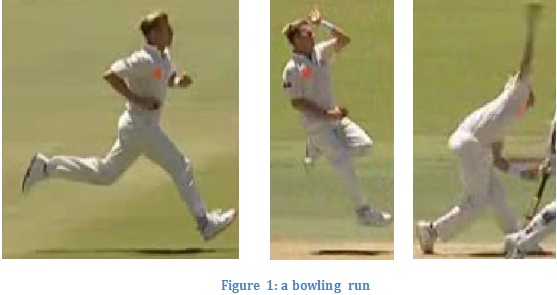
Demonstrate how the following code is executed in the computer.
function ???(const data: array of Integer): Integer;
var
i: Integer;
begin
result := 0;
for i := Low(data) to High(data) do begin
result := result + data[ i ];
end; end;
Hand Execute the program with the following parameter values to see if you can work out what it does.
data
|
Result
|
[6, -2, 3, 8, 1]
|
|
[2, 6, -1, 3]
|
|
Provide a name for this on your hand execution images.
The following code performs a useful tasks with an array of Integer values. What does it do?
function ???(const data: array of Integer;
val: Integer): Boolean;
var
i: Integer;
begin
result := False;
for i := Low(data) to High(data) do begin
if data[i] = val then begin
result := True;
exit; // end the function
end;
end; end;
Hand execute the function with the following parameter values.
data
|
val
|
Result
|
[2, 6, -3, 3, 7]
|
3
|
|
[-1, 8, 2, -4, 9]
|
6
|
|
Provide a suitable name for this function on your hand execution image you submit.
Questions
List the actions the computer executes when it runs the following code.
for i := 0 to 3 do begin
WriteLn('i is ', i);
end;
List the actions the computer executes when it runs the following code.
for i := 3 downto 0 do begin
WriteLn('i is ', i);
end;
Assume that names is an array of three names. Names array:
List the actions the computer executes when it runs the following code.
WriteLn('Array of ', Length(names), ' values'); for i := 0 to High(names) do
begin
WriteLn(names[i]);
end;
Assume that a Score record contains a name and a score, and that the scores variable is an array of three Score record values.
Scores array:
Index
|
Value
|
0
|
Name: Fred
Score: 10
|
1
|
Name: Wilma
Score: 20
|
2
|
Name: Betty
Score: 15
|
Assume you also have the following procedure:
procedure WriteScore(const toWrite: Score); begin
WriteLn(toWrite.name, ' scored ', toWrite.score);
end;
What will be output when the computer runs the following code:
WriteLn( 'Message 0' ); WriteLn( scores[1].Name ); WriteLn();
WriteLn( 'Message 1' );
for i := 0 to High(scores) do begin
WriteLn( scores[i].Name );
end;
WriteLn();
WriteLn( 'Message 2' );
for i := 0 to High(scores) do begin
WriteScore(scores[i]);
end;a
Hydrogen ion concentration in the solution
: A 25cm^3 sample of dilute sulfuric acid contains 0.025 moles of acid. What is the hydrogen ion concentration in the solution? Please explain working.
|
Biggest challenges facing organizations
: In this assignment, you will create a PowerPoint presentation that outlines what you believe will be the biggest challenges facing organizations in the next twenty (20) years.
|
Question regarding the transitions between terms
: Using the selection rules deltaS=0, deltaL=+-1 and deltaJ=0,+-1, predict which transitions are allowed from the ground level in A). Consider only transitions between terms within each configuration given.
|
What is relationship between a logical and a physical model
: Explain the use of a logical model. Provide a definition of a physical model. Explain the use of a physical model. List and discuss two approaches used for the development of logical and physical models.
|
How the computer can work with large amounts of data
: Arrays are key to understanding how the computer can work with large amounts of data. When you understand how arrays work you will be ready to start making more complex programs.
|
Discuss at least two monetary policy tool that you could use
: Discuss at least two monetary policy tools that you COULD use, the advantages and disadvantages of each, and the effects each has on GDP, exchange rates, interest rates, money supply, stock market and the bond market.
|
Internet to research the code of ethical conduct
: According to the textbook, ongoing challenges in the global business environment are mostly attributed to unethical business practices, failure to embrace technology advancements, and stiff competition among businesses. Use the Internet to researc..
|
How the evolutions of healthcare delivery in us positively
: Describe how the evolutions of healthcare delivery in the United States positively or negatively affect the current health care system. Provide examples with your response.
|
Temperature of the water inside the calorimeter
: A 12.0 g ice cube, at 0.00°C is placed inside a calorimeter (the heat capacity of which is negligible) containing 145 g of water at 20.8°C. Once the ice cube has melted what is the temperature of the water inside the calorimeter?
|