Reference no: EM131065530
Problem 1
a) Draw the synthesized logic resulting from the following VHDL code. Label all the signals on your diagram precisely.
entity unknown is generic (k : natural := 3);
port (x : in std_logic_vector ( 2**k-1 downto 0); f : out std_logic);
end unknown;
architecture struct of unknown is component and2 is
port (a, b : in std_logic; c: out std_logic);
end component;
component or2 is
port (a, b : in std_logic; c: out std_logic);
end component;
type matrix is array (k-1 downto 0, 2**k-1 downto 0) of std_logic;
signal temp : matrix;
begin
outer_loop: for j in k-1 downto 0 generate
inner_loop: for i in 0 to 2**j-1 generate
and_gen: if j = k-1 then generate
and_gate: and2 port map (X(2*i), X(2*i + 1), temp(j,i));
end generate;
or_gen: if j < k-1 then generate
or_gate: or2 port map (temp(j+1, 2*i), temp(j+1, 2*i + 1), temp(j,i));
end generate; end generate;
end generate; f <= temp(0,0); end struct;
What is the critical path delay for this circuit based on your diagram in a general case? State your answer in terms of variables used in the design assuming the delay of AND and OR gates are TAND and TOR respectively.
Problem 2
Write a function that creates a wide AND gate that operates on a vector of any length.
function wide_AND(a: STD_LOGIC_VECTOR) return std_logic is variable retVal: STD_LOGIC;
begin
retVal := '1';
for i in a'range loop
retVal := retVal and a(i); end loop;
return retVal;
end;
Problem 3
retVal := '1';
for i in a'range loop
retVal := retVal and a(i); end loop;
return retVal;
Draw the state diagram corresponding to the following FSM VHDL model:
library IEEE;
use IEEE.std_logic_1164.all; use IEEE.std_logic_arith.all;
use IEEE.std_logic_unsigned.all;
entity FSM_MIDTERM is port (
CLOCK: in STD_LOGIC; INPUT: in STD_LOGIC; RESET: in STD_LOGIC;
OUTPUT: out STD_LOGIC_VECTOR (2 downto 0));
end;
architecture FSM_MIDTERM_OPERATION of FSM_MIDTERM is type Sreg0_type is (S1, S2, S3, S4);
signal Sreg0: Sreg0_type;
begin
Sreg0_machine: process (CLOCK, reset) begin
if RESET='1' then Sreg0 <= S1; OUTPUT <= "000";
elsif CLOCK'event and CLOCK = '1' then case Sreg0 is
when S1=>
OUTPUT <= "000";
if INPUT='1' then
Sreg0 <= S2; elsif INPUT='0' then
Sreg0 <= S1;
end if;
when S2=>
OUTPUT <= "010";
if INPUT='1' then
Sreg0 <= S4; elsif INPUT='0' then
Sreg0 <= S3;
end if;
when S3=>
OUTPUT <= "100";
if INPUT='0' then
Sreg0 <= S1;
elsif INPUT='1' then
Sreg0 <= S4;
end if;
when S4=>
OUTPUT <= "101";
if INPUT='1' then
Sreg0 <= S4; elsif INPUT='0' then
Sreg0 <= S3;
end if;
end if; end case;
end process;
Problem 4
In this problem you are asked to design a peak detector circuit. The purpose of this circuit is to alert the user when samples of a signal exceed beyond the threshold level. The circuit consists of a moving average calculation and a peak detector circuit, counters and control signals. A filter calculating the average of the N last samples of a signal is given by:
y(k) = (1/N) · i=n∑N-1 x (k - t)
Draw the logic block diagram of this circuit including all control signals for N = 4 for the following two cases:
a) There is only one two-input adder available.
b) No limitation on number of adders.
Problem 5
a) What is the synthesis result of the following VHDL code? What function does this VHDL code represent?
entity unknown is
port( a: in unsigned (1 downto 0); d: in stdlogic;
z: out unsigned (3 downto 0));
end entity unknown;
architecture rtl of unknown is begin
process (a, d)
variable temp: integer range 0 to 3;
begin
temp := to_integer (a);
for j in z'range loop
if temp = j then
else end if;
end loop end process;
z(j)<= d; z(j)<= '0';
end architecture rtl;
Problem 6
Assume the following specifications for the logic circuit given below: Tmultiplier = 12 ns
Tadder = 5 ns
Tmux = 3 ns TNegation = 2 ns
FF's: Tc-q = 2 ns Tsu = 1 ns Thold = 1 ns
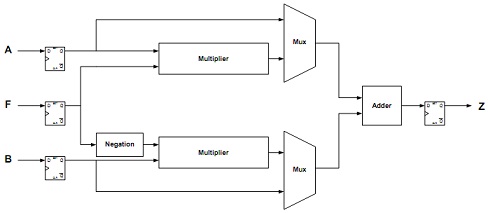
a. Minimum clock period for the circuit given in presence of no skew. Highlight the critical path on your design. Also highlight the shortest path in this design.
Tmin = Tc-q+Tneg+Tmult+Tmux+Tadd+Tsu
= 2+2+12+3+5+1=25ns
b. If you replace the multiplier in part (a) with a two level pipelined multiplier as follows, what would be the speed up for the new design? Calculate the new clock period in presence of no skew.
Tmin = Tc-q+1/3*Tmult+Tmux+Tadd+Tsu
= 2+1/3*12+3+5+1=15ns
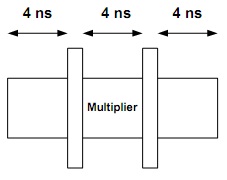
c. Redraw the complete pipelined circuit with new registers inserted in the paths.
d. Fill out the following table using the information obtained above.
|
Latency
|
throughput
|
Unpipelined
|
|
|
3-pipelined
|
|
|
Problem 7
Using shift and add/subtract instructions only, devise efficient routines for the operations by the following constants. Present your answer as a series of add and shift instructions.
a) Divide by 45
b) Multiply by 2.5
Problem 8
Consider the following VHDL code segment.
y <= (others => '0'); if (a > b) then
y <= a - b;
end if ;
if (crtl = '1') then
y <= c;
end if ;
(a) Draw the synthesized logic diagram.
(b) Rewrite the complete code using one if statement.
if ctrl = 't' then
y<= c;
elsif a> b then
y = <= a - b;
else
y<= (other=> '0';
(c) Repeat part (a) and (b) for the following VHDL code fragment. if (a > b) then
y <= a - b;
end if ;
if (crtl = '1') then
y <= c;
end if ;
Problem 9
Draw the state diagram corresponding to the following FSM VHDL model. Is this a Mealy or Moore machine? Why? Also complete the timing diagram for the given FSM code for y, g1, g2, g3.
entity arbiter is
port ( clock, resetn : in std_logic ;
r : in std_logic_vector(1 to 3) ;
g : out std_logic_vector(1 to 3)) ;
end arbiter ;
architecture behavior of arbiter is
type state_type is (idle, gnt1, gnt2, gnt3) ;
signal y : state_type ;
begin
process (resetn, clock)
begin
if resetn = '0' then y <= idle ;
elsif (clock'event and clock = '1') then case y is
when idle =>
if r(1) = '1' then y <= gnt1 ;
elsif r(2) = '1' then y <= gnt2 ;
elsif r(3) = '1' then y <= gnt3 ;
else y <= idle ;
end if ;
when gnt1 =>
if r(1) = '1' then y <= gnt1 ;
else y <= idle ;
end if ;
when gnt2 =>
if r(2) = '1' then y <= gnt2 ;
else y <= idle ;
end if ;
when gnt3 =>
if r(3) = '1' then y <= gnt3 ;
else y <= idle ;
end if ;
end case ;
end if ;
end process ;
g(1) <= '1' when y = gnt1 else '0' ; g(2) <= '1' when y = gnt2 else '0' ; g(3) <= '1' when y = gnt3 else '0' ;
end behavior;
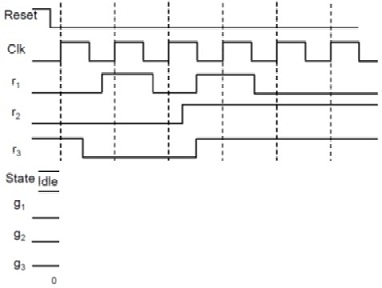
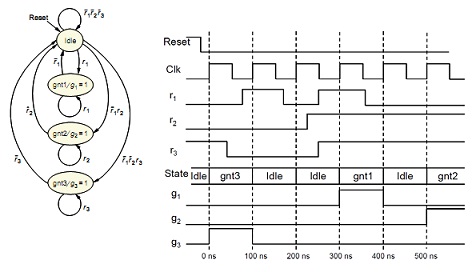