Reference no: EM132931391
Question 1: Write code to build explicitly all combinations of k 1s in n boxes
k=4, n=12
Recursion: Invoke fun(0.-1)
Define fun(bit_num, prev_bit_pos):
1) If bit_num-k,
(a) Print current combination;
(b) return (exit);
2) Otherwise
for(i-prev_bit_pos+1, i<=n-k+bit_num, i++){
(a) Place 1 at position i;
(b) invoke fun(bit_num+1,1);
(c) clear bit at position i;}
Iteration:
1) Consider all integer numbers from 0 to 2"n-1
2) Convert each int number to binary form
3) Compute the popcount (no. of 1s)
4) If popcount-k. this is one of the desired combination
Complexity: O(2^n)
Complexity: O(n! /( k!(n - k)!)))
Question 2:
Q1: a) Write an assembly language program to send continuously E3H to port 2. Timer 1 mode 1 is used to generate the time delay where the starting count start from AFH.
b) Write a program for the 8051 to transfer letter 'T&A' serially at 9600 baud continuously.
Q2:
a) Design a counter system to count number of people walking through a secured door. The electronic sensor system connected to the door gives a pulse output for each person who walks through door connected to T1 of 8051. Display the number of persons cross through the door using LED's connected at Port 2. Use counter 1 operated in mode 2.
c) Write a subroutine which checks the content of 20H. If it is a positive number, the subroutine find its two's complement and store it in same location and returns.
Question 3:
Need making a program (in C, or Java, or C++, or C#) that creates three new threads (besides the already existing main thread) and synchronizes them in such a way that each thread displays it's thread id in turn for 5 iterations. The output of the program should look like this:
Thread 1 - iteration no. 1
Thread 2 - iteration no. 1
Thread 3 - iteration no. 1
Thread 1 - iteration no. 2
Thread 2 - iteration no. 2
Thread 3 - iteration no. 2
Thread 1 - iteration no. 3
Thread 2 - iteration no. 3
Thread 3 - iteration no. 3
Thread 1 - iteration no. 4
Thread 2 - iteration no. 4
Thread 3 - iteration no. 4
Thread 1 - iteration no. 5
Thread 2 - iteration no. 5
Thread 3 - iteration no. 5
Question 4:
"Polybius was an ancient Greek writer who first proposed a method of substituting different two-digit numbers for each letter. The alphabet is written inside a 5-by-5 2d array which a has numbered rows and columns:
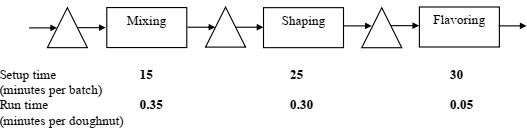
Note both Y and Z are written in the last cell to divide the letters evenly. The context of the message should make clear which of the two letters is intended.
To encode, substitute for each letter the numbers marking the row and column in which the letter appears. Always put the row number first. For example, the number for J is 25. The word WATERMELON would be encoded as
53 11 45 15 43 33 15 32 35 34
To decode, simply locate each letter indicated by the number. The first number, 53, tells you to find the letter at the intersection of the fifth row and the third column."
Test a program that uses the Polybius Checkerboard to encode strings and decode arrays of numbers.
Question 5:
Your task is to write a program, words, that reports information about the number of words read from standard input. For example, if the file qbf .txt contains the text the quick brown fox jumps over the lazy dog then running the program with its input redirected to come from that file will report 9 words
$ words .g qbf .txt
Total: 9
Before you begin, you will need to check out the practical directory from the topic subversion repository. This can be achieved with the svn utility. Open a command prompt, change into your local CP2 practical directory, and enter the following command (replacing FAN with your FAN):
The check out process will create a words directory representing your working copy. You will need to write all of your code within this directory, so be sure to change into it before reading on.
Automatic marking
This practical is available for automatic marking via the FLO quiz Prac 4 hand-in. To check your solution, first commit your code to the topic repository by typing
svn commit -m "replace this sentence with a suitable commit message"
and then enter the revision number into the answer box for the relevant question (level). There are no penalties for incorrect solutions, so if you fail one or more of the test cases, check the report output, modify your solution, and try again. Remember to finish and submit the quiz when you are ready to hand in. You may complete the quiz as many times as you like; your final mark for the practical will be the highest quiz mark achieved.
Background
The following skeleton code for the program is provided in words .cpp, which will be located inside your working copy directory following the check out process described above.
int main (int argc, chars* argv)
{
enum { total, unique } mode = total;
for (int c; (c = getopt(argc, argv, 'Yu")) != -1;)
9d itch(c) { case 't':
mode = total;
break; case 'u':
mode = unique;
break;
}
}
argc -= optind;
argv -= optind;
string word; int count = 9;