Reference no: EM132458299
CSCI3180 - Principles of Programming Languages - The Chinese University of Hong Kong
Assessment - TA Ranking System in COBOL and C
In this assignment, you have to implement part of a TA matching system. Your program should help each course to rank the top 3 TAs. You are required to implement it once using COBOL and once using C.
Assessment Details
Jimmy is designing a simple TA Matching System (the "System" hereafter) to find the most suitable TAs for each course. He is asking you to implement the TA ranking module of this system. The system reads 1) the courses' requirements as well as 2) the candidate TAs' skills and preferences as input, and reports the top 3 TAs for each course, where TAs are ranked by matching scores (to be explained later).
TA ranking system
In this System, course instructors will enter their requirements for TAs: 3 required skills, and 5 optional skills. Candidate TAs will enter their profiles: 8 skills, and their 1st, 2nd and 3rd preferred courses.
The System keeps the course instructors' requirements in a file: instructors.txt. Each line of the file represents the requirements of a course, containing the course ID, required skills, and optional skills. Also, the System keeps another file containing all the candidate TAs' information: candidates.txt. Each line of the file records a candidate TA's information, including the TA ID, skills, and preferred courses. Figures 1 and 2 give an example of the instructors.txt and candidates.txt files (spaces are explicitly displayed as ) respectively. Notice that skill names are padded by spaces so that each skill name takes exactly 15 characters. Some skill names may contain multiple words and have spaces between words, e.g. Shell script .
Jimmy has designed the following policy regarding the matching score that measures how suitable a candidate is to be the TA of a course. The matching score of each pair of (course, TA) is computed as:
score(course, TA) = {1 + skill score + preference_score if all the required skills are satisfied
{0 otherwise

Figure 1: Sample of instructors.txt

Figure 2: Sample of candidates.txt
where
• skill score: number of optional skills satisfied by the TA
• preference score: TA's preference to the course, according to the following table
|
1st preference
|
2nd preference
|
3rd preference
|
preference score
|
1.5
|
1
|
0.5
|
Taking the instructors.txt and candidates.txt in Figures 1 and 2 respectively as an example:
score(3180, 1155136773) = 1 + 5 + 1.5 = 7.5
score(3180, 1152147332) = 0
The output.txt file should not output the matching scores of every TA for each course directly, but reports only the top 3 TAs for each course. Figure 3 is an example of the output.txt file. Note that, if less than k candidates satisfy the required skills specified by the course, the Rank-k TA for that course is filled with 0000000000 :
Besides, pay attention to the cases that the instructors.txt file or the candidates.txt file is empty. If the instructors.txt file is empty, the output.txt file should be empty too; if the candidates.txt file is empty, the Rank-k TA for all the courses should be filled with 0000000000 .
Course ID Rank-1 TA Rank-2 TA Rank-3 TA
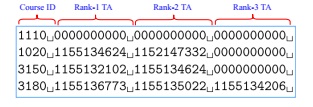
Figure 3: Sample of output.txt
Jimmy needs your help. You are required to implement a program to generate the ranking report of each course.
General Specification
You are required to write two programs, one in COBOL and the other one in C, for the student ranking module of the System. You should name your COBOL source as "ta ranking.cob" and your C source as "ta ranking.c".
Part 1: Input and Output Specification
Your programs should read two input files: instructors.txt and candidates.txt, which contain instructors' requirements and candidates' skills and preferences. The detailed spec- ification of input format is given in Section 2.3. For each course, your program needs to calculate the matching score for each candidate. These calculations should be strictly based on the policy described above. Afterwards, TAs are ranked by their matching scores with each course. In case of a tie (two TAs having the same matching score), the TA with lower TA ID will have a better rank than the other. Your program should output one file to report
the top 3 TAs for each course and display them in ranking order (i.e. the first one is the best TA, the second one is the second best and so on). The output file format should follow the description in Section 2.4. You can "hardcode" the input file names in your program. And the naming of the output file should be: output.txt.
Part 2: Restrictions on using COBOL and C
For COBOL, in order to force you to program as in the old days, ONLY 2 keywords are allowed in selection and loop statements: "IF" and "GOTO". You are not allowed to use modern control constructs, such as if-then-else or while loop. Using any other keywords will receive marks deduction. But for C, you can use whatever you want.
Part 3. Error Handling
The programs should also handle possible errors gracefully by printing meaningful error messages to the standard output. For example, your program should be able to check whether the input file exists or not. If not, display a warning message "non-existing file!". However, you CAN assume that the input files are free of format on content errors.
Part 4. Good Programming Style
A good programming style not only improves your grade but also helps you a lot in debugging. Poor programming style will receive marks deduction. Construct your program with good readability and modularity. Provide sufficient documentation by commenting your codes properly but never redundantly. Divide up your programs into subroutines instead of clogging the main program. The main section of your program should only handle the basic file manipulation such as file opening and closing, and subprogram calling. The main purpose of programming is not just to make the program right but also make it good.
Part 5. Other Notes
You are NOT allowed to implement your program in another language (e.g. Java/Python) and then initiate system calls or external library calls in COBOL and C. Your source codes will be compiled and PERUSED, and the object code tested!
Input File Format Specification
There are two input files: instructors.txt, and candidates.txt. All input files are in plain ASCII text. Each line is ended with the characters " r n" on windows machine, including the last line. You can write your programs on whatever OS you like, but please verify that they can be compiled and run correctly on Windows machines in SHB924/909 because we will grade your assignment there. You should strictly follow the format as stated in the following.
Each line of instructors.txt contains nine fields of fixed length for a course. The lines are sorted by course IDs in ascending order.
1. Course ID : a 4-digit number followed by a space.
2. Required skills: 3 required skills, each of which is a string of 15 characters (spaces are padded at the end in case the skill name is less than 15 characters).
3. Optional skills: 5 optional skills, each of which is a string of 15 characters (spaces are padded at the end in case the skill name is less than 15 characters).
Each line of candidates.txt contains twelve fields of fixed lengths of a candidate's skills and preferences. The lines are sorted by TA IDs in ascending order.
1. TA ID : a 10-digit number followed by a space.
2. Skills: 8 skills, each of which is a string of 15 characters (spaces are padded in case the skill name is less than 15 characters).
3. Preference: 3 preferences, each of which is a 4-digit number followed by a space. You may make the following assumptions on the files:
- All input files strictly follow the format specified in Section 2.3.
- File instructors.txt is sorted by course ID in ascending order.
- File candidates.txt is sorted by TA ID in ascending order.
- The number of skills in file candidates.txt is fixed to 8, and there are no duplicate skills.
Output File Format Specification
There is only one output file: output.txt. You should strictly follow the format as stated in the following.
Each line of output.txt contains 4 fields of fixed lengths of the top 3 TAs for each course. The lines should be sorted by course IDs in ascending order (the same order as in "instructors.txt").
1. Course ID : a 4-digit number followed by a space.
2. Rank-1 TA: The best TA, which is a 10-digit number followed by a space. If no candi- dates satisfy the required skills specified by the course, this field is filled by 0000000000 .
3. Rank-2 TA: The 2nd best TA, which is a 10-digit number followed by a space. If less than 2 candidates satisfy the required skills specified by the course, this field is filled by 000000000 .
4. Rank-3 TA: The 3rd best TA, which is a 10-digit number followed by a space. If less than 3 candidates satisfy the required skills specified by the course, this field is filled by 0000000000 .
Pay attention to the special cases, as we have mentioned in the previous sections:
- For Rank-k TA of a course, if less than k candidates satisfy the required skills specified by the course, the Rank-k TA for that course is filled with 0000000000 .
- If the instructors.txt file is empty, the output.txt file should be empty too.
- If the candidates.txt file is empty, the Rank-k TA for all the courses should be filled with 0000000000 .
- If the input file is non-existent, display a warning message "non-existing file!".
Attachment:- Principles of Programming Languages.rar