Reference no: EM132387908
DATA STRUCTURES AND ALGORITHMS
Question 1
The following program compiles and runs. What is the output of this program?
public class EquilateralTriangle
{
private double base; // the base length of the triangle private double angle; // the angle of the triangle
// constructor without parameters public EquilateralTriangle ()
{
base = 2.0;
angle = 60;
}
// constructor with parameters
public EquilateralTriangle( double b, double a )
{
base = b; angle = a;
}
public double getBase() //method to get base length of the triangle
{
return base;
}
public double getArea() //method to calculate area
{
return (0.4 * base * base );
}
public boolean isTriangle() //method to check if it is an equilateral triangle
{
return (angle == 60);
}
public static void main( String[] args )
{
EquilateralTriangle myTriang1 = new EquilateralTriangle();
System.out.println("Base is: " + myTriang1.getBase());
System.out.println("Area is: " + myTriang1.getArea());
System.out.println("Is it a EquilateralTriangle? " + myTriang1.isTriangle());
EquilateralTriangle myTriang2 = new EquilateralTriangle (1, 60);
System.out.println("Base is: " + myTriang2.getBase());
System.out.println("Area is: " + myTriang2.getArea());
}
}
Question 2
The following code defines class Computer, with a constructor that specifies the Computer's name and cost, and a toString method that returns a String containing the Computer's name and cost.
public class Computer
{
private String computerName; private double computerCost;
public Computer(String name, double cost)
{
computerName = name; computerCost = cost;
}
public String toString()
{
return String.format( "%s cost is %.2f", computerName, computerCost );
}
}
Your tasks are to:
define two set methods to set the computer's name and cost.
define two get methods to retrieve the computer's name and cost.
create a Computer object with the computer name "Dell" and the cost 689, and display a String containing the value of the object's instance variables.
Write java code for the above mentioned tasks.
Question 3
Based on the following Java programs, what will be the output when the
PayrollSystemTest is executed?
public abstract class Employee
{
private String firstName; private String lastName;
public Employee( String first, String last)
{
firstName = first; lastName = last;
System.out.println(firstName +" "+lastName);
}
public abstract double earnings();
}
public class HourlyEmployee extends Employee
{
private double wage; private double hours;
public HourlyEmployee( String first, String last, double hWg, double hWk )
{
super( first, last); wage = hWg; hours =hWk;
}
public double earnings()
{
System.out.println( "the method calculates earnings based on hours" ); if ( hours <= 50 )
return wage *hours; else
return 50 * wage + (hours - 50 ) * wage;
}
}
public class PayrollSystemTest
{
public static void main( String[] args )
{
Employee currentEmployee = new HourlyEmployee("Jim", "Son", 10, 51 ); System.out.println( "Calculate Employee Earnings \n" ); System.out.printf("Earnings $%,.2f\n\n", currentEmployee.earnings() );
}
}
Question 4
What will be the output when the following program is executed? Show all GUI components including frames, combo boxes, buttons and message dialog boxes.
import javax.swing.*; import java.awt.*; import java.awt.event.*;
public class MyTimetable extends JFrame
{
private JPanel panel; private JButton exitButton;
private JComboBox dataBox;
public MyTimetable()
{
super("My Timetable for Programming Courses");
setSize(400, 300); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
dataBox = new JComboBox(); dataBox.addItem("Programming Course COIT20245");
dataBox.addItem("Programming Course COIT20256");
exitButton = new JButton("EXIT");
dataBox.addActionListener(new DataComboBoxListener());
exitButton.addActionListener(new ExitButtonListener());
panel = new JPanel(); panel.add(dataBox); panel.add(exitButton); add(panel);
setVisible(true);
}
private class DataComboBoxListener implements ActionListener
{
public void actionPerformed(ActionEvent e)
{
int index = dataBox.getSelectedIndex(); switch (index)
{
case 0: JOptionPane.showMessageDialog(null, "Mon 8-10am"); break; case 1: JOptionPane.showMessageDialog(null, "Fri 9-11am"); break;
}
}
}
private class ExitButtonListener implements ActionListener
{
public void actionPerformed(ActionEvent e)
{
System.exit(0);
}
}
public static void main(String[] args)
{
MyTimetable myFrame= new MyTimetable();
}
}
Question 5
The following program is based on linked list operations. What will be the output when this program is executed?
import java.util.List; import java.util.LinkedList;
import java.util.ListIterator;
class LinkedListTest
{
public static void main(String[] args)
{
LinkedList<String> myList = new LinkedList<String>(); ListIterator<String> iter;
myList.add("Apple"); System.out.println("List: "+myList); myList.addLast("Mango"); myList.addLast("Orange"); System.out.println("List: "+myList); iter = myList.listIterator(); iter.next(); iter.next();
iter.add("Grapes");
iter.add("Banana"); System.out.println("List: "+myList);
while (iter.hasNext()) System.out.println("List: "+ iter.next()); iter.add("Apricot");
System.out.println("List: "+myList);
}
}
Question 6
Assume that each of the following operators =, <, &&, [ ], ==, ++ counts as an operation. What will be the worst-case running time for the following code? Justify your answer (write maximum four lines).
int maxVal=3, searchKeyFound=1; for (int i=1; i<N+1; i++)
{
if ( (i<N+1) && (myValue[i] == maxVal) ) searchKeyFound = 0;
else
}
searchKeyFound = 1;
Question 7
Suppose there is a text file (movies.txt) with data format (movie name, download cost) as below:
Jurassic World 10
The Sting 10
The Godfather 5
The following program contains the partial code. Complete the program code so that it can read the data file (movies.txt) and display the following information on the screen.
movie name.
total number of movies.
all movie names with download cost equal to 5. import java.io.*;
import java.util.*; public class FileTest
{
public static void main(String[] args) throws java.io.IOException
{
int countMovies=0; try
{
File movies=new File("movies.txt "); Scanner input=new Scanner(movies); while (input.hasNext())
{
String name1 = input.next(); String name2 = input.next(); int cost = input.nextInt();
//Display the movie name and increment countMovies
/*Student to COMPLETE*/
//Display the movie names which have download cost equal to 5
/*Student to COMPLETE*/
}
//Print the total number of movies
/*Student to COMPLETE*/
//Close the file
/*Student to COMPLETE*/
}
catch (FileNotFoundException fileNotFoundException)
{
/*Student to COMPLETE*/
}
}
}
Question 8
What is the output from the following sequence of queue operations? Assume that the PriorityQueue class here is a class that has implemented standard Queue operations offer, peek and poll.
PriorityQueue<Integer> q = new PriorityQueue<Integer>(); int num1 = 100, num2 = 102;
ffer(89); q.offer(num1); q.offer(33);
oll(); System.out.println(q.peek()); q.poll(); System.out.println(q.poll()); q.offer(303);
q.offer(num2); System.out.println(q.peek());
while (!q.isEmpty()) System.out.print(q.poll() + " ");
Question 9
The method findMax() takes an integer array as an argument. It returns the maximum value stored in the array.
public static int findMax(int [] myNumbers)
{
int max= 0;
for (int i = 0; i < myNumbers.length; i++) {
if (myNumbers[i]>max) max= myNumbers[i];
}
return max;
}
Write a generic version of findMax() method that uses a generic type T array instead of an integer array, so that this method can work with an array of integer and double types. Write a demonstration program to verify this method with the following arrays:
Integer [] intArray={4, 10, 2, 15};
Double [] doubleArray={3.2, 2,4, 3.4, 4.6};
Question 10
What does the following program do (e.g. power, square root, greatest common divisor, factorial, etc.)? Justify your answer (write maximum 3 lines). What will be the output of this program?
public class Recursion
{
public static int guessProg( int num1, int num2 )
{
if ( num2 > 0 )
return guessProg(num1, num2-1)*num1; else
return 1;
}
public static void main(String[] args)
{
System.out.println(guessProg (2,3));
System.out.println(guessProg (4,2));
}
}
Question 11
What are the values of A and B in the tree shown below so that it is a binary search tree? List the values of A and B. Manually provide the inorder, preorder and postorder traversals of the search tree shown below.
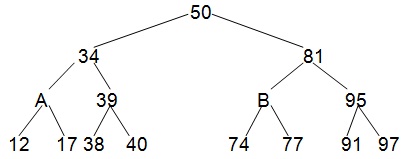
Attachment:- DATA STRUCTURES AND ALGORITHMS.rar