Reference no: EM132387896
Central Queensland University
Course: Data Structures and Algorithms
Subject Area: COIT
Catalog Number: 20256
Term 1 Standard examination
DATA STRUCTURES AND ALGORITHMS - COIT20256
Question 1
The following program compiles and runs. What is the output of this program? public class CricketResult {
private int runs; // the number of runs
private String gameName; // name of the game series
private String location; // game location
// default constructor public CricketResult ()
{
runs = 0;
gameName = "not set"; location = "undecided"
}
//parameterised constructor
public CricketResult(int run, String name, String loc )
{
runs = run; gameName = name; location =loc;
}
public String getGameName()
{
return this.gameName;
}
public String getGameLocation()
{
return this.location;
}
public int getRuns()
{
return this.runs;
}
public static void main(String [] args ) { CricketResult defaultGame = new CricketResult();
System.out.printf("\n %s %s %d ",defaultGame.getGameName(), defaultGame. getGameLocation(), defaultGame.getRuns());
CricketResult NZvsAusTest = new CricketResult (52,"firstTest", " Wellington") ; System.out.printf("\n%s %s %d ", NZvsAusTest.getGameName(),
NZvsAusTest. getGameLocation(), NZvsAusTest.getRuns());
}
}
Question 2
The following code defines class MusicAward, with a constructor that defines the name of the award, and year of award, and a toString() method that returns a String containing the MusicAward's name and year.
public class MusicAward { private String awardName; private int awardYear;
public MusicAward (String award, int year)
{
this.awardName = award; this.awardYear = year;
}
@Override
public String toString()
{
return String.format( "Award: %s Year: %d", this.awardName, this.awardYear );
}
Write java code for the four methods given below and a main method to create a MusicAward object having award name - ARIA, and award year - 2015.
- Two set methods to set the values of awardName and awardYear.
- Two get methods to return the awardName and awardYear.
Use the following Software class declaration to create a subclass called Game. The Game class will have additionally:
- Two private data members of type String to store the usage (networked or standalone), and purpose (entertainment, or educational).
- A constructor that uses the super class constructor.
- A toString() method that can be used to display the private data member values. public class Software {
private String name; private float price;
public Software (String name, float price)
{
this.name = name; this.price = price;
}
@Override
public String toString()
{
return String.format("\n Software name: %s and price is $%.2f", this.name,
this.price);
}
}
Question 4
What will be the output when the following program is executed? Show all GUI components. Assume the textField StudentName is not entered, the course name "Project" is selected in the Combo box, and displayJButton is clicked.
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
public class CourseInfo extends JFrame {
private final JComboBox<String> coursesJComboBox; // holds course names private JLabel choiceLabel;
private final JButton displayJButton; private JTextField displayfield; private String courseInfo;
private JLabel lblStudentName; private JTextField txtStudentName; private JLabel lblStudentId ;
private JTextField txtStudentId;
private static final String[] CourseNames = {"Responsive Web Design", "Database",
"Sofware Engineering", "Project", "Data Structures"}; private static final String[] CoordinatorNames = {"Andrew Chiou", "Brijesh Verma",
"Dennis Jarvis", "Lily Li", "Mary Tom"};
// QuestionFour constructor adds JComboBox to JFrame public CourseInfo() {
super("Display Course Coordinator ");
displayJButton = new JButton("Display Coordinator"); JPanel topPanel = new JPanel(new GridLayout(2,1));
coursesJComboBox = new JComboBox<String>(CourseNames); coursesJComboBox.setMaximumRowCount(5); // display five rows txtStudentName = new JTextField(15);
txtStudentId = new JTextField(15); lblStudentName = new JLabel("Student Name"); lblStudentId = new JLabel("StudentId"); displayfield = new JTextField(18);
choiceLabel = new JLabel("Course Coordinatr"); JPanel RowOnePanel = new JPanel(new FlowLayout()); JPanel RowTwoPanel = new JPanel(new FlowLayout());
RowOnePanel.add (lblStudentName); RowOnePanel.add (txtStudentName); RowOnePanel.add ( lblStudentId ) ; RowOnePanel.add ( txtStudentId);
RowTwoPanel.add (coursesJComboBox); RowTwoPanel.add (displayJButton); RowTwoPanel.add (choiceLabel); RowTwoPanel.add (displayfield);
topPanel.add (RowOnePanel); topPanel.add (RowTwoPanel);
add (topPanel, BorderLayout.NORTH);
coursesJComboBox.addItemListener(
new ItemListener() { // anonymous inner class
// handle JComboBox event @Override
public void itemStateChanged(ItemEvent event) {
// determine whether item selected
if (event.getStateChange() == ItemEvent.SELECTED)
{
courseInfo = CoordinatorNames[coursesJComboBox.getSelectedIndex()];
}
} // end anonymous inner class// end anonymous inner class
}
); // end call to addItemListener
displayJButton.addActionListener( new ActionListener() {
@Override
public void actionPerformed(ActionEvent event) { displayfield.setText(courseInfo); choiceLabel.setText( " Course Coordinator" );
}
}
); // end call to addActionListener
} //end constructor
public static void main(String args[]) { JFrame testFrame = new CourseInfo();
testFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); testFrame.setSize(600, 600);
testFrame.setVisible(true);
}
} // end class CourseInfo
The following program is based on linked list operations. What will be the output when this program is executed?
import java.util.LinkedList; import java.util.ListIterator; public class CourseList {
public static void main(String[] args)
{
LinkedList<String> listOfCourses = new LinkedList<String>(); ListIterator<String> iter;
listOfCourses.add("COIT20257"); System.out.println("List: "+ listOfCourses);
listOfCourses.addFirst("COIT20249"); listOfCourses.addFirst("COIT20245"); System.out.println("List: "+ listOfCourses);
listOfCourses.removeLast(); listOfCourses.add(1,"COIT20246" ); System.out.println("List: "+ listOfCourses);
iter = listOfCourses.listIterator(); iter.next();
iter.next(); iter.add("COIT20248");
System.out.println("List: "+ listOfCourses);
listOfCourses.addLast("COIT20256"); System.out.println("List: "+ listOfCourses);
}
}
Question 6
(a) By using the definition of Big-O, show that the running time term T(n)=78+2n + n2 is O(n2 ). Write your answer in no more than three or four lines.
(b) For the following running time expressions, what would be the order of the growth rate of each in terms of Big-O notation?
i. 2n +5n
ii. n3 + 2n-1 +5 log2n
iii. 2n2 +10√n +15
Question 7
Suppose there is a text file - enrolments.txt with data format (course code, number of students enrolled) as given below:
COIT20245
|
160
|
COIT20246
|
220
|
COIT20256
|
90
|
The following program contains the partial code. Complete the program code so that it can read the data file (enrolments.txt) and display the following information on the screen.
- course code
- Total number of entries in the file.
- The course with the highest enrolments
import java.io.*; import java.util.*;
public class Enrolments { private static Scanner input;
public static void main(String[] args) {
int totalNumberOfCourses=0; String highestEnrolledCourse = ""; String code = "";
int NoOfEnrolments = 0; int maxEnrolment =0; File enrolments;
// open file for reading and throw exception for failure to open. try {
enrolments = new File("enrolments.txt"); input = new Scanner(enrolments);
}
// Student to complete
catch (FileNotFoundException fileNotFoundException) {
}
// process data. Student to complete
try {
// read file, count number of entries, compute maximum number of enrolments,
// highest enrolled course, display code, and number of enrolments
while (input.hasNext() {
}
}
catch (NoSuchElementException elementException) { System.err.println("File improperly formed. Terminating.");
}
catch (IllegalStateException stateException) { System.err.println("Error reading from file. Terminating.");
}
// Student to complete: display Course code and number of enrolments in
// the highest enrolled course.
// close file. Student to complete
} // end main
} //end class
What will be the contents of the stack after the characters in String display are pushed into the stack? Write the missing lines of code as indicated in the source code below. Write the output of the program.
import java.util.*;
public class StackQueue {
public static void main (String [] args)
{
Stack<Character> stack = new Stack<Character> ();
// LinkedList is used to create a queue. So only queue operations can be done.
LinkedList<Character> queue = new LinkedList <Character>();
int count =0;
String display = "yadhtrib yppah" ; while (count < display.length())
{
stack.push(new Character(display.charAt(count))); count++;
} // Write contents of stack now
//move contents of stack into the queue
//write the missing lines of code
while (!stack.empty())
{
}
while(!queue.isEmpty()) System.out.print(queue.removeFirst());
}
}
The following program converts a collection of values which may contain duplicates to a Set which is one of the collections. Write the output of the program.
import java.util.*;
public class SetGeneric <T > {
LinkedList <T> input;
Set<T> set;
// constructor
public SetGeneric( Collection<T> values )
{
set = new HashSet<T>(values); input = new LinkedList<T>(values);
} // end constructor
@Override
public String toString()
{
return String.format("Original values : %s, \n Set values: %s", input.toString(),
set.toString());
}
public static void main (String [] args)
{
Integer [] numbers = {23, 45, 67, 12, 5, 7, 8, 23, 12, 65};
List <Integer> values = Arrays.asList (numbers);
SetGeneric numberSet = new SetGeneric (values); System.out.println (numberSet);
}
}
In the binary search tree shown below certain values are misplaced by not following the binary search tree rules. Correct the misplaced values.
What are the values of A and B in the tree shown below so that it is a binary search tree? List the values of A and B. Manually provide the inorder, preorder and postorder traversals of the binary search tree with values of A, B, and all values correctly placed.
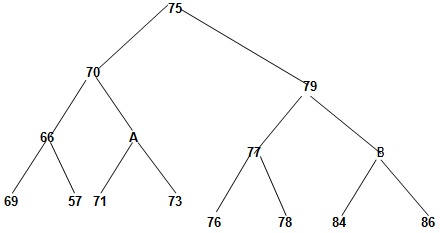
Question 11
Write a method to calculate xy recursively. For example, if x is 2, and y is 3, it will be 23 and the method should return 8. Write a main method to test your method.