Reference no: EM132526027
COIT20245 Introduction to Programming - Central Queensland University
1. Introduction
In this assignment, you are to implement a console applicationfor the management of a premiership table for an Australian Rules football league. The table will have the structure shown in Figure 1.
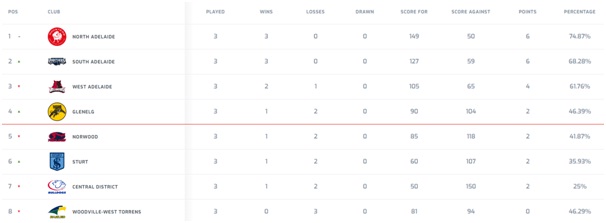
Figure 1. Premiership table for the SANFL Women's League after Round 3
In this particular league, there are 8 teams, with names of your choosing- I recommend t1-t8. Also, the table displays team names only and not team icon + team name, as in Figure 1. Position in the table is determined on the basis of premiership points (points in Figure 1); teams with the same number of premiership points are ranked accordingto percentage. Premiership points are awarded for each game -2 to the winning team, 0 to the losing team and if the game is drawn, 1 to each team. As described in Assignment 1, the outcomeof a game is determined using the points scored by each team, where points = goals*6+behinds. The percentage for each team is calculated as follows:
percentage = scorefor / (score for + score against)
where
• score for is the total number of points scored by the team in all games played so far and
• score against is the total number of points that the opposing teams have scored in all games played so far
Note that in the AFL, the premiership points available per game and the way in which percentage is calculated are different, but the ranking process is the same. And what happens, if at the end of the season, two teams have the same number of premiership points, score for and score against? I don't know.
The Application
The current state of the premiership table is to be stored in 6 arrays, using array initialisers. E.g.
// The data is from a sorted table, so there is no need to sort the table
// once it is generated.The data is from the SANFL women's league, where
// scores are lower thanin the AFL. Also in the SANFL, premiership
// points and percentages are calculated differently to the AFL.
String teams[] = {"t1", "t2", "t3", "t4", "t5", "t6", "t7", "t8"};
int wins[] = {3, 3, 2, 1, 1, 1, 1, 0};
int losses[] = {0, 0, 1, 2, 2, 2, 2, 3};
int drawn[] = {0, 0, 0, 0, 0, 0, 0, 0};
intscoreFor[] = {149, 127, 105, 90, 85, 60, 50, 81};
intscoreAgainst[] = {50, 59, 65, 104, 118, 107, 150, 94};
Note that not all columns in Figure 1 are present. Team position is determined from its index in the arrays (add 1 to the index). The remaining columns can be derived from the above data.
The raw data is to be used to create objects of type Entry, which are to be stored in an array of type Entry. The Entry class is to conform to the class shown in the UML Diagram of Figure 2 .Note that in Figure 2, the visibility of class methods and attributes are designated as + (public) and - (private). Associations are annotated with a label (for readability) and multiplicity. The multiplicity captures the number of object instances that can be involved in the association. In this case, only two values apply - 1 and *, which means many.
Creation of the Entry array is to occur when the Table object is created, using a private method called load(). This method will iterate through the 6 arrays that define the current state of the table, construct Entry objects and add them to the entry array.As befitting a premiership table, the data array is ordered on position, as in Figure 1. When the table is updated, the table will need to be sorted. As noted in Section 1, this will require sorting on two attributes -the primary attribute ispremiership points and the secondary attribute ispercentage. The easiest way to do this is to define a compare function:
private int compare(Entry e1, Entry e2) {
// Returns
// -1 if e1 < e2
// 0 if e1 == e2
// +1 if e1 > e2
}
Your (private) sort method must implement selection sort.
The application's Controller classis to execute (using a switch statement) the following command options:
1. Display available commands
2. Display current table
3. Display selected statistics
4. Display the entry for a specified team
5. Display entries for teams with the same points as a specified team
6. Add a new result
7. Exit the application
For 3, the statistics to be generated are
In the unlikely event that two or more teams have the highest score for or the lowest score against, just return the first of the teams.
For 6, the premiership table must be updated
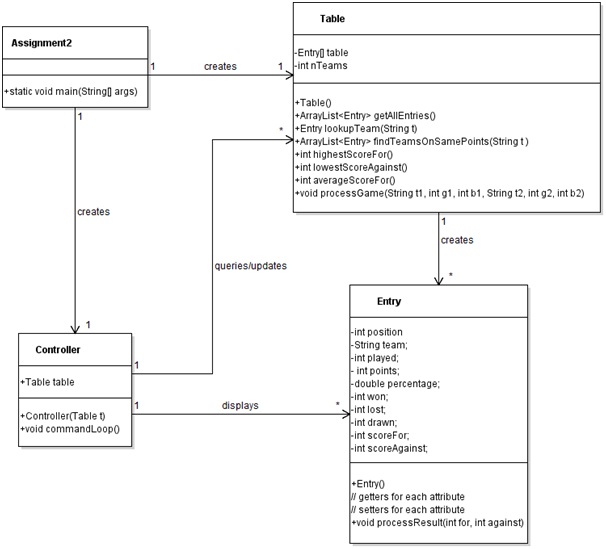
Figure 2. Class Diagram
As it is a console application, the user will need to be prompted to enter a command and its arguments (if any). My personal preference is for a minimal interaction scheme, as shown below:
run:
Available commands are:
help 0
display entries 1
display selected statistics 2
lookup a specified team 3 team
find teams with same points as a specified team 4 team
add a new result 5 team1 g1 b1 team2 g2 b2
quit 9
> 3 t4
Pos Team Played Points % Won Lost Drawn PF PA
4 t4 2 2 48.8 1 1 0 59 62
>
Feel free to adopt the above scheme or if you prefer, implement a more verbose interaction scheme.
Note that
1. Each command is designated a number
2. The command options are displayed at the start of the application and whenever a "help" command is entered, rather than after each command.
3. Percentages are to be displayed to 1 decimal point
For the commands that require arguments
1. For commands 3, 4 and 5, an error message must be displayed if a specified team is not in the table. No other data validation is required.
2. The application must conform to the class diagram of Figure 2, although additional private members and private methods are permitted.
Attachment:- Introduction to Programming.rar