Using a servlet to respond to user input:
We extend the previous example to show how to write a servlet that will process data from an HTML form. As we noted earlier, servlets are versatile and can also be applied to many other activities.
First, we must briefiy explain HTML forms. Most people will have used online forms as they are commonly used for input of personal data when making purchases or subscribing to organizations or events on the web. An area of a web page can be defined as a form, by a section of HTML enclosed in <FORM > and </FORM > tags. A web page can have one or more forms, defined within the body of the HMTL page. Between the <FORM > and </FORM > tags, normal text or other HTML tags can be used. In addition, there are special tags that can define user interface components such as text fields, command buttons, option buttons, and so on.
Let us create a simple form that allows a user to log in to the lottery system introduced above. This will prove useful later when we consider how the system could be used to actually submit a lottery entry. In a real online lottery system a form might have lots of security features, help systems and sophisticated layout but, for this example, we shall keep the information and the form layout very simple.
Figure shows how the form would look, when viewed using a browser.
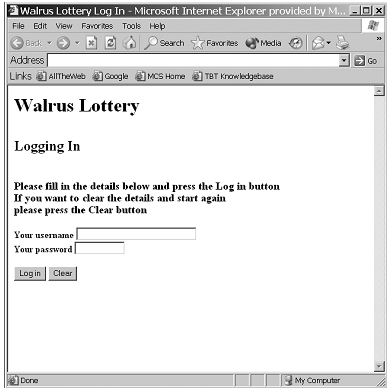
Figure: The HTML form page for logging in, viewed using a browser
The HTML required for the form part of this web page is as follows.
<FORM METHOD = "POST" ACTION = "/servlet/WalrusLottery/LotteryLogin"> Your username
<INPUT TYPE = "TEXT" NAME = "USERNAME" SIZE = "30"> <BR> Your password
<INPUT TYPE = "PASSWORD" NAME = "PASSWORD" SIZE = "10"> <BR>
<BR>
<INPUT TYPE = "SUBMIT" NAME = "LOGIN" VALUE = "Log In">
<INPUT TYPE = "RESET" NAME = "RESETLOGIN" VALUE = "Clear">
</FORM>
In order to concentrate on the structure of the form, we have omitted the standard HTML to set up the head and body parts of the web page and to display the supporting text at the start of the page. The <FORM > tag contains two attributes. The METHOD attribute indicates that the result of sending the form will be an HTTP POST request. The ACTION attribute specifies that this request will be processed by a servlet called LotteryLogin in the WalrusLottery domain.
The two text fields are defined using the <INPUT > tags with appropriate type parameters. For the user name field, the TYPE parameter is set to "TEXT" while for the password field, the type parameter is set to "PASSWORD" to ensure that the contents are not displayed when the user fills in this field. The SIZE parameter specifies the size of the text field in number of characters, although normally the text field will scroll to allow additional characters beyond this limit. The NAME parameter specifies a name for the text field - this will be used by the servlet that processes the data. Each <INPUT > tag is preceded by some plain text to tell the user what to input.
Two of the standard button types associated with forms are specified by setting the TYPE attribute of the <INPUT > tag to "SUBMIT" or "RESET" respectively, as shown above. When the SUBMIT button is clicked, the HTTP request specified in the <FORM > tag (in this case, a POST request) is sent for processing. The RESET button clears all the text fields on the form, allowing the user to start filling in data again, normally because the data was completely wrong. The user sees these buttons labelled as the Login button and the Clear button respectively, as defined by the VALUE attribute of the
<INPUT > tag.
The response we expect after submitting this form will be a web page, with two possible outcomes. If there was missing or erroneous information, we could expect a page with an error message, indicating what had gone wrong. If all was well, we would expect a web page confirming a valid login and inviting the user to proceed with their lottery entry. To keep this example simple, we shall assume that a successful login results in the servlet sending back a set of lucky numbers, as before.
The servlet class LotteryLogin needs the following structure.
- Inherits from class HttpServlet.
- Overrides the doPost method.
- Checks that all input data is present and corresponds to the previously registered user name and password.
- Returns a suitable error page if any data is missing or erroneous.
- If the data is correct, it returns a web page confirming the login and containing a set of 'lucky numbers'.
The start of the code for the class LotteryLogin is as follows.
public class LotteryLogin extends HttpServlet
{
public void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException
{
try
{
UserData user = getUserData(req);
sendLuckyNumbers(resp, user);
}
catch (BadUserDataException e)
{
}
}
...
sendErrorReport(resp, e);
This shows the only public method of the servlet, doPost, which constructs and sends the response to a POST request from the client. This method has two parameters that reference objects corresponding to the request data received and the response to be constructed respectively. The code above uses three helper methods, getUserData, sendLuckyNumbers and sendErrorReport to do all the work. We shall look at these in more detail below but, briefiy, the operation of the doPost method is as follows. It invokes the getUserData method to check the data input onto the form by the user and to store this in a UserData object. If there are any problems with the data, the method will throw a BadUserDataException, which is handled by the catch clause of the doPost method. If the data is valid, the doPost method will invoke the method sendLuckyNumbers to send back a set of numbers, as before. If an exception occurs due to bad input data then the method sendErrorReport will be invoked to send a suitable response page. Clearly, this is a rather simplistic approach, but we are mainly concerned with demonstrating the basic principle of servlets here.
An outline of the helper method getUserData is as follows.
private UserData getUserData (HttpServletRequest req) throws BadUserDataException
{
UserData user = new UserData( );
String userName = req.getParameter("USERNAME");
if (userName.length( ) == 0)
{
throw BadUserDataException("Invalid user name");
}
user.setName(username);
String userPassword = req.getParameter("PASSWORD");
if (userPassword.length( ) == 0)
{
throw BadUserDataException("Invalid password");
}
user.setPassword(userPassword);
return user;
}
Again, this method takes a very simplistic approach. In a more realistic example, it would check the user name and password against values previously stored in a database. The obvious errors of blank user name or password could also be more efficiently detected using JavaScript code running on the client system. However, this method does show the basic approach to extracting data from the HTTP request object, using the getParameter method. This method returns a String corresponding to the specified item on the HTML form. Consider the following line from the method code above:
String userName = req.getParameter("USERNAME");
This extracts the value entered in the text field labelled "USERNAME" by the following line that we saw earlier in the HTML form:
<INPUT TYPE = "TEXT" NAME = "USERNAME" SIZE = "30"> <BR>
If the getUserData method finds any errors in the input data, it throws an exception including a suitable error message that can ultimately be returned to the user. We omit details of the class UserData, but its general structure should be clear from the above code examples.
Returning to the doPost method again, remember that it invokes one of two other helper methods to send a response back to the client. We briefiy indicate here how these might work, without going into detailed code.
The sendLuckyNumbers method would be almost identical to the code in the doGet method of the class LotteryLuckyNumbersServlet in Subsection 4.3 above. In this case, it would also be possible to confirm the successful log in and to display the user name as part of the response. An alternative approach would be for this method to pass the request on to the LotteryLuckyNumbersServlet, which could be running on the same server or even on a different server - the details of this are beyond the scope of this unit.
The sendErrorReport method could simply return a standard static web page indicating a log in error. It would be more helpful to the user if this method were to construct a dynamic web page, containing the error message associated with the BadUserDataException. In the next subsection, we shall see that a servlet may not be the best way to achieve this, and that the related technology of Java Server Pages (JSP) is more appropriate in this case.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your Using a servlet to respond to user input homework and assignments? Live Using a servlet to respond to user input experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer Using a servlet to respond to user input homework help, java assignment help and Using a servlet to respond to user input projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours