The Iterator interface in Java:
We have seen that a Java interface is not a class - it cannot be instantiated. You can, however, declare variables of an interface type. Any class that implements the interface may be referenced by such a variable. So, using the example from the previous section, the following is possible:
Comparable<Employee>employee1 = new Employee(...);
To show how a variable of an interface type can be used, we consider the Iterator interface. This allows you to traverse (or 'iterate' through) any collection, such as an ArrayList, without needing to know details of its implementation. Table lists the three methods for the Iterator interface.
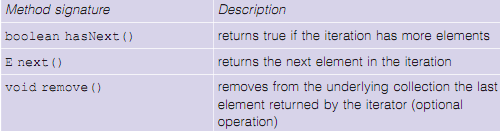
You can access each element of a collection in turn by repeatedly invoking the next method. You use the hasNext method to check if there are any more elements to iterate through.
Most collection classes in Java have an associated iterator - an object that implements the Iterator interface for their type. Table described the iterator method for the ArrayList class - this returns a reference to an object that implements the Iterator interface. We can use it to display the items in an ArrayList, as follows:
ArrayList<String> userList = new ArrayList<String>();
...
Iterator <String>listIt = userList.iterator(); System.out.print("The list is [");
while (listIt.hasNext())
{
System.out.print(listIt.next() + " ");
}
System.out.println(" ]");
To help understand this code, let us look at a specific example. Suppose that the ArrayList object userList contains the user IDs of four users. We represent this diagrammatically as in Figure, for simplicity ignoring the fact that the ArrayList actually stores references rather than the strings themselves.

Table shows the result of executing the above code for each iteration of the while loop. The second and third columns show the values returned by the iterator methods hasNext and next during each iteration - strictly speaking, the next method returns an object reference, rather than the characters themselves.
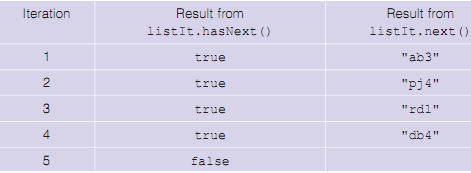
The loop terminates after the test at the beginning of the fifth iteration. The final printed output from this would be as follows:
The list is [ab3 pj4 rd1 db4 ]
In this example, listIt is a variable of the interface type Iterator. It can reference any object that implements the Iterator interface. Hence, almost exactly the same code can be applied to any collection that provides an iterator. To use a different type of list, the linked list, we would need to change only the first line of the above example, as follows:
LinkedList<String> userList = new LinkedList<String>();
...
Alternatively, we could use yet another type of collection, which implements the mathematical concept of a set, simply by changing the first line to this:
HashSet<String> userList = new HashSet<String>();
...
Note that we can regard iterators as disposable. Once we have completed the traversal of a collection, using an iterator, we no longer need the iterator object and it can be made available for garbage collection by the system. If we need to traverse a collection again later, it is normally best to obtain a new iterator object.
You might be tempted to ask: why are you teaching us Iterator objects. After all, the for-each statement will do the same thing. In general, this is correct: if you are just going to traverse a collection then use the for-each statement. However, if you need to remove items from a collection then you'll need to use an Iterator object and, in particular, the method remove. This deletes from the collection the item returned by the most recent call to next. This is the preferred approach when you need to remove a number of data items from most collections because it is normally more efficient.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your The Iterator interface in Java homework and assignments? Live The Iterator interface in Java experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer The Iterator interface in Java homework help, java assignment help and The Iterator interface in Java projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours