The GameCanvas:
Painting the display
A GameCanvas is where all the game objects are displayed. It has a Graphics object, which defines what should appear on the screen; by using this Graphics object we can arrange to display whatever graphics we want.
The GameCanvas has an off-screen buffer where the graphics are constructed preparatory to being displayed. The buffer is then fiushed to the screen. This makes for much smoother graphics drawing.
Using GameCanvas graphics could not be simpler. Here's a little class (not part of the case study) that draws a red rectangle, some green text and then some blue text. For simplicity we have placed all the drawing statements in its constructor. Recall that the origin of the coordinate system for Graphics is the top-left corner, with axes pointing horizontally to the right and vertically down.
import javax.microedition.lcdui.*;
import javax.microedition.lcdui.game.*;
public class DemoCanvas extends GameCanvas
{
private Graphics g; // lcdui.Graphics
public GameScreen()
{
super(true);
g = getGraphics();
g.setColor(0xff0000); // red
g.fillRect(10, 50, 50, 70); // x, y, width, height
g.setColor(0x00ff00); // green
String message = "The canvas width is " + getWidth();
g.drawString(message, 10, 10, g.TOP|g.LEFT);
g.setColor(0x0000ff); // blue
message = "The canvas height is " + getHeight();
g.drawString(message, 10, 30, g.TOP|g.LEFT);
flushGraphics();
}
}
In an associated MIDlet class we create and display an instance of DemoCanvas:
public void startApp()
{
This simple code will not work in a real game.
DemoCanvas canvas = new DemoCanvas();
display.setCurrent(canvas);
}
The result is shown in Figure.
Each of the six digits can vary independently, apparently giving
16 777 216 colours. Mobile devices currently don't support anything like this number: 65 536 or 4096 colours are typical.
There is also a setColor (int red, int green, int blue) method, but this is less common than the single-argument version we have described.
Figure: A simple drawing on a GameCanvas
Some explanation is needed of how the colours are set. Each colour is described by an int, written in the form 0x00RRGGBB, where each of the R, G and B symbols stands for a hexadecimal digit in the range 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, a, b, c, d, e, f. To make the red component of the colour as large as possible, we would replace RR by ff. To have no red at all, we would replace the RR by 00. The blue and green components work in the same way. So 0x00000000 switches all three components off, giving black.
0x00ffffff turns them all up to full, giving white. Different combinations give other colours; for example 0x00ffff00 is yellow. The high-order byte of this value is ignored. For example, 0x00ff0000 is equivalent to 0xff0000, so you may also see colours with only six hexadecimal digits.
So in our code above 0xff0000 is red, 0x00ff00 is green, 0x0000ff is blue.
Notice that the rectangle is drawn relative to the top left of the canvas and text position is defined relative to an anchor g.TOP|g.LEFT in the same position. Text can be drawn relative to anchors in other positions, but this is the only anchor we shall be using. Notice also that there are methods that let us find the size of the canvas.
Drawing layers
To draw layers is also very simple. Adding the following code to our canvas draws a Sprite at position (100, 150). The try-catch is needed because the attempt to read the graphics file can result in an IOException.
try
{
Image arrowImage = Image.createImage("arrow.png");
Layer arrow = new Sprite(arrowImage);
arrow.move(100, 150);
arrow.paint(g);
}
catch (Exception e)
{
e.printStackTrace();
}
The display will now be as shown in Figure.
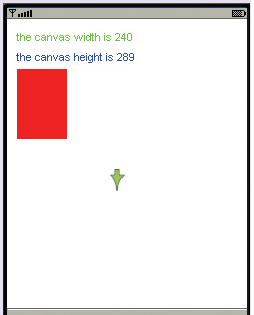
Figure: A Sprite has been added
Polling the keys
To find out whether the user has pressed any keys we use an idiom similar to the following:
while (true)
{
int keyStates = this.getKeyStates();
if((keyStates & RIGHT_PRESSED) != 0)
{
System.out.println("right");
}
}
This example loops round, asking the keys if they have been pressed. The information about which ones have is passed back as a number. The code keyStates & RIGHT_PRESSED checks to see if the right arrow key was amongst them and if so prints out a message.
Checking other keys works the same, so to check for a left arrow press we use keyStates & LEFT_PRESSED and so on. In our game we use only the four multidirectional keys. You can find a full list of keys from the Games API documentation.
This polling of the keys is convenient to use. However, to use it we have to make our game canvas run as a separate thread. To do this we modify our class as follows:
import javax.microedition.lcdui.*;
import javax.microedition.lcdui.game.*;
public class DemoCanvas extends GameCanvas implements Runnable
{
private Graphics g; // lcdui.Graphics
public DemoCanvas()
{
super(true);
Thread thread = new Thread(this);
thread.start();
g = getGraphics();
// unchanged graphics drawing code goes here
flushGraphics();
}
public void run()
{
while (true)
{
int keyStates = this.getKeyStates();
if((keyStates & RIGHT_PRESSED) != 0)
{
System.out.println("right");
}
}
}
}
This uses another idiom very common in mobile applications; the self-launching thread. DemoCanvas now implements Runnable and has a run() method in which the key polling takes place. To launch itself it creates the Thread in which it will run, passing itself to the constructor as an argument, then starts the thread off.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your The GameCanvas homework and assignments? Live The GameCanvas experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer The GameCanvas homework help, java assignment help and The GameCanvas projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours