The client code:
This section describes the client code for the name service. The client user interface consists of a single window, containing two labelled text fields and two buttons, as shown in Figure.
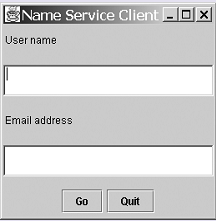
Figure: The client user interface window
The Go button sends the employee's user name in the first text field to the server. When the server replies with the email address of the user, this is displayed in the second text field. The Quit button disconnects the client from the server and closes the client.
The client class is declared as follows:
public class NameClient extends JFrame implements ActionListener
{
...
This means that the NameClient class is a kind of frame; it inherits from the JFrame class provided by the Swing library of user interface classes. It also implements the interface ActionListener - this ensures that the client window responds to events such as clicking on one of the buttons.
The constructor is quite simple - it has three tasks, as shown below:
public NameClient (String title)
{
super(title); setUpGUI( );
connectToServer( );
}
First, it invokes the constructor for its superclass (in this case, JFrame) - this sets the window title. Using two private helper methods, it constructs the graphical interface (as shown in Figure 8) and then connects to the name server, whose code we looked at in Subsection 8.1. Once a client object has been created by executing this constructor, it simply waits for the user to click on one of the buttons before responding. This is what we defined as event-driven programming - the button click events drive the client system.
The detailed code for setting up the GUI is as follows:
// set up client graphical user interface
private void setUpGUI ( )
{
final int CLIENT_WINDOW_WIDTH = 260;
final int CLIENT_WINDOW_HEIGHT = 250;
final int TEXTFIELD_WIDTH = 20;
userName = new TextField(TEXTFIELD_WIDTH);
userEmailAddress = new TextField(TEXTFIELD_WIDTH);
goButton = new JButton("Go");
quitButton = new JButton("Quit");
Container content = getContentPane( );
content.setLayout(new GridLayout(5, 1));
content.add(new Label("User name"));
content.add(userName);
content.add(new Label("Email address"));
content.add(userEmailAddress);
JPanel buttonPanel = new JPanel( );
buttonPanel.add(goButton);
buttonPanel.add(quitButton);
content.add(buttonPanel);
// register button listeners goButton.addActionListener(this);
quitButton.addActionListener(this);
setSize(CLIENT_WINDOW_WIDTH, CLIENT_WINDOW_HEIGHT);
setVisible(true);
}
This follows the standard pattern of creating a number of user interface objects - text fields, buttons and labels - and adding these to the frame using a suitable layout. In this case, the frame layout manager is a simple GridLayout, but other more complex layouts would be possible. Finally, the client frame object is registered as a listener for both the buttons. This means that the NameClient class must provide a method that responds to any button clicks - more details of this are given later in this section.
The code for connecting to the server is in the helper method connectToServer. This creates a socket and then opens the streams for communication with the server:
// set up connection to the server
private void connectToServer ( )
{
try
{
socket = new Socket(SERVER_ADDRESS, SERVER_PORT_NUMBER);
openStreams( );
}
catch (IOException e)
{
System.out.println("Trouble contacting the server " + e);
}
}
The socket that is created here and referenced by the instance variable socket is associated with the address of the server as well as the port number, which must be the same as the port number used in the server code. Either the IP address or the symbolic form of the server address can be used. This method also invokes the openStreams method, which is very similar to the corresponding methods we have seen previously. Any exceptions that occur are handled within the connectToServer method. The code for the method openStreams is as follows:
// open streams for input and output
private void openStreams ( ) throws IOException
{
final boolean AUTO_FLUSH = true;
is = socket.getInputStream( );
fromServer = new BufferedReader(new InputStreamReader(is));
os = socket.getOutputStream( );
toServer = new PrintWriter(os, AUTO_FLUSH);
}
The client code so far has set up the client window and the connection to the server, but we have not yet seen how anything happens. For this, we must look at the actionPerformed method, which is required since the NameClient class implements the ActionListener interface. When one of the buttons is clicked, the actionPerformed method is invoked because the NameClient class is registered as a listener:
// select an action depending on which button was pressed
public void actionPerformed (ActionEvent ae)
{
Object buttonClicked = ae.getSource( );
try
{
if (buttonClicked.equals(goButton))
{
processGo( ); // respond to GO button
}
else if (buttonClicked.equals(quitButton))
{
processQuit( ); // respond to QUIT button
}
}
catch (IOException e)
{
System.out.println("Problem with the server " + e);
}
}
Here, the ActionEvent argument ae allows us to find which object is the source of the event. In this case, the only relevant events are a click on one of the two buttons. If one of the two buttons is clicked, the corresponding method will be invoked to process this.
When the Go button is clicked, the method processGo is invoked. Its code is as follows:
// respond to Go button - User has requested an email address
private void processGo ( ) throws IOException
{
String usName;
String emailAddress;
// get the user name
usName = userName.getText( );
// send it to the server
toServer.println(usName);
// receive reply
emailAddress = fromServer.readLine( );
// display email address
userEmailAddress.setText(emailAddress);
}
Here, the client sends the contents of the userName text field to the server. It then waits for the server to respond with the email address for that user and displays this in the other text field. To keep this example simple, we ignore the possibility that the userName text field may be blank. If this were the case, the server would simply reply with a message that the name had not been found.
Returning to the actionPerformed method, if the Quit button was clicked then the method processQuit would be invoked. The code is as follows:
// respond to Quit button
private void processQuit ( ) throws IOException
{
toServer.println(CLIENT_QUITTING);
closeStreams( ); // close streams to server
socket.close( ); // close socket connection to server
System.exit(0); // close down client program
}
A special message is sent to the server to inform it that the client is terminating the connection. In response to this, we have seen earlier that the server will close the connection to the client. The client then closes the streams from its end using the helper method closeStreams. This is similar to the corresponding method in NameServer, with the code in the NameClient method as follows:
// close streams to and from the server
private void closeStreams ( ) throws IOException
{
toServer.close( );
os.close( );
fromServer.close( );
is.close( );
}
The client code is run by a main method located in the separate class, TestNameClient, as follows:
public class TestNameClient
{
public static void main (String [] args)
{
NameClient client1 = new NameClient("Name Service Client");
}
}
In this case, we simply need to create a NameClient object in order to connect to the server and to set up the GUI. Because the client is driven by events (button clicks) from this point onward, there is no need to invoke any other methods and, in fact, the NameClient class does not offer any public methods. Where we invoked a run method to handle the interaction with the server because there was no GUI.
Before running the client, we need to start the name server. Otherwise, an exception will occur when the client tries to create a socket linked to the server. When the client and server above run successfully, the client will keep running until we click the Quit button, at which point it will close down. We can then start up another client, as the name server should still be running, and continue to interact with the server. In this example, there is no elegant way to close the server; we simply have to end the program by whatever means the environment offers to terminate programs.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your The client code homework and assignments? Live The client code experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer The client code homework help, java assignment help and The client code projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours