The circular buffer:
A buffer is a set of memory locations used to store data which is produced and consumed by a number of programs or, in our case, a number of threads. For example, a buffer is used when data is transferred from a user program to a printer: the user program deposits data that is required to be printed into a buffer and the program operating the printer takes the data out of the buffer - usually on a first-in-first-out (FIFO) basis as in the case below. One popular way of implementing a buffer is as a circular queue. This, of course, is only a logical view of the queue; in practice it is implemented in
Java using an array. An example of such a queue is shown in Figure.
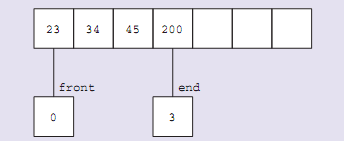
Figure: A circular buffer represented by an array
The circular buffer is represented as a fixed-length array with two instance variables that point to the beginning and end of the buffer. One variable, front, contains the index to the first item in the buffer and the second variable, end, contains the index to the final item in the buffer.
When an item is removed from the buffer the element indexed by front is updated and the value of the front index is incremented by one. When an item is added to the buffer, the end index is incremented and the item is written to the location at which end now points. If the item to be added overfiows the end of the array it is added to the front of the array, provided there is space.
A class describing a circular buffer is shown below. As well as having instance variables for front and end it also has an instance variable numberInBuffer, which contains the current number of items in the buffer, and an instance variable bufferLength, which contains the maximum number of items in the buffer. For the sake of simplicity we shall assume that the buffer contains integers.
public class CircBuffer
{
private int front, end, numberInBuffer, bufferLength;
private int [] buffer;
public CircBuffer (int length)
{
buffer = new int [length];
front = 0;
bufferLength = length; end = -1; numberInBuffer = 0;
}
public CircBuffer ()
{
this(100);
}
public void addItemToBuffer (int i) throws BufferFullException
{
if (numberInBuffer < bufferLength)
{
if (end == bufferLength - 1)
{
end = 0;
}
else
{
end++;
}
buffer [end] = i;
numberInBuffer++;
}
else
{
throw new BufferFullException();
}
}
public int removeItemFromBuffer () throws BufferEmptyException
{
int value;
if (numberInBuffer > 0)
{
value = buffer [front];
if (front == bufferLength - 1)
{
front = 0;
}
else
{
front++;
}
numberInBuffer- -;
return value;
}
else
{
throw new BufferEmptyException();
}
}
public int numberInBuffer ()
{
return numberInBuffer;
}
}
The class contains two constructors: one that allows the buffer length to be set to a specific value, the other that defaults the buffer to 100 locations. The class also throws two exceptions - one when the buffer is full and one when the buffer is empty. The code for the exceptions is given below:
public class BufferEmptyException extends Exception
{
public BufferEmptyEx ()
{
super();
System.out.println ("buffer empty");
}
}
public class BufferFullException extends Exception
{
public BufferFullException ()
{
super();
System.out.println("buffer full");
}
}
This is a good implementation of a buffer in a non-threaded application. However, it is not so good in a threaded environment when there might be a large number of threads adding and removing data to and from the buffer. For example, the buffer might contain packets of data that are being deposited in it by a number of computers that are connected to the computer running the buffer application. The reason that this would not be a good implementation in such an environment is that if the buffer is empty, then the method that retrieves a value from the buffer exits because an exception is thrown. In a threaded environment there may be a number of processes just about to write data to the buffer and what is needed is for the thread that wants to take data out of the buffer to wait for this to happen. Similarly, the method that adds an item to the buffer exits if the buffer is full, even when there may be some threads about to read - that is, take out data from the buffer. Another problem, of course, is that the buffer is a shared resource and some of the methods within the circular buffer class need to be declared as synchronized. A possible solution that implements the two methods that are now able to wait is shown below. Only the changed methods are shown.
public synchronized void addItemToBuffer (int i)
{
while (numberInBuffer == bufferLength)
{
try
{
Thread.sleep(20);
}
catch (InterruptedException e)
{
String errMessage = e.getMessage();
System.out.println("Error " + errMessage);
}
}
if (end == bufferLength - 1)
{
end = 0;
}
else
{
end++;
}
buffer [end] = i;
numberInBuffer++;
}
public synchronized int removeItemFromBuffer ()
{
int value;
while (numberInBuffer == 0)
{
try
{
Thread.sleep(20);
}
catch (InterruptedException e)
{
String errMessage = e.getMessage();
System.out.println("Error " + errMessage);
}
}
value = buffer[front];
if (front == bufferLength - 1)
{
front = 0;
}
else
{
front++;
}
numberInBuffer- -;
return value;
}
In the addItemToBuffer method, the following section of code sends the thread to sleep for 20 milliseconds while the value of numberInBuffer remains equal to bufferLength; that is, the buffer is full.
while (numberInBuffer == bufferLength)
{
try
{
Thread.sleep(20);
}
catch (InterruptedException e)
{
String errMessage = e.getMessage();
System.out.println("Error " + errMessage);
}
}
Similarly, in the removeItemFromBuffer method the following section of code puts the thread to sleep until the buffer contains at least one item:
while (numberInBuffer == 0)
{
try
{
Thread.sleep(20);
}
catch (InterruptedException e)
{
String errMessage = e.getMessage();
System.out.println("Error " + errMessage);
}
}
This looks quite a nice solution to the problem. However, remember that when a thread enters a synchronized method it gains the lock to that object and any other synchronized methods relating to that object are not able to execute. When the thread goes to sleep it does not release that lock but keeps hold of it.
In order to focus on this problem and its implications, let us concentrate on the method that adds items to the buffer. When the code which sends the current thread to sleep is executed we hope that another thread will remove at least one item from the buffer. When this happens and the current thread awakes from its sleep it can continue executing. Unfortunately, no other thread can do anything to the buffer object since its methods are synchronized: only one thread - the one that is sleeping - is allowed access because the sleeping thread still holds the lock. So the solution above does not represent any advance: a thread wanting to add an item to a full buffer cannot do so, even though there may be another thread that is about to remove an item from the buffer.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your The circular buffer homework and assignments? Live The circular buffer experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer The circular buffer homework help, java assignment help and The circular buffer projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours