Sockets on the server:
On the server system we also need a socket for each connection to a client. However, creation of sockets must be approached a little differently. This is because the server does not normally initiate a connection - rather, it waits for a client to request a connection. Hence it cannot create a socket until it knows the address of the client that wants to establish a connection.
The key class for dealing with this is the ServerSocket class. This is used within a server for setting up sockets that are to be associated with a client. The class has two important constructors. The first has the form:
ServerSocket(int)
This sets up a ServerSocket object associated with a particular port specified by its single argument. As you will see below, this object is then used to create the sockets that are associated with clients communicating with the server via that port. For example, the following code causes the server to 'listen' on port 80 (normally used for HTTP requests).
ServerSocket ss = new ServerSocket(80);
In practice, this means that the server checks incoming TCP packets to see whether they are addressed to port 80.
The second constructor is defined by:
ServerSocket(int, int)
The first argument is the port to be used, as before. The second argument is the maximum number of clients allowed to wait for connections from the server. For example, the code:
ServerSocket ss = new ServerSocket(3000, 30);
sets up the ServerSocket object referenced by ss, which is associated with port 3000 and with a client queue of maximum length 30. If, while processing a request from a client, another client attempts to access the service via this port, then the new client connection request will be placed in the queue and will be dealt with after the first client's connection has terminated. If the queue is full, any further client connections will be refused.
Once a ServerSocket object is created, the server will wait for clients to request a connection. When a connection is made, a socket linked to the client is created; this is achieved via the accept method.
An example of its use is shown below:
// server code
ServerSocket ss = new ServerSocket(3000, 30);
...
// wait until connection request made by client
Socket sock = ss.accept( );
When the accept method is first executed, it is blocked: it stops running until a client attempts to create a socket on the port associated with the ServerSocket object. The accept method creates a socket that links to the client requesting the service provided on port 3000 by the server. This socket can then be used to access input and output streams in the same way as we saw above on the client.
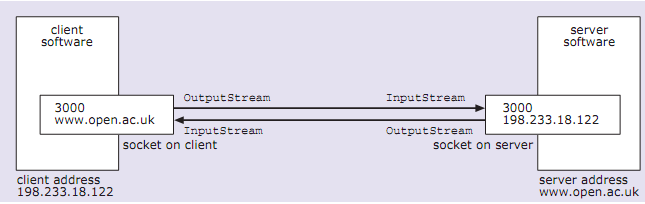
In programming servers and clients you should bear in mind that:
- a connection is a linked pair of sockets;
- at the server end, the socket has the address of the client and a suitable port number for the service required by the client;
- at the client end, the socket has the address of the server and the same port number as the server for the particular service required;
- the InputStream entering the client receives data from the OutputStream leaving the server and the InputStream entering the server receives data from the OutputStream leaving the client.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your Sockets on the server homework and assignments? Live Sockets on the server experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer Sockets on the server homework help, java assignment help and Sockets on the server projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours