Object wrapper classes:
In some (so-called pure) object-oriented languages everything is an object, including the equivalents of the primitive data types in Java - integers, characters, floating-point numbers, and so on. In Java, mainly for efficiency reasons, the language designers decided to treat primitive data types in the way we have explained: that is, they store actual values.
To bridge this gap, Java 1.4 employed object wrappers, which 'wrap around' or encapsulate all the primitive data types and allow them to be treated as objects. There is an object wrapper class for each primitive data type, as summarized in Table. Note that the class names are not entirely consistent - the compiler will remind you if you get mixed up!
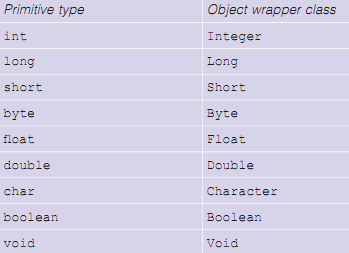
Object wrapper classes
The object wrapper for int is the class Integer. It contains constructors for creating an Integer object from an int or from a String. It also has methods to return the value of the Integer object as a String, as an int, or as various other primitive data types such as fioat or short. So, for example, you could have a collection:
ArrayList<Integer>intlist = new ArrayList<Integer>();
In Java 1.4 we would have written:
intlist.add(new Integer(3));
In Java 1.5 we can deposit Integer objects directly into a collection and also remove them directly. For example, the code below places the Integer 3 into an ArrayList object, retrieves it, then adds 2 to it and displays the result (5):
ArrayList <Integer> holder =
new ArrayList <Integer>(); holder.add(3); System.out.println(holder.remove(0)+2);
An interesting part of this code is the final line where we retrieve the Integer that was stored and then add 2 to it. This seems an incorrect thing to do since, with the exception of the + operator that is used to concatenate strings, operators such as *, -, ++ can be applied only to basic types such as int.
What actually happens in the final line is that the code:
holder.remove(0)
returns an Integer object, which is then automatically converted to an int and the + operator applied. The process of converting an object wrapper to its corresponding primitive type is known as unboxing, and was introduced in Java 1.5.
Another example of unboxing is shown below, this time using the Character object wrapper:
ArrayList <Character> holder =
new ArrayList <Character>();
holder.add('Z');
System.out.println("The answer is "+ holder.remove(0));
Here the character 'Z' is retrieved and then concatenated with the string "The answer is "; the result is then displayed as:
The answer is Z
From Java 1.5, it is no longer necessary to manually wrap primitives, as we now have
autoboxing, which does this for us.
Collections such as the ArrayList collection can be sequentially accessed via a special type of for statement known as the for-each statement. This iterates over the objects contained in a collection and extracts each of them. An example of this statement in action is shown below.
ArrayList <Character> holder =
new ArrayList <Character>();
holder.add('a'); holder.add('b'); holder.add('c');
for (Character i : holder) System.out.println("The value is "+ i);
Here three characters are added to the ArrayList object holder. The for statement then iterates, extracting out each of the stored Character objects, unboxes them and concatenates them with the string "The value is" before displaying the concatenated string that is formed. The general form of this for statement is:
for (Classname element_identifier: collection_identifier)
The Classname gives the type of the stored object, element_identifier is given the values of the object and collection_identifier identifies the collection that is traversed. An example is shown below:
for(Employees empl: empColl)
{
...
if (empl.pay()>100000)
{
System.out.println(empl);
}
}
Here the collection empColl that contains employee objects is traversed, and each element retrieved and assigned to the variable empl. Each empl then invokes pay(), which extracts the annual salary of the employee. This is then compared with 100000 and any employee who earns more than a hundred thousand pounds is displayed.
We have shown you how the ArrayList class can store references and wrapped primitives. We have also emphasized the importance of using the Java API documentation. Next, we describe another important Java collection class, namely HashMap.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your Object wrapper classes homework and assignments? Live Object wrapper classes experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer Object wrapper classes homework help, java assignment help and Object wrapper classes projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours