Moving ball
The class MovingBall and its associated class Ball, as shown below, create a very simple moving image. A red ball is created at some random point on the screen and it then falls at a constant speed down the screen. In the next section, we shall see how this can be developed into a simple game.
We have taken a prototyping approach of using the paint method in the JFrame rather than the more extensible approach of painting in a JPanel that is added to the JFrame.
import java.awt. *; import javax.swing. *; import java.awt.event. *;
public class MovingBall extends JFrame
{
protected final int FRAME_WIDTH = 240;
protected final int FRAME_HEIGHT = 320;
private Ball myBall = new Ball(FRAME_WIDTH, FRAME_HEIGHT);
public MovingBall (String title)
{
super(title);
setSize(FRAME_WIDTH, FRAME_HEIGHT);
addWindowListener(new CloseAndQuit( ));
}
public void paint (Graphics g)
{
super.paint(g);
myBall.paint(g);
}
public void move ( )
{
while (true)
{
myBall.move( );
repaint( );
try
{
Thread.sleep(50);
}
catch (InterruptedException e)
{
System.exit(0);
}
}
}
}
public class MovingBallTest
{
public static void main (String [] args)
{
MovingBall world = new MovingBall("Moving Ball");
world.setVisible(true);
world.move( );
}
}
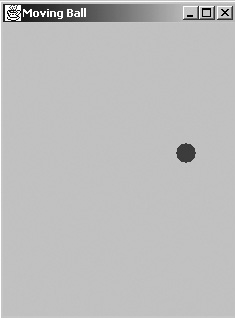
Figure A MovingBall frame showing a ball
The class MovingBall sets up a frame of 240 by 320 pixels, which is a typical PDA sized screen. It then repeatedly calls the method move, which in turn calls the method move of the class Ball that we shall look at shortly. After this, the method repaint is called. Note that we have not defined a method repaint, but it is inherited from Component. The repaint method calls the paint method, which we have defined but cannot call directly. This approach is part of the Java system to ensure that components are drawn in the correct order. In paint we tell the system what to paint; by calling repaint, we indicate that we wish this event to take place.
What is actually specified in the paint method is a call to super.paint(), which in effect clears the screen and redraws the background. You may wish to try taking out the call to super; you will see that the moving ball becomes a smear because the previous drawings of the ball are not being cleared. We then call the paint method of Ball.
In the move method, there is a try-catch block and the code Thread.sleep(50). The sleep method of the Thread class suspends the program for a period of time given in milliseconds. While the program sleeps, the system has time to finish the time consuming process of repainting the window. You will learn more about the Thread class in a later unit. Again, try taking out the try-catch block. This time you will see that the ball is always at the bottom of the frame because the system has not had time to repaint the screen before the ball reaches the bottom.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your Moving Ball homework and assignments? Live Moving Ball experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer Moving Ball homework help, java assignment help and Moving Ball projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours