Mouse clicker:
The first example displays a panel. When the user clicks at a point in the panel a message is displayed. The user can switch the colour in which the message is displayed by clicking a radio button. In Figure, we see the radio buttons (the blue button has been clicked) and the result of a number of clicks on the panel in the top half of the screen. The text displayed is the string 'Hello there'.
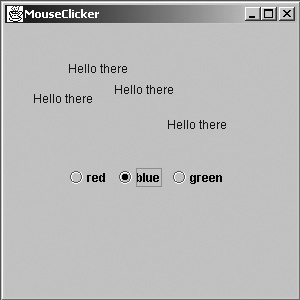
Figure The MouseClicker frame showing the text Hello there in the chosen colour in a variety of positions on the screen
The main class for this application is shown below:
public class MouseClickerTest
{
public static void main (String [] args)
{
MouseClicker world = new MouseClicker( );
world.setVisible(true);
}
}
// the MouseClicker class
import java.awt. *;
import javax.swing. *;
import java.awt.event. *;
public class MouseClicker extends JFrame
{
private Color currentColour;
private JRadioButton redButton;
private JRadioButton blueButton;
private JRadioButton greenButton;
private JPanel bottom;
private ClickablePanel top;
public MouseClicker( )
{
setTitle("MouseClicker");
setSize(300, 300);
ButtonGroup colour = new ButtonGroup( );
bottom = new JPanel( );
top = new ClickablePanel( );
redButton = new JRadioButton("red", true);
bottom.add(redButton);
blueButton = new JRadioButton("blue", false);
bottom.add(blueButton);
greenButton = new JRadioButton("green", false);
bottom.add(greenButton);
// now add the radio buttons to the button group
colour.add(redButton);
colour.add(blueButton);
colour.add(greenButton);
getContentPane( ).setLayout(new GridLayout(2, 1));
getContentPane( ).add(top);
getContentPane( ).add(bottom);
redButton.addItemListener(new ButtonWatcher( ));
blueButton.addItemListener(new ButtonWatcher( ));
greenButton.addItemListener(new ButtonWatcher( ));
addWindowListener(new CloseAndQuit( ));
} // ends constructor
}
In MouseClicker we have declared two panel variables, one a JPanel and the other a ClickablePanel, which we will define later. We have created a radio button group and attached an ItemListener to each button. This ItemListener is defined below as an inner class. The radio buttons have been placed on the bottom panel and both panels have been added to the frame. The code also declares currentColour, which will reference the current colour in which text is displayed on the panel.
The next code segment is that for handling the event of a radio button being checked. The code is shown below:
private class ButtonWatcher implements ItemListener
{
public void itemStateChanged (ItemEvent i)
{
if (redButton.isSelected( ))
{
currentColour = Color.red;
}
if (blueButton.isSelected( ))
{
currentColour = Color.blue;
}
if (greenButton.isSelected( ))
{
currentColour = Color.green;
}
top.setClickColour(currentColour);
} // ends method itemStateChanged
} // ends class ButtonWatcher
The ItemListener interface has a method itemStateChanged, and here we have provided the implementation for it. Since the constructor has added an instance of ButtonWatcher to each of the radio buttons, this code is executed when any of these buttons is clicked. The method isSelected returns true if the radio button is in the 'on' position (for example, the blue button in Figure 6).
All that is now needed is to program the ClickablePanel class. The first lines of this class are shown below:
import java.awt. *;
import javax.swing. *;
import java.awt.event. *;
public class ClickablePanel extends JPanel
{
private int [] xVals,yVals;
private int numberOfClicks;
private Color colour;
private Clicker cl;
This class inherits from JPanel. It contains instance variables that hold the current colour used for writing the strings, the number of clicks that have occurred within this component, and two arrays that hold the x- and y-values of each click that has occurred. It also holds an instance variable for the instance of the inner class Clicker, which is used to track mouse clicks.
The constructor of the ClickablePanel class is shown below. It sets up the panel, initializes the number of clicks, sets the first colour to red (Color.red) and allocates memory for the arrays. It also registers the instance variable cl as a mouse listener, which is a very important statement. It is this line of code that turns an ordinary panel into a clickable panel because it now has the capability to listen to mouse clicks.
public ClickablePanel( )
{
super( );
cl = new Clicker( );
addMouseListener(cl);
colour = Color.red;
xVals = new int [100 ];
yVals = new int [100 ];
numberOfClicks = 0;
}
Two further methods of the ClickablePanel class are shown below:
public void setClickColour (Color c)
{
colour = c;
}
public void paintComponent (Graphics g)
{
super.paintComponent(g);
g.setColor(colour);
for (int i = 0; i < numberOfClicks; i++)
{
g.drawString("Hello there", xVals [i ], yVals [i ]);
}
}
The first method, setClickColour, is used in the ButtonWatcher class and simply sets the current colour. The next method overrides the default paintComponent method supplied with the JPanel class. This method is the JPanel analogue of the paint method of JFrame. The paintComponent method sets the colour for drawing to the current colour and then draws all the instances of the string "Hello there" on the panel. The code uses the methods drawString and setColor provided as part of the Color class in the
java.awt package.
The inner class is shown below:
private class Clicker extends MouseAdapter
{
public void mouseClicked (MouseEvent m)
{
xVals [numberOfClicks ] = m.getX( );
yVals [numberOfClicks ] = m.getY( );
numberOfClicks++;
repaint( );
}
} // ends inner class Clicker
} // ends class ClickablePanel
This, then, is the code for our application. The code for ClickablePanel keeps track of the past mouse clicks and refreshes the screen with all the text that needs to be displayed. It does this by calling paintComponent via the repaint method when a relevant event occurs.
An important point to make about the code above is that some of it is vestigial but it has been placed there in order to clarify the mechanisms that are involved in using inner classes.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your Mouse clicker homework and assignments? Live Mouse clicker experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer Mouse clicker homework help, java assignment help and Mouse clicker projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours