Event programming:
The remaining code within MyFrame is simple: it counts the number of times that the button is clicked, displays the count in a label and resets the count back to zero when it reaches ten.
But notice that there is something quite remarkable happening in this program, something that we have not seen before. Every time that the user clicks the button, the code is executed no matter how many times they click. Yet there are no loops programmed in the code. To get this type of repetitive behaviour up to now you would have had to include some sort of a loop construct statement to get the program to cycle over and over again. However, with event-driven programming this is not needed. The above code has defined (in the inner class), created (using new ButtonWatcher) and attached (addActionListener) a listener to the button. This listener simply sits there waiting to 'fire' when 'its' event happens on 'its' component.
There are some final points worth making about the above code. First, it needs to import javax.swing for the visual object classes; second, it needs to import java.awt. event for the event classes and interfaces; third, although the actionPerformed method has a single ActionEvent argument a, it is not used within the code. This argument contains subsidiary information about the event, which can be used for more complicated processing.
The event argument
The next example shows the use of the event argument.
import javax.swing. *; import java.awt.event. *; import java.awt. *;
public class MySecondFrame extends JFrame
{
private int buttonClicks;
private JButton buttonUp, buttonDown;
private JLabel label;
public MySecondFrame (String s)
{
super(s); setSize(300, 300); buttonClicks = 0;
buttonUp = new JButton("Press Me Up");
buttonDown = new JButton("Press Me Down");
label = new JLabel("Starting");
buttonUp.addActionListener(new ButtonWatcher( ));
buttonDown.addActionListener(new ButtonWatcher( ));
getContentPane( ).setLayout(new FlowLayout( ));
getContentPane( ).add(buttonUp);
getContentPane( ).add(buttonDown);
getContentPane( ).add(label);
}
/ * Define the listener class by implementing the interface ActionListener. */
private class ButtonWatcher implements ActionListener
{
/ * Here is the code which is executed when the button is clicked. */
public void actionPerformed (ActionEvent a)
{
Object buttonPressed = a.getSource( );
if (buttonPressed.equals(buttonUp))
{
buttonClicks++;
}
if (buttonPressed.equals(buttonDown))
{
buttonClicks--;
}
label.setText(buttonClicks + "");
}
}
}
public class MySecondFrameTest
{
public static void main (String [] args)
{
MySecondFrame world = new MySecondFrame("Two Buttons");
world.setVisible(true);
}
}
In this example, there are two buttons. The first button increments the integer displayed in the label and the second decrements the integer. Both buttons have the same listener (ButtonWatcher) attached, so when actionPerformed is executed there is a need to determine the source of the event. This is done by invoking the method getSource. This method returns an object and, using the equals method of the Object class, the specific button pressed can be identified and the appropriate code executed. Figure 2 shows the interface after a number of clicks.
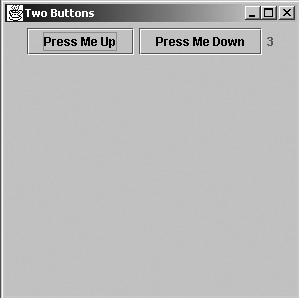
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your Event programming homework and assignments? Live Event programming experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer Event programming homework help, java assignment help and Event programming projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours