Defining Sprites:
Defining a Sprite is easier than defining a TiledLayer. We still begin with an image object though, and it is still broken up into equal-sized subunits. However, for a Sprite these are not tiles but animation frames.
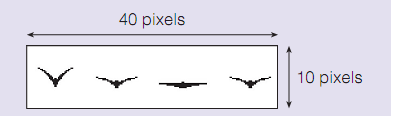
Figure: Animation frames
Assuming we've already used the image above to create an Image object referenced by birdImage, the constructor call:
Sprite birdSprite = new Sprite(birdImage, 10, 10);
will result in a Sprite object with 4 animation frames, each 10 6 10 pixels. To animate the object, we repeatedly call:
birdSprite.nextFrame();
This causes the successive frames to be shown one after another like a film loop, causing the illusion of the bird fiapping its wings. When the last frame is reached the animation will return automatically to the start of the sequence.
Movement
Once a Sprite has been constructed and placed in the game it can be moved very easily by calling move(int dx, int dy). For example:
birdSprite.move(10, -20);
will move the bird 10 pixels right and 20 up from its current position.
Collision detection
In a game we often need to know if two things have collided with one another. The method collidesWith makes it straightforward to find out if a Sprite has hit something else. For example, if we wanted to respond if our bird had fiown into another bird, birdSprite2, we would use something like:
if (birdSprite.collidesWith(birdSprite2, false))
{// take appropriate action}
The second argument to the method indicates how we want the collision checked. Every Sprite is enclosed in a collision rectangle. By default this is just the bounding rectangle of the Sprite, although it can be resized and/or shifted at will using the defineCollisonRectangle method.
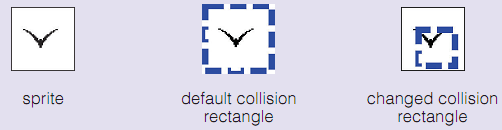
Figure: The collision rectangle
If the second argument to collidesWith is false, the software simply checks for a collision using the collision rectangle. If the argument is true a more complex scheme is used, which checks whether opaque pixels overlap; roughly speaking this means the boundary of the actual shape is used rather than the collision rectangle.
Rotations and refiections
Methods also exist for rotating sprites through a multiple of 90 degrees and for creating mirror images of them. This often lets us get away with a smaller number of animation frames than would be needed otherwise. For example, if we want to show a sprite moving forwards and backwards we just create a forward set of frames, then use the same sequence with a mirror transform to produce the backwards movement.
We don't use transforms in our game, so we won't take more space describing them here, but if you are interested you can find out more from the Games API documentation.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your Defining Sprites homework and assignments? Live Defining Sprites experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer Defining Sprites homework help, java assignment help and Defining Sprites projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours