Capturing an event from a button:
We will start by looking at the intuitively simple example of a button being clicked. This also turns out to be one of the simplest events to program.
Although there are many events to which you might want a button to respond (such as the mouse passing over it), the main event that you are likely to be interested in is that of the button being clicked by the user. This means that we need to create an object that will sit around waiting for the particular button to be pressed. This object will contain the code that we wish to run when the button is pressed.
To achieve this, we use the ActionListener interface. All of the listeners for the various events are in the form of interfaces. The ActionListener interface corresponds to a number of events that are generated by visual objects. They include a button being clicked, an item being selected from a list box, a menu item being selected and the Enter key being pressed in a text field.
As ActionListener is an interface, we need to define a class that implements all of the methods in the interface in order to create a usable object. With the ActionListener interface, there is only one method to be implemented: the actionPerformed method. This method is the one that will be executed when an event such as a button being clicked occurs.
However, with some listeners there are many methods and we will see later how we can avoid the need to write code for many methods, most of which we might not want to use.
Once we have created our ActionListener object, we need to register it with the component to which we want it to 'listen' - in this case, a button. This is achieved using the addActionListener method. All components have methods to add and remove listeners from themselves, and the method names follow a fairly simple convention of add (or remove) followed by the event followed by Listener. You will see more examples of this later.
The following code shows the above points.
import java.awt.event. *;
import javax.swing. *;
public class MyFrame extends JFrame
{
private int buttonClicks;
private JButton button;
private JLabel output;
public MyFrame (String s)
{
super(s); setSize(300, 300);
buttonClicks = 0;
button = new JButton("Press Me");
output = new JLabel("Starting");
getContentPane( ).add(button, "West");
getContentPane( ).add(output, "East");
/ * Create an instance of ButtonWatcher and register this object listener with the button. */
button.addActionListener(new ButtonWatcher( ));
}
/ * Define the listener class by implementing the interface ActionListener. */
private class ButtonWatcher implements ActionListener
{
/ * Here is the code which is executed when the button is clicked. */
public void actionPerformed (ActionEvent a)
{
buttonClicks++;
output.setText("" + buttonClicks);
if (buttonClicks == 10)
{
buttonClicks = 0;
}
}
}
}
public class MyFrameTest
{
public static void main (String [] args)
{
MyFrame world = new MyFrame("Button Watcher");
world.setVisible(true);
}
}
Read through this code carefully, as there are many interesting features about it. We have created a button and a label, placed them in a frame and displayed them through the main method. Figure shows the screen after a number of button clicks.
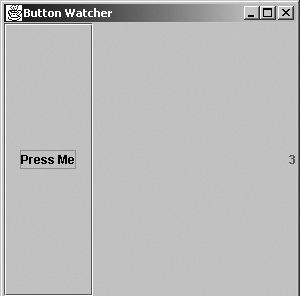
In order to link the button with some code, we have defined a class of listener called ButtonWatcher (it watches our button for events). In the constructor for MyFrame we create an instance of ButtonWatcher using new and then register it with the button to which we want it to listen, using the addActionListener method. All this takes place in a single line:
button.addActionListener(new ButtonWatcher( ));
The code to define this listener is reproduced below:
/ * Define the listener class by implementing the interface ActionListener. */
private class ButtonWatcher implements ActionListener
{
/ * Here is the code which is executed when the button is clicked. */
public void actionPerformed (ActionEvent a)
{
buttonClicks++;
output.setText("" + buttonClicks);
if (buttonClicks == 10)
{
buttonClicks = 0;
}
}
}
The biggest surprise that you will have had is the fact that this is the definition of a class, ButtonWatcher, inside the definition of another class, MyFrame. We say that ButtonWatcher is an example of an inner class.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your Capturing an event from a button homework and assignments? Live Capturing an event from a button experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer Capturing an event from a button homework help, java assignment help and Capturing an event from a button projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours