Canvas:
Low level interfaces are based on the Canvas class, which we met earlier - it is an abstract subclass of Displayable. So when we make use of Canvas we have to extend it and implement the paint method, which acts in the same way as in J2SE. Also, as with J2SE, we do not invoke paint directly but instead invoke repaint. The class Canvas also has a number of concrete methods that allow the MIDlet to find out about the physical nature of the display device on which it is running. We will focus on two of these methods:
public int getWidth( )
which returns the screen width in pixels and
public int getHeight( )
which returns the screen height in pixels. Other methods are available that can determine whether the display supports colour, pointing devices, and so on.
As an example of using low level interfaces we will look at a simple MIDlet in which a circle is drawn on the screen and then by using the multidirectional arrow keys, the circle can be moved around the screen. The MIDlet is composed of two classes. The first one, SimpleGraphics, has the basic MIDlet structure and creates an instance of MyCanvas and makes use of the constructor to show that to a large extent much code can go into either the constructor or the startApp method. The startApp method then uses the instance of MyCanvas as the current screen to be displayed.
import javax.microedition.midlet. *;
import javax.microedition.lcdui. *;
public class SimpleGraphics extends MIDlet
{
private Display display;
private MyCanvas canvas;
public SimpleGraphics ( )
{
display = Display.getDisplay(this);
canvas = new MyCanvas( );
}
public void startApp ( )
{
display.setCurrent(canvas);
}
public void pauseApp ( )
{
}
public void destroyApp (boolean b)
{
}
}
The functionality of the MIDlet resides largely in the MyCanvas class.
import javax.microedition.midlet.*;
import javax.microedition.lcdui.*;
public class MyCanvas extends Canvas
{
private int width, height, widthPosition, heightPosition;
public MyCanvas ()
{
width = getWidth();
height = getHeight();
widthPosition = width/3;
heightPosition = height/3;
}
public void paint (Graphics g)
{
// clear screen
g.setColor(0xffffff);
g.fillRect(0, 0, width, height);
// draw circle
g.setColor(0);
g.drawArc(widthPosition, heightPosition, width/4, height/4, 0, 360);
}
protected void keyPressed (int keyCode)
{
if (getGameAction(keyCode) == Canvas.UP)
{
heightPosition = heightPosition - 1;
repaint();
}
if (getGameAction(keyCode) == Canvas.DOWN)
{
heightPosition = heightPosition + 1;
repaint();
}
if (getGameAction(keyCode) == Canvas.LEFT)
{
widthPosition = widthPosition - 1;
repaint();
}
if (getGameAction(keyCode) == Canvas.RIGHT)
{
widthPosition = widthPosition + 1;
repaint();
}
}
}
The structure of this class is the same as that of any standard class in J2SE. We have a constructor handling initializations and we override the paint method as we do in J2SE. First, let us consider the paint method shown above.
This simple paint method clears the screen by filling in a screen-sized rectangle with white. It then draws a circle using the drawArc method. This takes six arguments, the first two representing the top left-hand corner of a box within which the circle will be drawn. The next two arguments are the width and height of the arc, which are equal since this is a circle. The final two arguments give the angle in degrees through which the arc should be drawn. In this case, it goes from zero degrees to 360 degrees and so forms a complete circle. Figure shows the initial screen display.
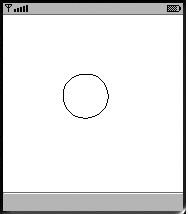
Figure: SimpleGraphics display
Of more interest is the ability for the user to interact with the graphical display. This is achieved by making use of other methods from the Canvas class. Due to the wide range of devices a MIDlet might run on, a developer is quite limited in the keys that can be assumed to be present on a mobile phone. The only keys that are guaranteed to be present are the numbers 0 to 9, the # and * keys. Each of these is assigned a standard key code. However, on our emulator we have a multidirectional key as well and we have made use of this here.
Below is the keyPressed method. This method registers when a user has pressed a key. There are two other similar methods:
protected void keyReleased (int keyCode);
and
protected void keyRepeated (int keyCode);
The method keyPressed is invoked when the user presses a key. To determine which key has been pressed, we use another method from the Canvas class called getGameAction. This converts the key code generated by the key press into one of the actions corresponding to the multidirectional key. Of course, if the device does not have this multidirectional key then there will be no interaction. For devices on which there is a multidirectional key there are standard codes: UP, DOWN, LEFT and RIGHT. These are then used to suitably change the values of heightPosition and widthPosition and then to call repaint to have the circle drawn again with different coordinates from the top left-hand corner of the enclosing box.
protected void keyPressed (int keyCode)
{
if (getGameAction(keyCode) == Canvas.UP)
{
heightPosition = heightPosition - 1;
repaint( );
}
if (getGameAction(keyCode) == Canvas.DOWN)
{
heightPosition = heightPosition + 1;
repaint( );
}
if (getGameAction(keyCode) == Canvas.LEFT)
{
widthPosition = widthPosition - 1;
repaint( );
}
if (getGameAction(keyCode) == Canvas.RIGHT)
{
widthPosition = widthPosition + 1;
repaint( );
}
}
After a few key presses a typical screen is as shown in Figure.
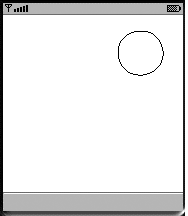
Figure: SimpleGraphics display after several key presses
There is, of course, a great deal more that can be done graphically. However, we hope that we have given you a fiavour of the potential of low level interfaces.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your Canvas homework and assignments? Live Canvas experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer Canvas homework help, java assignment help and Canvas projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours