CAD package in Java:
The next example is of an application that carries out the drawing of many-sided figures using a preselected colour and a preselected style so that the figure can either be an outline or be filled in. The user can draw many polygons in a variety of colours and styles on the same 'canvas'.
An example display for this application is shown in Figure.
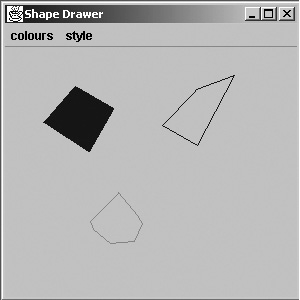
Figure: An example of ShapeDrawer showing two outline shapes and a filled shape
Colour and style are selected using drop-down menus. A panel is used to draw n-sided figures using a mouse. The first time the user clicks the mouse determines the starting location of the first side of the polygon. Any subsequent clicks will determine the lengths of the other sides.
When the mouse clicks on the first position of the polygon again, the polygon is complete and the current colour and style are applied to it. The first lines of the application are shown below:
import javax.swing. *;
import java.awt.event. *;
import java.awt. *;
public class ShapeDrawer extends JFrame
{
private final int FRAME_HEIGHT = 300;
private final int FRAME_WIDTH = 300;
private final String [] colourChoices = {"red", "blue", "green" };
private final Color [] colChoices ={Color.red, Color.blue, Color.green };
private final String []drawChoices = { "outline", "filled" };
private JMenuBar bar;
private JMenu colours;
private JMenu style;
private JMenuItem [] col = new JMenuItem [colourChoices.length ];
private JMenuItem [] sty = new JMenuItem [drawChoices.length ];
private Color defaultcol = Color.red;
private String defaultsty = "filled";
private PaintCanvas canvas;
The above code sets up a PaintCanvas object (which is defined later) and a JMenuBar with two sets of drop-down menu items.
Below is the constructor that puts all of the pieces together. The JMenuItems are given their values, added to the JMenus and then set in a JMenuBar, which is added to the frame along with the PaintCanvas.
public ShapeDrawer (String title)
{
super(title);
setSize(FRAME_WIDTH, FRAME_HEIGHT);
bar = new JMenuBar( );
setJMenuBar(bar);
canvas = new PaintCanvas(defaultcol, defaultsty);
getContentPane( ).add(canvas);
colours = new JMenu("colours");
for (int i=0; i < colourChoices.length; i++)
{
col [i ] = new JMenuItem(colourChoices [i ]);
col [i ].addActionListener(new MenuSelection( ));
colours.add(col [i ]);
}
style = new JMenu("style");
for (int i=0; i < drawChoices.length; i++)
{
sty [i ] = new JMenuItem(drawChoices [i ]);
sty [i ].addActionListener(new MenuSelection( ));
style.add(sty [i ]);
}
bar.add(colours);
bar.add(style);
addWindowListener(new CloseAndQuit( ));
}
We have now created the graphical user interface but, of course, nothing happens when you make a selection from the menus. Below is an inner class that listens for menu items being selected and then uses the choices to set the colour and the style of the drawing in the PaintCanvas object.
private class MenuSelection implements ActionListener
{
public void actionPerformed (ActionEvent e)
{
for (int i = 0; i < colourChoices.length; i++)
{
if (e.getSource( ) == col [i ])
{
canvas.setColour(colChoices [i ]);
}
}
for (int i = 0; i < drawChoices.length; i++)
{
if (e.getSource( ) == sty [i ])
{
canvas.setStyle(drawChoices [i ]);
}
} // ends for
} // ends method actionPerformed
} // ends inner class MenuSelection
} // ends class ShapeDrawer
This ends the ShapeDrawer class. Note that the ShapeDrawer class makes use of a class called PaintCanvas that does all of the drawing work. The class extends JPanel and has a number of arrays that store the colours, styles and x- and y-coordinates (that define the shape and size of each polygon) for up to 100 polygons.
Each polygon can have up to 50 (x, y) coordinate pairs, which we will refer to as points, even though the Point class is not used in this example.
import javax.swing. *;
import java.awt.event. *;
import java.awt. *;
public class PaintCanvas extends JPanel
{
private Clicker cc;
private Color [] polyColours;
private String [] polyStyle;
private Polygon [] polyStore;
private int numberOfPoints;
private int polyCount;
private Color currentColour;
private int []yPoints;
private int [] xPoints;
private String currentStyle;
public PaintCanvas (Color c, String sty)
{
super( ); currentColour = c;
currentStyle = sty;
polyCount = 0;
numberOfPoints = 0;
polyColours = new Color [100 ];
polyStyle = new String [100 ];
polyStore = new Polygon [100 ];
xPoints = new int [50 ];
yPoints = new int [50 ];
cc = new Clicker(this);
addMouseListener(cc);
}
The mouse is used to indicate where the points of each polygon are located. The class PaintCanvas has an inner class, Clicker, which records the coordinates of each click and repaints the panel when there is more than one point. If a point comes close enough to the first point, it is assumed that the user intended that to be the end of the polygon. This allows for the user not being able to click precisely on the original spot. How close exactly is decided by the method isCloseEnough (belonging to class PaintCanvas), which uses Pythagoras' theorem to see whether the current point and the first point are within an arbitrary 10 pixels of each other. If they are, then the arrays holding the x- and y-coordinates are used to create a new Polygon, which is stored in an array of polygons.
private class Clicker extends MouseAdapter
{
private PaintCanvas tpc;
public Clicker (PaintCanvas pc)
{
tpc = pc;
}
public void mouseClicked (MouseEvent e)
{
int xVal = e.getX( ), yVal = e.getY( );
xPoints [numberOfPoints ] = xVal;
yPoints [numberOfPoints ] = yVal;
numberOfPoints++;
if (numberOfPoints > 1)
{
tpc.repaint( );
}
if (numberOfPoints > 2 && isCloseEnough( ))
{
polyStore [polyCount ] = new
Polygon(xPoints, yPoints, numberOfPoints);
polyColours [polyCount ] = currentColour;
polyStyle [polyCount ] = currentStyle;
polyCount++;
numberOfPoints = 0;
tpc.repaint( );
} // ends if
} // ends method mouseClicked
} // ends inner class Clicker
The code for methods isCloseEnough, setColour and setStyle are given below. The isCloseEnough method uses the methods pow and sqrt, which are static methods in the Math class in the java.lang library. The pow method takes two arguments, the first a double value that is raised to the power indicated by the second (for squaring this is 2.0). The sqrt method returns the square root of its argument's value. Notice the use of the (double) cast, which makes sure that the integer values within the arrays holding the polygon points are of the right type for these two methods.
public boolean isCloseEnough ( )
{
double xLength = (double)(xPoints [0] - xPoints [numberOfPoints - 1 ]);
double yLength = (double)(yPoints [0] - yPoints [numberOfPoints - 1 ]);
double hypot = Math.sqrt(Math.pow(xLength, 2.0) + Math.pow(yLength, 2.0));
if (hypot < 10)
{
return true;
}
else
{
return false;
}
}
public void setColour (Color c)
{
currentColour = c;
}
public void setStyle (String s)
{
currentStyle = s;
}
The final method of PaintCanvas class we now discuss is paintComponent. This method is called whenever the program calls repaint. In a sense, the program is inefficient in that it redraws everything every time. The first for loop draws the completed polygons in either the filled or the outline style. The second for loop draws the current polygon as it is being created by the user.
public void paintComponent (Graphics g)
{
super.paintComponent(g);
for (int i = 0; i < polyCount; i++)
{
g.setColor(polyColours [i ]);
if (polyStyle [i ].equals("filled"))
{
g.fillPolygon(polyStore [i ]);
}
else
{
g.drawPolygon(polyStore [i ]);
}
}
g.setColor(currentColour);
for (int j = 0; j < numberOfPoints - 1; j++)
{
g.drawLine(xPoints [j ], yPoints [j ], xPoints [j + 1 ], yPoints [j + 1 ]);
}
} // ends method paintComponent
) // ends class PaintCanvas
The method isCloseEnough in class PaintCanvas makes use of Pythagoras' theorem to see whether the current point and the first point are within an arbitrary 10 pixels of each other. However, the code for isCloseEnough is quite dense and so to help you follow it we will now give an example to draw out the mathematics.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your CAD package in Java homework and assignments? Live CAD package in Java experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer CAD package in Java homework help, java assignment help and CAD package in Java projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours