An applet example:
In this subsection, we look at a more substantial example of an applet to illustrate the use of some of the methods discussed above.
This example is a simple game we call 'Spot the Robot'. This involves a 'magic robot' that can disappear and reappear on the screen in any position. The game requires the user to click, using the mouse, to indicate the position where they think the magic robot will next appear.
For this example, we use an applet class, SpotTheRobot, which extends the Applet class, rather than the JApplet class that we used in the previous example. This should allow the applet to run on a wider variety of browsers. We do not require any of the more advanced features offered by JApplet, such as the Swing libraries, so there is no disadvantage in doing this.
Figure contains a typical view of the applet window as shown by the appletviewer.The code for the applet is as follows:
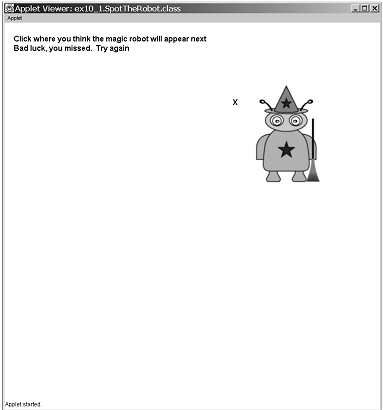
import java.awt.*;
import java.awt.event.*; import java.applet.*; import java.util.*; import java.net.*;
public class SpotTheRobot extends Applet
{
private Image magicRobotImage;
private final int WINDOW_X_SIZE = 800; private final int WINDOW_Y_SIZE = 800; private Font messageFont;
private Random random;
private int mouseXPosition;
private int mouseYPosition;
private boolean starting;
// initialize the applet
public void init ()
{
/* Load the magic robot image. Assume that the HTML file for this applet is stored in the project base folder and that the image is
stored in subfolder 'images' of this base folder.*/
magicRobotImage = getImage (getCodeBase (), "images/MagicRobot.jpg");
resize (WINDOW_X_SIZE, WINDOW_Y_SIZE); messageFont = new Font("Sanserif", Font.BOLD, 16);
random = new Random();
this.addMouseListener(new MouseTrap());
mouseXPosition = 0;
mouseYPosition = 0;
} // end init
public void start ()
{
starting = true; // indicates starting or re-starting
}
public void paint (Graphics g)
{
g.setFont(messageFont);
g.drawString("Click where you think the magic robot will appear next", 20, 40);
// just display opening message first time
if (starting)
{
starting = false;
}
else // not the first time, so display robot and mouse click
{
int robotWidth = magicRobotImage.getWidth(this);
int robotHeight = magicRobotImage.getHeight(this);
// choose a random position for the robot
int robotXPosition = random.nextInt(WINDOW_X_SIZE - robotWidth);
int robotYPosition = random.nextInt(WINDOW_Y_SIZE - robotHeight);
// display the robot
g.drawImage(magicRobotImage, robotXPosition, robotYPosition, robotWidth, robotHeight, null);
// show where the mouse was when last clicked g.drawString("X", mouseXPosition, mouseYPosition);
// check whether the user clicked in the right place
Rectangle imageRectangle = new Rectangle(robotXPosition, robotYPosition, robotWidth, robotHeight);
if (imageRectangle.contains(mouseXPosition, mouseYPosition))
{
g.drawString("Well done, you found the magic robot!", 20, 80);
starting = true; // restart the game
}
else
{
g.drawString("Bad luck, you missed. Try again", 20, 60);
}
}
} // end paint
// inner class - keeps track of position of mouse clicks class MouseTrap extends MouseAdapter
{
// see later for coding details
} // end MouseTrap
}
This applet makes use of two of the four life cycle methods, namely init and start. It also uses several of the methods listed in Table - it overrides the paint method to draw the applet window and also invokes the getImage and resize methods.
The init method loads the robot image to be used, by invoking the getImage method. A special feature of the getImage method is that it returns immediately, and the next statement in the init method is executed without waiting for the image to complete loading. This is because, in general, the applet would expect to load an image over the web from the server where it originated and this might take a considerable time. The image must be completely loaded before any attempt to display it and the drawImage method used later will wait, if necessary, until the image has been loaded.
The applet method getDocumentBase returns the URL where the HTML file associated with this applet can be found. This allows it to find the robot image file for the applet.
The init method deals with other initialization such as setting the applet window size, the font to be used for displayed messages, and the default values of instance variables.
It also sets up the random number generating object and defines a listener to monitor any mouse clicks. This listener is an object of the MouseTrap class, which is defined as an inner class of the applet, and is explained in more detail later.
The paint method contains most of the code that makes the applet work. This is invoked each time the applet window needs to be redrawn, in particular, after each mouse click made by the user. Using the Graphics object referred to by the argument g, it invokes the drawString and drawImage methods of the Graphics class to display the current position of the magic robot and the user's guessed position indicated by their mouse click (shown as an 'X'). If the user clicked inside the rectangle that includes the robot image, then a suitable message is displayed. We use the getWidth and getHeight methods of the Image class to find the size of the robot image. We do not do this in the init method because, as noted earlier, the image may not have finished loading, and the loading of the image may only be completed after the first attempt to display it.
When the paint method for this applet is first invoked, it only displays the message inviting the user to guess. On subsequent invocations, it displays both the user's guess and the position of the robot until the user finds the robot. After a successful guess, it starts the game again. This behaviour is controlled by the boolean instance variable starting. The start method sets the value of this variable to ensure that the applet behaves as if starting, both the first time it is viewed and on any subsequent return to viewing the applet.
Finally, the detailed code for the inner class MouseTrap is as follows:
// inner class - keeps track of position of mouse clicks class MouseTrap extends MouseAdapter
{
// record position of mouse click and redraw the applet public void mouseClicked (MouseEvent e)
{
mouseXPosition = e.getX( );
mouseYPosition = e.getY( );
repaint( ); // invoke the applet paint method
}
} // end MouseTrap
The MouseTrap class extends the adapter class MouseAdapter. The method mouseClicked is invoked each time the user presses the mouse button. This records the x and y positions of the mouse pointer in the instance variables mouseXPosition and mouseYPosition. The method then invokes the repaint method for the applet, which causes the paint method to be invoked. This ensures that the positions of the mouse click and of the robot are shown on screen. Figure 6 shows the result of a successful guess.
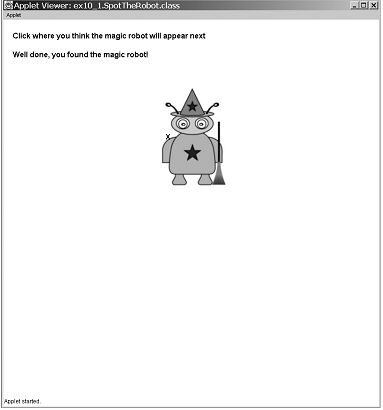
Figure: The SpotTheRobot applet window after a successful guess
In Figure, the X that indicates the position of the user's guess is not exactly on the robot picture, yet it is regarded as a successful guess. This is because we have used a simple algorithm that just checks whether the mouse click is within the bounding rectangle of the image.
The paint method should not normally be invoked directly by your program code - you should use the repaint method, which will, in turn, invoke the paint method, but ensures that other details like the background and foreground colours are correctly set.
An alternative approach to using an inner class like MouseTrap would be to make the applet class itself the listener. To do this, we would declare SpotTheRobot as implementing the MouseListener interface and would then have to provide implementations for all the methods of that interface.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your An applet example homework and assignments? Live An applet example experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer An applet example homework help, java assignment help and An applet example projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours