Adding a text area and a drawing area:
We will now continue to add some other useful elements to the top panel. We will add a text area, and an area for drawing that we will form from a JPanel component. As you will remember, a JTextArea is a general-purpose text control element that can be used to hold arbitrary text strings. We will also add scroll bars to the JTextArea.
The code to add these two elements to the display is as follows:
import java.awt. *;
import javax.swing. *;
public class SwingClass3 extends SwingClass2
{
private JTextArea ta = new JTextArea("TextArea", 10, 50);
public SwingClass3 ( )
{
super( );
// set the layout model for the topPanel
topPanel.setLayout(new GridLayout(1, 2));
// create JScrollPane around JTextArea
JScrollPane scr = new JScrollPane(ta);
// add scrollable text area to topPanel
topPanel.add(scr);
// add the MyCanvas item
topPanel.add(new MyCanvas( ));
}
}
The code for the MyCanvas class is as follows:
public class MyCanvas extends JPanel
{
public void paintComponent (Graphics g)
{
g.drawString("Canvas", 20, 20);
}
}
and in order to display the GUI we need the following class:
public class SwingClass3Test
{
public static void main (String args [])
{
SwingClass thisClass = new SwingClass3( );
thisClass.setVisible(true);
}
}
The display from this class is shown in Figure.
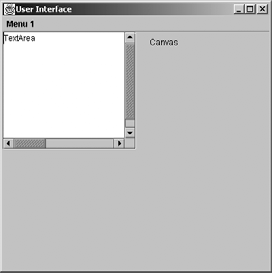
As before, we have extended the class from the previous example. In the constructor, we begin by setting the layout for the panel we will use. In this case, we initially want a grid with one row and two columns. We then employ the add method to insert a JScrollPane containing a JTextArea (with initial text set to the string "TextArea") and a MyCanvas object.
All container classes have a method for causing the components and images placed in them to appear on the screen. For a frame, this method is known as paint; for a panel, as in the example above, the method is called paintComponent. Both take an argument - a Graphics object - that enables drawing to take place. The Graphics object is supplied by the system and contains all of the methods needed for drawing on the screen. You will see more of this class and its methods. All the above code does is create a new class that inherits from JPanel and overrides the paintComponent method of JPanel with a simple instruction to display the string "Canvas" starting at (20, 20) on the panel. In the next unit, we shall describe how to draw, using objects defined by the Graphics class. For the moment, just assume that the MyCanvas object is an object with a simple string "Canvas" displayed on it. We will meet a related method, which containers also have: repaint. You have already made indirect use of repaint when you called setVisible to get a window to display. The method setVisible calls repaint, which calls paint and the window is then displayed on screen.
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your Adding a text area and a drawing area homework and assignments? Live Adding a text area and a drawing area experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer Adding a text area and a drawing area homework help, java assignment help and Adding a text area and a drawing area projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours