Adding a menu:
The first thing that we will add is a menu bar. This GUI element has not been described before. It is just a bar that contains menus; these, in turn, contain individual menu items. The JMenuBar holds JMenus, which in turn hold JMenuItems. It is the menu items that the user finally selects that cause some action to be carried out. The procedure for creating menus is somewhat long-winded. First, you must create a JMenuBar:
JMenuBar mb = new JMenuBar( );
Then you must create a new item for each menu that is required to appear in the menu bar, for example:
JMenu m = new JMenu("Menu 1");
After this you must create a JMenuItem for each selectable item that is required to appear in the menu, then tell the menu to hold the item:
m.add(new JMenuItem("MenuItem 1"));
m.add(new JMenuItem("MenuItem 2"));
Once you have completed adding items to the menu, you must add the menu to the menu bar:
mb.add(m);
Finally, when all of this has been done, you must tell the frame that you want it to use the JMenuBar that you have prepared:
setJMenuBar(mb);
This approach is fairly straightforward and the code below shows how the menu mechanism can be incorporated within a typical frame.
The code now becomes:
import java.awt. *;
import javax.swing. *;
public class SwingClass2 extends SwingClass
{
public SwingClass2 ( )
{
super( );
// add the menu bar and items
JMenuBar mb = new JMenuBar( );
JMenu m = new JMenu("Menu 1");
m.add(new JMenuItem("MenuItem 1"));
m.add(new JMenuItem("MenuItem 2")); mb.add(m);
setJMenuBar(mb);
}
}
To run the code, we use the following class:
public class SwingClass2Test
{
public static void main (String [] args)
{
SwingClass thisClass = new SwingClass2( );
thisClass.setVisible(true);
}
}
The following are a number of things to notice about this code.
1 The class SwingClass2 extends the class SwingClass defined previously, so all the public and protected methods and instance variables in this class become available to SwingClass2.
2 The constructor in SwingClass2 calls super, which in turn calls the constructor in SwingClass. This sets the frame and the panels previously defined.
3 The reference variable thisClass is declared as being of type SwingClass but has an object of type SwingClass2 assigned to it. This is an example of polymorphism, because thisClass is expecting an instance of SwingClass but is actually assigned an instance of SwingClass2 without any problems arising.
Be sure that you understand how this interface is created. Here, we have considered only the very simplest form of menu. It is possible, for example, to have items such as JCheckBoxMenuItem or JRadioButtonMenuItem and to set mnemonic selectors.
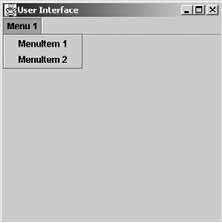
Figure: A window with a menu bar and menu items
Java Assignment Help - Java Homework Help
Struggling with java programming language? Are you not finding solution for your Adding a menu homework and assignments? Live Adding a menu experts are working for students by solving their doubts & questions during their course studies and training program. We at Expertsmind.com offer Adding a menu homework help, java assignment help and Adding a menu projects help anytime from anywhere for 24x7 hours. Computer science programming assignments help making life easy for students.
Why Expertsmind for assignment help
- Higher degree holder and experienced experts network
- Punctuality and responsibility of work
- Quality solution with 100% plagiarism free answers
- Time on Delivery
- Privacy of information and details
- Excellence in solving java programming language queries in excels and word format.
- Best tutoring assistance 24x7 hours